Overview
Each persistent data object has an EXPRESS data dictionary definition as well as C++ class information. The EXPRESS definitions are stored as instances of the RoseDomain class, while the C++ class information is handled by the RoseType class. This arrangement is illustrated below for data describing a point.
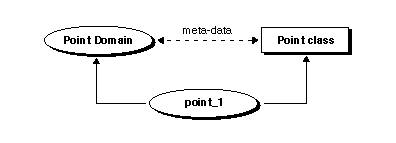
The EXPRESS data dictionary information and C++ classes are generated by the EXPRESS compiler.
Note
The RoseDomain functions return actual EXPRESS data dictionary information used by the ROSE library. This information should be considered read-only and should not be deleted or modified in any way.
Each RoseDomain contains a persistent list of the attributes defined by the domain and a list of the domains one level above it in the inheritence hierarchy. In addition, when the domain is read into memory, it will construct several non-persistent lists of resolved type information.
The domain constructs a list containing inherited as well as locally defined attributes. This list is constructed by making non-presistent copies of each local or inherited attribute. Each non-persistent copy keeps a pointer back to the original persistent object. The copies hold extra C++ specific information such as offsets and byte alignment, that is used internally by the ROSE library.
The domain also constructs a list of all supertypes above the domain in the type hierarchy and all subtypes below the domain in the type hierarchy. The list of subtypes is open-ended. A domain may have subtypes in schemas that have not been read into memory, but this list only contains subtypes that are in memory.
findTypeAttribute()
RoseAttribute * findTypeAttribute( const char * att_name ); RoseAttribute * findTypeAttribute( RoseAttribute * att );
The findTypeAttribute() functions search through all locally defined and inherited attributes of a domain. The first version searches for an attribute with a specific name while the second searches for the non-persistent copy of an attribute. The second version is equivalent to RoseAttribute::nonPersistentViewIn(). If the attribute is not found, the functions return null.
findTypeAttributeForType()
RoseAttribute * findTypeAttributeForType( const char * domain_name ); RoseAttribute * findTypeAttributeForType( RoseDomain * domain );
The findTypeAttributeForType() functions search through all locally defined and inherited attributes of a domain. The functions search for the first attribute that could hold the specified type of data. These functions are useful when working with select types.
findTypeImmediateAttribute()
RoseAttribute * findTypeImmediateAttribute( const char * att_name ); RoseAttribute * findTypeImmediateAttribute( RoseAttribute * att );
The findTypeImmediateAttribute() functions search through the locally defined attributes of a domain. The first version searches for an attribute with a specific name while the second searches for the persistent copy of an attribute. The second version is equivalent to the RoseAttribute::persistentView() function. If the attribute is not found, the functions return null.
findTypeSubType()
RoseDomain * findTypeSubType( const char * domain_name );
The findTypeSubType() function searches through the subtypes of a domain for one with a particular name. If no subtype is found, the function will return null. Note that the list of subtypes is open-ended. A domain may have subtypes in schemas that have not been read into memory, but this function can only search for those subtypes that are in memory.
findTypeSuperType()
RoseDomain * findTypeSuperType( const char * domain_name );
The findTypeSuperType() function searches through the supertypes of a domain for one with a particular name. If no supertype is found, the function will return null. A domain may have supertypes in other schemas, but all such schemas are read into memory when the domain is first resolved.
isSuperTypeOf()
RoseBoolean isSuperTypeOf( const char * domain_name ); RoseBoolean isSuperTypeOf( RoseDomain * domain );
The isSuperTypeOf() functions are the inverse of the typeIsa() function. Instead of determining whether a domain is derived from the argument, this function determines whether the argument has been derived from the domain.
name()
char * name();
The name() function returns the name of the domain.
pnewInstance()
RoseObject * pnewInstance(); RoseObject * pnewInstance( unsigned sz, ); RoseObject * pnewInstance( RoseDesign * owner, unsigned sz = 0 ); RoseObject * pnewInstance( RoseDesignSection * owner, unsigned sz = 0 );
The pnewInstance() functions create an instance the object type described by the domain. The design and section arguments specify the owner of the new object. The functions that do not accept an owner argument put the new object in the RoseInterface current design
The size parameter is used when creating instances of aggregates. The size specifies the initial size in the case of arrays, or that capacity in the case of lists, sets, and bags.
For applications that need more control over object identifiers, the third version can be used to create a instance with a specific ROSE object identifier (OID). The OID should be one that has been created by ROSE. Consult RoseDesign::pnewInstance() for more information and examples.
short_name()
char * short_name(); void short_name( char * new_name );
The short_name() function returns the STEP short name of an entity, if one has been supplied. Refer to Short Names for more information about specifying short names. The second function can be used to temporarily change the short name of a domain. The information will not be preserved past the application run. The function does not copy the string, so an application should make sure that the supplied string will not be inadvertantly deleted.
typeAttributes()
ListOfRoseAttribute * typeAttributes();
The typeAttributes() function return a list of all locally declared and inherited attributes of a domain. These objects are non-persistent copies of the attributes as described in the introduction.
typeImmediateAttributes()
ListOfRoseAttribute * typeImmediateAttributes();
The typeImmediateAttributes() function return a list of only locally declared attributes of a domain. These objects are persistent as described in the introduction.
typeImmediateSubTypes()
ListOfRoseDomain * typeImmediateSubTypes();
The typeImmediateSubTypes() function returns a list containing domains directly derived from this domain. Immediate subtypes are one level below this domain in the type hierarchy. Note that the list of subtypes is open-ended. A domain may have subtypes in schemas that have not been read into memory, but this list only contains those subtypes that are in memory.
typeImmediateSuperTypes()
ListOfRoseDomain * typeImmediateSuperTypes();
The typeImmediateSuperTypes() function returns a list containing the domains that this domain has been directly derived from. Immediate supertypes are one level above this domain in the type hierarchy.
typeIsa()
RoseBoolean typeIsa( const char * domain_name ); RoseBoolean typeIsa( RoseDomain * domain );
The typeIsa() function examines the type hierarchy and determines whether this domain has been derived from the specified domain. The function returns true if this domain derived from the specified domain.
typeIsAbstract()
RoseBoolean typeIsAbstract();
The typeIsAbstract() function indicates whether an EXPRESS entity definition has been tagged as an abstract supertype using the ABSTRACT keyword.
typeIsBestFit()
RoseBoolean typeIsBestFit();
The typeIsBestFit() function indicates whether an application has an exact C++ type for an EXPRESS definition. If the function returns true, the application does not have a specific C++ class for the domain and will use the closest approximation or best fit
class. If the function returns false, the application has an exact C++ match for the EXPRESS definition.
typeIsComplex()
RoseBoolean typeIsComplex();
The typeIsComplex() function indicates whether a domain represents an EXPRESS AND/OR combination of entity types. Complex domains have no attributes and have as supertypes the domains that are ANDed together. The name of a complex domain is constructed by concatenating the names of the supertypes with _and_
.
typeIs<type>()
RoseBoolean typeIsSimple(); RoseBoolean typeIsAggregate(); RoseBoolean typeIsEntity(); RoseBoolean typeIsSelect();
The typeIsSimple() function returns yes if the domain represents one of the EXPRESS primitive types, like real, integer, or string. The typeIsAggregate() function determines whether the domain represents an aggregate. The typeIsEntity() function checks if it is an EXPRESS entity type, and the typeIsSelect() function checks if it is an EXPRESS select type.
typeNodeType()
RoseNodeType typeNodeType();
The typeNodeType() function returns an enumeration indicating the basic category of EXPRESS data that is described by the domain. This enumeration is used internally in many places. Consult RoseNodeType for a description of the enumeration.
typeRoseType()
RoseTypePtr& typeRoseType();
The typeRoseType() function returns the RoseType definition for the C++ data type that has been matched with this EXPRESS definition.
typeSubTypes()
ListOfRoseDomain * typeSubTypes();
The typeSubTypes() function returns a list containing all domains derived from this domain. Note that the list of subtypes is open-ended. A domain may have subtypes in schemas that have not been read into memory, but this list only contains those subtypes that are in memory.
typeSuperTypes()
ListOfRoseDomain * typeSuperTypes();
The typeSuperTypes() function returns a list containing all domains that this domain has been derived from. This includes those supertypes that may have been declared in the EXPRESS schema, all inherited supertypes, as well as RoseObject and the base classes such as RoseStructure, RoseUnion, or RoseAggregate.