Overview
Products are at the very center of all STEP data. They are organized into assembly trees and CAD shape data is attached to them as a special type of propery.
The products and product_definition objects also have a context that indicates the general type of information described by the file. These also refer to an application context, which describes the application protocol and general area of use. Typically, a file only has one AP context.
STEP assemblies are structured as two parallel trees. A tree of products describes the logical hierarchy of an assembly, while a tree of shapes describe the geometry of each component and its placement in space. The two trees are usually roughly parallel, with links between a product and its shape, and between corresponding product and shape relationship objects.
Individual products, sub-assemblies, and associated shape structure might appear many times, and in differnt ways. Resolving all of these connections is challenging, so we do that and provide you with simple ways to use STEP assemblies.
Call stix_asm_tag() before using these functions. This scans the STEP assembly data and adds extra information for efficient access. You only need to call the function once unless you change the assembly structure.
This creates an index for the product tree and one for the shape tree that gives a unique number to each appearance of a product or shape across repeated sub-assemblies. With this "use number" we can quickly get information about any point in an assembly, such as:
- STEP product definition and relationship
- STEP representation and relationship
- Parent product use or shape use
- Product use for any shape use, and shape uses for any product use.
- Count of product uses and shape uses in a subtree, each of which will be in a continuous block.
- Fully resolved transform to place any shape use within the global space. The incremental transforms at each layer in the shape tree are available from the STEP relationship objects.
Example
The program fragment below prints an indented list showing the product and shape structure for the assemblies in a file.
RoseDesign * d; // index the STEP assembly information. stix_asm_tag(d); unsigned i,sz; StixAsmProductIndex * pidx = stix_asm_product_index(d); StixAsmShapeIndex * sidx = stix_asm_shape_index(d); printf(PRODUCT TREE -----\n); for (i=0, sz=pidx->size(); i<sz; i++) { stp_product_definition * pd = pidx->getAsmPdef(i); const char * name = pd-> formation()-> of_product()-> name(); indent(pidx->getAsmDepth(i)); printf([%u] PD #%lu, size: %u - %s\n, i, pd->entity_id(), pidx->getAsmSize(i), name); // Much other information available, link to shape uses in the // shape tree, parent in product tree, STEP relation object. } printf(SHAPE TREE -----\n); for (i=0, sz=sidx->size(); i<sz; i++) { stp_representation * rep = sidx->getAsmRep(i); const double * v = sidx->getAsmGlobalXform(i); // just print the origin because it is the most interesting part indent(sidx->getAsmDepth(i)); printf([%u] REP #%lu, product-use: [%u], origin: %.4f %.4f %.4f\n, i, rep->entity_id(), sidx->getAsmProductUse(i), v[12], v[13], v[14]); // Much other information available, link to product use in the // product tree, parent in shape tree, STEP relation object. }
Running this program on this bracket assembly produces the following output. Note that the assembly uses the bolt and bracket sub-assemblies several times, so the origins will be different in the shape tree.
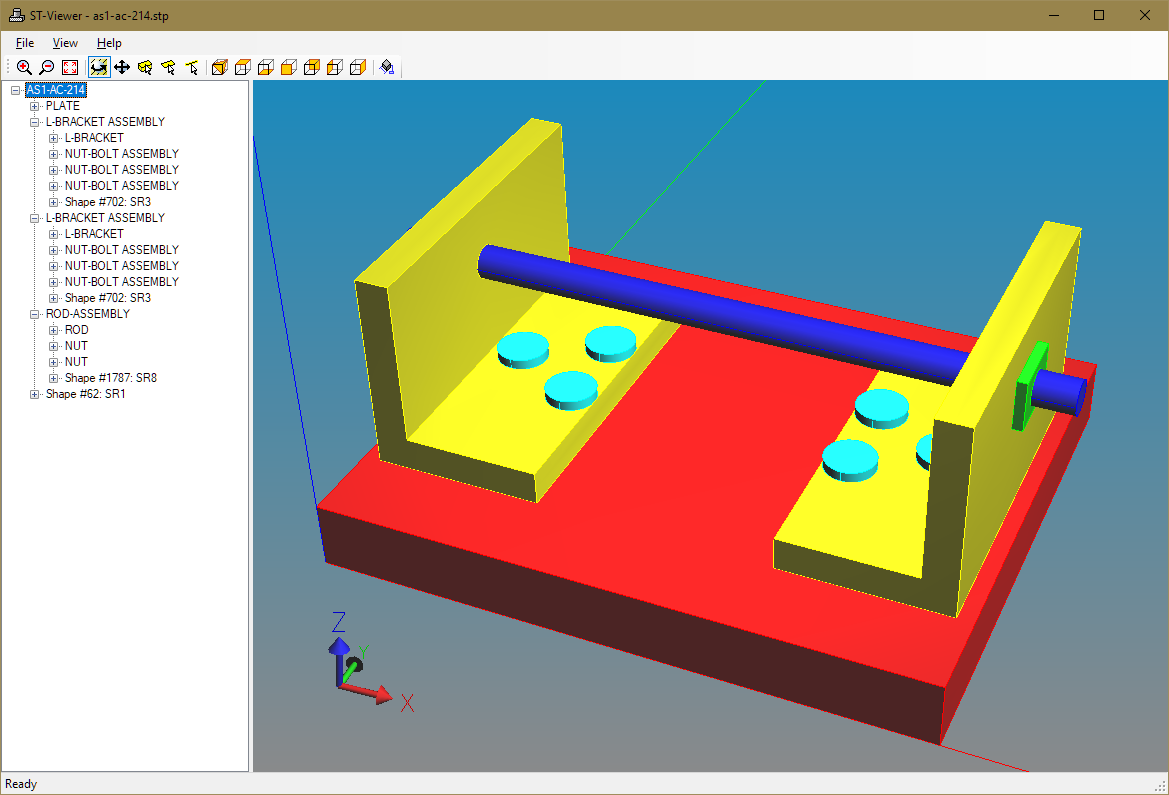
PRODUCT TREE ----- [0] PD #52, size: 28 - AS1-AC-214 [1] PD #72, size: 1 - PLATE [2] PD #701, size: 11 - L-BRACKET ASSEMBLY [3] PD #712, size: 1 - L-BRACKET [4] PD #1257, size: 3 - NUT-BOLT ASSEMBLY [5] PD #1268, size: 1 - BOLT [6] PD #1469, size: 1 - NUT [7] PD #1257, size: 3 - NUT-BOLT ASSEMBLY [8] PD #1268, size: 1 - BOLT [9] PD #1469, size: 1 - NUT [10] PD #1257, size: 3 - NUT-BOLT ASSEMBLY [11] PD #1268, size: 1 - BOLT [12] PD #1469, size: 1 - NUT [13] PD #701, size: 11 - L-BRACKET ASSEMBLY [14] PD #712, size: 1 - L-BRACKET [15] PD #1257, size: 3 - NUT-BOLT ASSEMBLY [16] PD #1268, size: 1 - BOLT [17] PD #1469, size: 1 - NUT [18] PD #1257, size: 3 - NUT-BOLT ASSEMBLY [19] PD #1268, size: 1 - BOLT [20] PD #1469, size: 1 - NUT [21] PD #1257, size: 3 - NUT-BOLT ASSEMBLY [22] PD #1268, size: 1 - BOLT [23] PD #1469, size: 1 - NUT [24] PD #1786, size: 4 - ROD-ASSEMBLY [25] PD #1797, size: 1 - ROD [26] PD #1469, size: 1 - NUT [27] PD #1469, size: 1 - NUT SHAPE TREE ----- [0] REP #62, product-use: [0], origin: 0.0000 0.0000 0.0000 [1] REP #73, product-use: [1], origin: 90.0000 75.0000 10.0000 [2] REP #680, product-use: [1], origin: 90.0000 75.0000 10.0000 [3] REP #702, product-use: [2], origin: 64.6367 125.0000 282.5273 [4] REP #713, product-use: [3], origin: 150.0000 75.0000 50.0000 [5] REP #1236, product-use: [3], origin: 150.0000 75.0000 50.0000 [6] REP #1258, product-use: [4], origin: -97.5273 56.0634 98.8529 [7] REP #1269, product-use: [5], origin: 155.0000 75.0000 31.5000 [8] REP #1448, product-use: [5], origin: 155.0000 75.0000 31.5000 [9] REP #1470, product-use: [6], origin: 155.0000 75.0000 -1.5000 [10] REP #1705, product-use: [6], origin: 155.0000 75.0000 -1.5000 [11] REP #1258, product-use: [7], origin: -120.0273 43.0730 98.8529 [12] REP #1269, product-use: [8], origin: 132.5000 62.0096 31.5000 [13] REP #1448, product-use: [8], origin: 132.5000 62.0096 31.5000 [14] REP #1470, product-use: [9], origin: 132.5000 62.0096 -1.5000 [15] REP #1705, product-use: [9], origin: 132.5000 62.0096 -1.5000 [16] REP #1258, product-use: [10], origin: -120.0273 69.0538 98.8529 [17] REP #1269, product-use: [11], origin: 132.5000 87.9904 31.5000 [18] REP #1448, product-use: [11], origin: 132.5000 87.9904 31.5000 [19] REP #1470, product-use: [12], origin: 132.5000 87.9904 -1.5000 [20] REP #1705, product-use: [12], origin: 132.5000 87.9904 -1.5000 [21] REP #702, product-use: [13], origin: 115.3633 25.0000 282.5273 [22] REP #713, product-use: [14], origin: 30.0000 75.0000 50.0000 [23] REP #1236, product-use: [14], origin: 30.0000 75.0000 50.0000 [24] REP #1258, product-use: [15], origin: 277.5273 93.9366 98.8529 [25] REP #1269, product-use: [16], origin: 25.0000 75.0000 31.5000 [26] REP #1448, product-use: [16], origin: 25.0000 75.0000 31.5000 [27] REP #1470, product-use: [17], origin: 25.0000 75.0000 -1.5000 [28] REP #1705, product-use: [17], origin: 25.0000 75.0000 -1.5000 [29] REP #1258, product-use: [18], origin: 300.0273 106.9270 98.8529 [30] REP #1269, product-use: [19], origin: 47.5000 87.9904 31.5000 [31] REP #1448, product-use: [19], origin: 47.5000 87.9904 31.5000 [32] REP #1470, product-use: [20], origin: 47.5000 87.9904 -1.5000 [33] REP #1705, product-use: [20], origin: 47.5000 87.9904 -1.5000 [34] REP #1258, product-use: [21], origin: 300.0273 80.9462 98.8529 [35] REP #1269, product-use: [22], origin: 47.5000 62.0096 31.5000 [36] REP #1448, product-use: [22], origin: 47.5000 62.0096 31.5000 [37] REP #1470, product-use: [23], origin: 47.5000 62.0096 -1.5000 [38] REP #1705, product-use: [23], origin: 47.5000 62.0096 -1.5000 [39] REP #1787, product-use: [24], origin: 190.0000 -101.7520 -70.5288 [40] REP #1798, product-use: [25], origin: 90.0000 75.0000 60.0000 [41] REP #1897, product-use: [25], origin: 90.0000 75.0000 60.0000 [42] REP #1470, product-use: [26], origin: 3.5000 75.0000 60.0000 [43] REP #1705, product-use: [26], origin: 3.5000 75.0000 60.0000 [44] REP #1470, product-use: [27], origin: 176.5000 75.0000 60.0000 [45] REP #1705, product-use: [27], origin: 176.5000 75.0000 60.0000
stix_ap_find_context()
stp_application_context * stix_ap_find_context ( RoseDesign * d, StplibSchemaType s = stplib_schema_none );
The stix_ap_find_context() function searches for an application protocol definition that matches the given schema type and returns the AP context associated with it. Definitions with a null context are ignored. If "none" or "other" are given, the function will use the first application protocol definition that it finds. See stix_ap_make_context() if you want to create a context if one does not exist.
stix_ap_find_definition()
stp_application_protocol_definition * stix_ap_find_definition( RoseDesign * d, StplibSchemaType s = stplib_schema_none );
The stix_ap_find_definition() function searches for an application protocol definition that matches the given schema type. Definitions with a null context are ignored. If "none" or "other" are given, the function will use the first application protocol definition that it finds.
stix_ap_make_context()
stp_application_context * stix_apcontext_make( RoseDesign * d, StplibSchemaType s = stplib_schema_none );
The stix_ap_make_context() function returns the AP context given by stix_ap_find_context() if one exists. If no match is found, the function will create an AP context and associated application protocol definition object.
When creating a new context, the function will use the value returned by stplib_get_schema() if the schema type of "none" or "other" is given.
stix_ap_make_definition()
stp_application_protocol_definition * stix_ap_make_definition( RoseDesign * d, StplibSchemaType s = stplib_schema_none );
The stix_ap_make_definition() function creates an AP definition with the appropriate schema strings. The returned object is incomplete as it does not refer to an AP context. Use stix_ap_make_context() instead to create a complete definition.
For "none" or "other" the function will use the value returned by stplib_get_schema().
stix_ap_merge_context()
unsigned stix_ap_merge_context (RoseDesign * d);
The stix_ap_merge_context() function merges repeated application protocol definition and context information in a file. After calling this function, a file will have at most one stp_application_context instance and at most one stp_application_protocol_definition instance.
The function returns the number of duplicates that were merged. If non-zero, call rose_empty_trash() to update the pointers and delete the duplicates.
stix_asm_find_root_products()
void stix_asm_find_root_products( StpAsmProductDefVec * roots, RoseDesign * design );
The stix_asm_find_root_products() function fills a list with all of the root-level product definitions.
This function does not return products associated with orphan shapes, even though they are technically root level objects. If you want to see those, traverse all of the product definitions and look for ones that have no parent NAUO links.
stix_asm_find_root_shapes()
void stix_asm_find_root_shapes( StpAsmShapeRepVec * shapes, RoseDesign * design );
The stix_asm_find_root_shapes() function fills a list with all of the root shapes in the design.
stix_asm_find_shapes_wo_product()
void stix_asm_find_shapes_wo_product( StpAsmShapeRepVec * shapes, RoseDesign * design );
The stix_asm_find_shapes_wo_product() function fills a list with all of the shape_reps with no owning product_definition. These should not exist, but there are some files which include such data. This function does use a ROSE traversal.
stix_asm_get_related_pdef()
stp_product_definition * stix_asm_get_related_pdef( stp_product_definition_relationship * rel );
The stix_asm_get_related_pdef() function returns the related product definition from a product definition relationship. The function returns null if the value is not a product_definition.
The type of the related and relating attributes were changed when moving from AP203/214 to AP242. They now hold a select type that can take other types of values. Use this function and stix_asm_get_relating_pdef() to migrate older code and to get values without worrying about the select type.
stix_asm_get_relating_pdef()
stp_product_definition * stix_asm_get_relating_pdef( stp_product_definition_relationship * rel );
The stix_asm_get_relating_pdef() function returns the relating product definition from a product definition relationship. The function returns null if the value is not a product_definition.
The type of the related and relating attributes were changed when moving from AP203/214 to AP242. They now hold a select type that can take other types of values. Use this function and stix_asm_get_related_pdef() to migrate older code and to get values without worrying about the select type.
stix_asm_get_reprel_1/2()
stp_representation * stix_asm_get_reprel_1( stp_representation_relationship * rel ); stp_representation * stix_asm_get_reprel_2( stp_representation_relationship * rel );
The stix_asm_get_reprel_1() and stix_asm_get_reprel_2()
functions return the representation value of the rep_1
and rep_2
attributes of a representation relationship.
The functions return null if the value is not a representation.
The type of these attributes were changed when moving from AP242e1, AP214, and AP203 to AP242 second edition. They now hold a select type that can take other types of values. Use these functions to migrate older code and to get values without worrying about the select type.
stix_asm_product_index()
StixAsmProductIndex * stix_asm_product_index( RoseDesign * d );
The stix_asm_product_index() function returns the assembly product tree index for a given file. The index is built by calling stix_asm_tag() after reading the file into memory.
stix_asm_put_related_pdef()
void stix_asm_put_related_pdef( stp_product_definition_relationship * rel, stp_product_definition * pd );
The stix_asm_put_related_pdef() function sets the related product definition of a product definition relationship to a given product definition.
The type of the related and relating attributes were changed when moving from AP203/214 to AP242. They now hold a select type that can take other types of values. Use this function and stix_asm_put_relating_pdef() to migrate older code and to set values without worrying about the select type.
stix_asm_put_relating_pdef()
void stix_asm_put_relating_pdef( stp_product_definition_relationship * rel, stp_product_definition * pd );
The stix_asm_put_relating_pdef() function sets the relating product definition of a product definition relationship to a given product definition.
The type of the related and relating attributes were changed when moving from AP203/214 to AP242. They now hold a select type that can take other types of values. Use this function and stix_asm_put_related_pdef() to migrate older code and to set values without worrying about the select type.
stix_asm_put_reprel_1/2()
void stix_asm_put_reprel_1( stp_representation_relationship * rel, stp_representation * rep ); void stix_asm_put_reprel_2( stp_representation_relationship * rel, stp_representation * rep );
The stix_asm_put_reprel_1() and stix_asm_put_reprel_2()
functions set the rep_1
and rep_2
attributes
of a representation relationship to a given representation. The type
of these attributes were changed when moving from AP242e1, AP214, and
AP203 to AP242 second edition. They now hold a select type that can
take other types of values.
Use these functions to migrate older code and to set values without worrying about the select type.
stix_asm_relation_xform()
void stix_asm_relation_xform ( double result[16], stp_shape_representation_relationship * shape_rel, RoseUnit u = roseunit_unknown ); void stix_asm_relation_xform ( double result[16], stp_mapped_item * shape_rel, RoseUnit u = roseunit_unknown ); void stix_asm_relation_xform ( double result[16], StixMgrAsmRelation * m, RoseUnit u = roseunit_unknown );
The stix_asm_relation_xform() function returns the local transformation matrix for a given representation relationship or mapped item.
stix_asm_shape_index()
StixAsmShapeIndex * stix_asm_shape_index( RoseDesign * d );
The stix_asm_shape_index() function returns the assembly shape tree index for a given file. The index is built by calling stix_asm_tag() after reading the file into memory.
stix_asm_tag()
void stix_asm_tag( RoseDesign * d );
The stix_asm_tag function looks at all of the product definitions, shapes, and relationships in the file, recognizes assemblies, annotates them with backpointers, and determines the correct parent-child direction for relationships.
You must call this function before using any of the assembly functions or managers described on this page.
stix_pdef_find_context()
stp_product_context * stix_pdef_find_context( RoseDesign * d, StplibSchemaType s = stplib_schema_none );
The stix_pdef_find_context() function searches for a product definition context object. If a schema type is given, the function searches for a life cycle value that is appropriate for that AP. If "none" or "other" are given, the function will return the first context that it finds.
stix_pdef_make_context()
stp_product_definition_context * stix_pdef_make_context ( RoseDesign * d, StplibSchemaType s = stplib_schema_none );
The stix_pdef_make_context() function returns the context given by stix_pdef_find_context() if one exists. If no match is found, the function will create a context. A product definition must have a context which identifies life cycle stage of the data being presented. Normally only one is needed in a given file.
When creating a new context, if a schema type of "none" or "other" is given the function will use the value returned by stplib_get_schema() to determine the life cycle string.
stix_pdef_make_for_shape()
stp_product_definition * stix_pdef_make_for_shape( stp_shape_representation * rep );
The stix_pdef_make_for_shape() function creates an AP242 product definition for a given shape_representation. The fields are initialized with constants for AP242. This can simplify creating valid STEP file when writing out geometry.
stix_product_find_context()
stp_product_context * stix_product_find_context( RoseDesign * d, StplibSchemaType s = stplib_schema_none );
The stix_product_find_context() function searches for a product context object. If a schema type is given, the function searches for a discipline value that is appropriate for that AP. If "none" or "other" are given, the function will return the first context that it finds.
stix_product_make_context()
stp_product_context * stix_product_make_context( RoseDesign * d, StplibSchemaType s = stplib_schema_none );
The stix_product_make_context() function returns the context given by stix_product_find_context() if one exists. If no match is found, the function will create a context. A product must have a context which identifies engineering discipline's point of view the data is being presented by the AP properties (mechanical, electrical, etc.) Normally only one is needed in a given file.
When creating a new context, if a schema type of "none" or "other" is given the function will use the value returned by stplib_get_schema() to determine the discipline string.
stix_product_make_for_shape()
stp_product * stix_product_make_for_shape_rep( stp_shape_representation * rep );
The stix_product_make_for_shape function creates an AP242 product structure (e.g. product, product_definition_formation, product_definition and other associated instances). For a given shape_representation. The fields are initialized with constants for AP242. This can simplify creating valid STEP file when writing out geometry.
stix_product_merge_contexts()
unsigned stix_product_merge_contexts( RoseDesign * d );
The stix_product_merge_contexts() function examines all product contexts, product definition contexts, and other application context elements in a file. If the attributes are the same (aside from upper/lower case differences) they will be merged. So two product contexts will be merged with the same "discipline_type", product definition contexts with the same "life_cycle_stage", or product concept contexts with the same "market_segment_type".
The function returns the number of duplicates that were merged. If non-zero, call rose_empty_trash() to update the pointers and delete the duplicates.
stix_tess_make_shape_rep()
stp_tessellated_shape_representation * stix_tess_make_shape_rep( RoseDesign * targ_des, const RoseMesh * fs, stp_representation_context * ctx, stp_manifold_solid_brep * geom_link = 0 );
The stix_tess_make_shape_rep creates an AP242 tessellated shape representation for a given mesh. This function calls stix_tess_make_solid() to create a solid from the mesh and then creates a shape representation object to hold the solid.
The ctx context parameter specifies the units for the resulting shape representation.
If the optional geom_link parameter is given, the resulting solid point back to this brep through its geometric_link field. This parameter should be the original brep that was faceted to make the mesh, and the target design should be the same design as the original data. This will also set the geometric link for each tesselated face back to the original STEP face that was faceted to make the mesh face.
stix_tess_make_solid()
stp_tessellated_solid * stix_tess_make_solid( RoseDesign * targ_des, const RoseMesh * fs, stp_manifold_solid_brep * geom_link = 0 );
The stix_tess_make_solid() function creates an AP242 tessellated solid from a mesh. The resulting solid will have faces corresponding to any found in the mesh.
If the optional geom_link parameter is given, the resulting solid point back to this brep through its geometric_link field. This parameter should be the original brep that was faceted to make the mesh, and the target design should be the same design as the original data. This will also set the geometric link for each tesselated face back to the original STEP face that was faceted to make the mesh face.
StixMgrAsmProduct class
class StixMgrAsmProduct
The StixMgrAsmProduct class is a subtype of RoseManager that is attached to a product_definition. This holds pointers to the NAUOs that this product participates in.
/* Back pointer to next_assembly_usage_occurrence instance that * reference this product as a parent. To get the child products, * get the NAUO, and follow the related_product_defintition * attribute. */ StpAsmNauoVec child_nauos; /* Back pointer to the next_assembly_usage_occurrence that * reference this product as a child */ StpAsmNauoVec parent_nauos; /* True if a owned shape_rep (in shapes below) is a child of * another shape rep, but there is no NAUO relating the products. * If it were not for those shapes, this would be a root product. */ RoseBoolean has_orphan_shapes; /* * The shape reps that belong to this product */ StpAsmShapeRepVec shapes; /* True if this product has a parent. This can by true even of no * NAUO references it, since we do have some data where there is a * mapped_item between two shape_reps, but no NAUO relating the * products. */ RoseBoolean has_parent;
StixMgrAsmShapeRep class
class StixMgrAsmShapeRep
The StixMgrAsmProduct class is a subtype of RoseManager that is attached to a shape_representation.
StpAsmProductDefVec owning_roots; /* The parent and child rep_relationships */ StpAsmShapeRepRelVec child_rels; StpAsmShapeRepRelVec parent_rels; /* The parent and child mapped items. Child is not really * necessary -- you could search the items set for mapped_item * instances, but this will simplify the process. */ StpAsmMappedItemVec child_mapped_items; StpAsmMappedItemVec parent_mapped_items; /* Back pointers to product_defs and linking shape_def_reps that * point to the shape_rep. These vectors parallel each other and * we could probably remove one of them. */ StpAsmProductDefVec products; StpAsmShapeDefRepVec shape_def_reps; stp_axis2_placement_3d * root_placement;
StixMgrAsmRelation class
class StixMgrAsmRelation
The StixMgrAsmRelation class is a subtype of RoseManager that records the relation between two representation_items. Normally these are the axis placements in the parent and child that are used to place the component. Depending on how the assembly was constructed, this manager is attached to either a shape_reprepresentation_relationship or a mapped_item.
stp_next_assembly_usage_occurrence * owner; StpAsmProductDefVec owning_roots; /* The source and target placements. */ stp_representation_item * origin; stp_representation_item * target; /* For a shape_rep_relationship, we assume that the component is * rep_1 and and assembly as a whole is rep_2. If this is not * true, then the reversed variable is true. In either case, the * origin and target rep_items above are always consistent. */ RoseBoolean reversed; stp_representation * child;