Overview
The geometry in CAD data is usually a boundary representation based on a variety of mathematically exact surfaces. Graphics hardware and APIs like GL or DirectX need a simpler description based on triangles. The STEP mesher and IFC mesher compute this mesh description from STEP or IFC geometry.
The mesh classes and functions are defined in
the rose_mesh.h
,
rose_delaunay.h
, rose_mesh_topo.h
,
and rose_mesh_worker.h
header files.
- RoseMesh holds the data that makes up a mesh: lists of numeric data for coordinates and normals, lists of facets, and the groupings into faces.
- RoseMeshFacet is a simple structure with the coordinates and normal vectors for the three corners of a triangle.
- RoseMeshFace calls out a range of facets with associated properties.
- RoseMeshTopology is a class that analyzes and validates the topology of a facet set. This class assembles information about edges that exist between the facets so an application can determine which facets are adjacent to each other.
The rose_mesh_worker
functions provide a basic
interface for managing multi-threaded meshing of geometry. You can
set up a pool of threads and submit jobs to facet shells and
individual faces, then wait for the results.
See rose_mesh_worker_render()
for discussion and examples.
The Mesh XML File Format is a
simple XML structure read and written by
the rose_mesh_xml
functions.
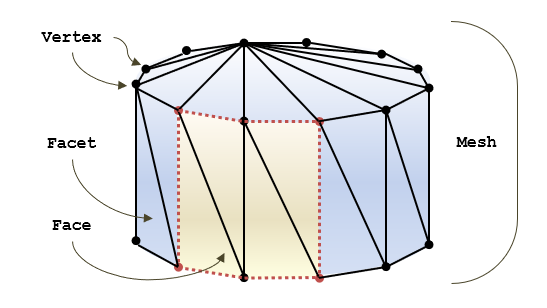
Mesh Macros
// missing value for surface normal or vertex #define ROSE_MESH_NULL_IDX ((unsigned)-1) #define ROSE_MESH_NULL_COLOR 0xff000000 #define ROSE_MESH_UNCERTAINTY 1e-8
These macros are used in the mesh structures to indicate special
values. The ROSE_MESH_NULL_IDX
value is used in
a RoseMeshFacetto indicate
that a vertex or surface normal is not provided.
The ROSE_MESH_NULL_COLOR
value is used by the various
color functions,
like getFaceColor(), to
indicate that no color value is present.
The ROSE_MESH_UNCERTAINTY
value is the default global
uncertainty used by
the RoseMeshOptions. When meshing
STEP or IFC solids, the uncertainty is usually given by the
representation context.
rose_mesh_cache_add()
void rose_mesh_cache_add( RoseObject * obj, RoseMesh * mesh );
The rose_mesh_cache_add() function associates a mesh with a data object. Once added, the mesh is owned by the object and will be deleted when the object is deleted. Many meshes can be associated with an object.
rose_mesh_cache_delete()
void rose_mesh_cache_delete ( RoseObject * obj, RoseMesh * mesh = 0 );
The rose_mesh_cache_delete() function deletes one or more meshes associated with a data object. If a mesh is given, only that mesh will be deleted. If null is passed in, all meshes will be deleted.
rose_mesh_cache_detach()
void rose_mesh_cache_detach( RoseObject * obj, RoseMesh * mesh );
The rose_mesh_cache_detach() function removes the association between a mesh and a data object. The caller must take responsibility for deleting the mesh at the appropriate time.
rose_mesh_cache_find()
RoseMesh * rose_mesh_cache_find( RoseObject * obj, RoseMeshOrigin org = ROSE_MESH_ORIGIN_EFFECTIVE, RoseMeshNotify * notify = 0 );
The rose_mesh_cache_find() function searches the meshes associated with the object for the first one that matches the given qualifications. By default, it searches for the first mesh with a computed origin, body origin, or anything else, in that order. See RoseMesh::getOrigin() for more discussion.
rose_mesh_cache_find_with_rep()
RoseMesh * rose_mesh_cache_find_with_rep( RoseObject * obj, RoseObject * rep_obj, RoseMeshOrigin org = ROSE_MESH_ORIGIN_EFFECTIVE, RoseMeshNotify * notify = 0 );
The rose_mesh_cache_find_with_rep() function searches the meshes associated with the object for the first one that matches the given qualifications. The getRepObject() value of the mesh must match, followed by the origin as described in rose_mesh_cache_find().
rose_mesh_cache_get()
RoseMesh * rose_mesh_cache_get( RoseObject * obj, unsigned i );
The rose_mesh_cache_get() function returns a mesh associated with a data object. The total number is given by rose_mesh_cache_size() and meshes are retrieved by index.
rose_mesh_cache_size()
unsigned rose_mesh_cache_size( RoseObject * obj );
The rose_mesh_cache_size() function returns the total number of meshes associated with a data object.
rose_mesh_copy()
void rose_mesh_copy ( RoseMesh * result, const RoseMesh * src, const double xform = 0 // double [16] ); void rose_mesh_copy ( RoseMesh * result, const RoseMesh * src, const RoseXform &xform );
The rose_mesh_copy() function duplicates a set of facets. The result facet set is is cleared and then all facet data from src is copied into it. If a transform is specified, it will be applied to the points and normals as they are copied.
The RoseMesh class also defines copy and assignment operators, which may be easier to use if you are not copying with a transform. The copy and assignment operators simply call rose_mesh_copy().
RoseMesh base; RoseMesh fs; // copy mesh with simple assignment fs = base; // copy mesh with copy constructor RoseMesh fs2(fs); // copy using function rose_mesh_copy (&fs, &base); // a rotation 90deg around Y, so X=>-Z and Z=>X RoseXform rot90; rot90.put_rotation(RoseDirection(0,1,0), 90, roseunit_deg); rose_mesh_copy (&fs, &base, rot90);
rose_mesh_delete_all()
void rose_mesh_delete_all( RoseDesign * d, RoseMeshNotify * notify = 0 );
The rose_mesh_delete_all() function deletes all meshes associated with a design by calling rose_mesh_cache_delete() on every data object in a design.
rose_mesh_fpu_restore()
void rose_mesh_fpu_restore( RoseMeshFPUState * fpu );
The rose_mesh_fpu_restore function restores the state of the floating point unit to the state saved by a previous call to rose_mesh_fpu_save().
rose_mesh_fpu_save()
void rose_mesh_fpu_save( RoseMeshFPUState * fpu=0 ); typedef unsigned RoseMeshFPUState;
The rose_mesh_fpu_save() function saves the state of the floating point unit in an optional parameter and then puts it into high precision mode for use by faceting applications.
rose_mesh_job_cancel()
void rose_mesh_job_cancel( RoseMesh * mesh, int wait = 1 ); void rose_mesh_job_cancel( RoseMeshJob * job, int wait = 1 );
The rose_mesh_job_cancel() function cancels a single background job, or all jobs for a mesh so that they will not run. If a job is already running, this function will set a flag in the job that may cause it to end early. A non-zero value for the "wait" flag will force the function to block until the jobs are no longer running.
rose_mesh_job_cancel_all()
void rose_mesh_job_cancel_all( int wait = 1 );
The rose_mesh_job_cancel_all() function cancels all background jobs currently running or waiting to run. If a job is already running, this function will set a flag in the job that may cause it to end early. A non-zero value for the "wait" flag will force the function to block until jobs are no longer running.
rose_mesh_job_prioritize()
void rose_mesh_job_prioritize( RoseMesh * mesh ); void rose_mesh_job_prioritize( RoseMeshJob * job );
The rose_mesh_job_prioritize() function moves the given job or jobs for a mesh to the head of the queue so that they will be given a thread as early as possible.
rose_mesh_job_start()
int rose_mesh_job_start( RoseMesh * mesh ); int rose_mesh_job_start( RoseMeshJob * job );
The rose_mesh_job_start() function adds one job, or all of jobs in a mesh, to the queue for background execution. The function returns zero on error and nonzero on success. The STEP and IFC meshing libraries create a variety of background jobs for constructing mesh data in parallel.
Once a job is in the queue, it run when a thread is available. You can check the status of a particular job with the isDone() function or use the rose_mesh_job_wait() function to block execution until a job is finished.
rose_mesh_job_wait()
void rose_mesh_job_wait( RoseMesh * mesh ); void rose_mesh_job_wait( RoseMeshJob * job );
The rose_mesh_job_wait() function blocks until the given job is complete. It raises the priority of the job so that it will be given a thread as early as possible.
rose_mesh_job_wait_all()
void rose_mesh_job_wait_all();
The rose_mesh_job_wait_all() function waits until all background jobs have completed before returning.
rose_mesh_make_box()
void rose_mesh_make_box( RoseMesh * fs, const double xyz1[3], const double xyz2[3] ); void rose_mesh_make_box( RoseMesh * fs, const RosePoint pt1, const RosePoint pt2 ); void rose_mesh_make_box( RoseMesh * fs, double x1, double y1, double z1, double x2, double y2, double z2 ); void rose_mesh_make_box( RoseMesh * fs, const RoseBoundingBox& box );
The rose_mesh_make_box() function creates a faceted representation of a box. The resulting mesh is placed in the fs parameter. This can be used to generate simple data for testing or visualization.
Each side of the box is a pair of facets. They are created in a fixed order so you can attach faces afterwards if you wish.
- Facets 0-1 are the bottom face in the XY plane with the normal pointing in the -Z direction.
- Facets 2-3 are the face in the YZ plane at the max X coordinate with the normal pointing in the +X direction.
- Facets 4-5 are the face in the XZ plane at the max Y coordinate with the normal pointing in the +Y direction.
- Facets 6-7 are the face in the YZ plane at the min X coordinate with the normal pointing in the -X direction.
- Facets 8-9 are the face in the XZ plane at the min Y coordinate with the normal pointing in the -Y direction.
- Facets 10-11 are the top face in the XY plane with the normal pointing in the +Z direction.
The vertices are similarly created in a fixed order. The four vertices in the XY plane at min Z are created first, followed by the four at max Z. Within the plane, the min XY vertex is first moving counter-clockwise: (max X, min Y), (max X, max Y) and (min X, max Y).
rose_mesh_topology_dump()
void rose_mesh_topology_dump( RoseMeshTopology * topo, FILE * fd=stdout );
The rose_mesh_topology_dump() function dumps out the contents of a RoseMeshTopology object to an output stream. This can be used to analyse the topology object for debugging purposes.
rose_mesh_write_3mf()
int rose_mesh_write_3mf( const char * fname, const RoseMesh * fs, unsigned color=ROSE_MESH_NULL_COLOR );
The rose_mesh_write_3mf() function writes a complete mesh using the 3D Manufacturing format (3MF), which is an XML-based format. The function takes a filename amd writes a complete 3MF XML document. The function returns nonzero on success.
rose_mesh_write_amf()
int rose_mesh_write_amf( const char * fname, const RoseMesh * fs, unsigned color=ROSE_MESH_NULL_COLOR ); int rose_mesh_write_amf( RoseXMLWriter * xml, const RoseMesh * fs, unsigned color=ROSE_MESH_NULL_COLOR ); int rose_mesh_write_amf( RoseOutputStream * out, const RoseMesh * fs, unsigned color=ROSE_MESH_NULL_COLOR );
The rose_mesh_write_amf() function writes a complete mesh using the Additive manufacturing file format (AMF), which is an XML-based format. The function versions that take a filename or output stream write a complete AMF XML document while the version that takes an XML writer object just writes the <amf> element to the stream as part of a larger structure.
rose_mesh_write_stl()
int rose_mesh_write_stl( FILE * out, const RoseMesh * fs, int binary = 1 ); int rose_mesh_write_stl( const char * fname, const RoseMesh * fs, int binary = 1 );
The rose_mesh_write_stl() function writes a complete mesh using the STL file format. STL is a simple faceted model commonly used for 3D printing application.
rose_mesh_xml_read()
int rose_mesh_xml_read( RoseMeshShellRecordVec * shells, const char * fname );
The rose_mesh_xml_read() function reads a file containing multiple shells that have been written using the Mesh XML File Format. The function returns zero on error and nonzero on success.
You must add rosexml.lib
or librosexml.a
to your link line when using this function.
This function is primarily for debugging. Look at the capabilities in the STEP faceting library and the IFC faceting library for functions and applications that work with complete data sets.
rose_mesh_xml_read_shell()
int rose_mesh_xml_read_shell( RoseMeshShellRecord * rec, const char * fname );
The rose_mesh_xml_read_shell() function reads a file containing a single shell that is written using the Mesh XML File Format. The function returns zero on error and nonzero on success.
You must add rosexml.lib
or librosexml.a
to your link line when using this function.
This function is primarily for debugging. Look at the capabilities in the STEP faceting library and the IFC faceting library for functions and applications that work with complete data sets.
rose_mesh_xml_read_shell_element()
typedef void (*RoseMeshXMLCompleteFn) (XML_Parser xp); void rose_mesh_xml_read_shell_element( XML_Parser xp, RoseMeshShellRecord * rec, RoseMeshXMLCompleteFn fn ); void rose_mesh_xml_start_shell_element( XML_Parser xp, RoseMeshShellRecord * rec, RoseMeshXMLCompleteFn fn, const char * el_name, const char ** el_atts );
The rose_mesh_xml_read_shell_element() and rose_mesh_xml_start_shell_element() are internal functions used to embed a reader for the shell element within a larger XML document. These functions are only defined if the "rose_expat.h" header has been included.
You must add rosexml.lib
or librosexml.a
to your link line when using this function.
rose_mesh_xml_save()
void rose_mesh_xml_save( RoseXMLWriter * xml, const RoseMesh * shell, const char * id=0, unsigned color=ROSE_MESH_NULL_COLOR ); void rose_mesh_xml_save( RoseXMLWriter * xml, RoseMeshXMLOpts * opts, const RoseMesh * shell, const char * id=0, unsigned color=ROSE_MESH_NULL_COLOR ); void rose_mesh_xml_save( RoseOutputStream * out, RoseMeshXMLOpts * opts, const RoseMesh * shell, const char * id=0, unsigned color=ROSE_MESH_NULL_COLOR ); void rose_mesh_xml_save( RoseOutputStream * out, const RoseMesh * shell, const char * id=0, unsigned color=ROSE_MESH_NULL_COLOR ); void rose_mesh_xml_save( const char * fname, const RoseMesh * shell, const char * id=0, unsigned color=ROSE_MESH_NULL_COLOR ); void rose_mesh_xml_save( const char * fname, RoseMeshXMLOpts * opts, const RoseMesh * shell, const char * id=0, unsigned color=ROSE_MESH_NULL_COLOR ); struct RoseMeshXMLOpts { int write_facet_normals; RoseMeshXMLOpts(); };
The rose_mesh_xml_save() function writes a complete mesh using the Mesh XML File Format. The version that takes a filename or output stream writes a complete XML document while the version that takes an XML writer object just writes the <shell> element to the stream as part of a larger structure.
This function is primarily for debugging. Look at the capabilities in the STEP faceting library and the IFC faceting library for functions and applications to write complete data sets.
Be aware when writing facet sets that have faces defined on them, only the facets associated with a face will be written. Use rose_mesh_xml_save_no_faces() to ignore the faces and write all facets.
The RoseMeshXMLOpts structure contains options for the XML output. If the write_facet_normals field is true, the XML output will include a nested <fn> element on each <f> (facet) element. The contents of the <fn> is a list of three numbers giving the normal of the facet. By default the facet normal is not stored, since it can be computed from the facet values.
rose_mesh_xml_save_face()
void rose_mesh_xml_save_face( RoseXMLWriter * xml, const RoseMesh * shell, const RoseMeshFace * face ); void rose_mesh_xml_save_face( RoseXMLWriter * xml, RoseMeshXMLOpts * opts, const RoseMesh * shell, const RoseMeshFace * face );
The rose_mesh_xml_save_face() function writes a single <facets> element to an XML stream. It is an internal utility used by rose_mesh_xml_save().
rose_mesh_xml_save_facets()
void rose_mesh_xml_save_facets( RoseXMLWriter * xml, const RoseMesh * shell, rose_uint_vector * facet_list ); void rose_mesh_xml_save_facets( RoseXMLWriter * xml, const RoseMesh * shell, unsigned first, unsigned sz, const char ** extra_atts );
The rose_mesh_xml_save_facets() function writes a single <facets> element to an XML stream. It is an internal utility used by rose_mesh_xml_save().
rose_mesh_xml_save_footer()
void rose_mesh_xml_save_footer( RoseXMLWriter * xml ); void rose_mesh_xml_save_footer( RoseXMLWriter * xml, RoseMeshXMLOpts * opts );
The rose_mesh_xml_save_footer() function is an internal utility used by rose_mesh_xml_save(). It simply closes a <shell> element in an XML stream. See rose_mesh_xml_save_header() for the companion open element operation.
rose_mesh_xml_save_fragment()
void rose_mesh_xml_save_fragment( RoseXMLWriter * xml, const RoseMesh * shell, rose_uint_vector * facets ); void rose_mesh_xml_save_fragment( RoseXMLWriter * xml, RoseMeshXMLOpts * opts, const RoseMesh * shell, rose_uint_vector * facets ); void rose_mesh_xml_save_fragment( RoseOutputStream * out, const RoseMesh * shell, rose_uint_vector * facets ); void rose_mesh_xml_save_fragment( RoseOutputStream * out, RoseMeshXMLOpts * opts, const RoseMesh * shell, rose_uint_vector * facets ); void rose_mesh_xml_save_fragment( const char * fname, const RoseMesh * shell, rose_uint_vector * facets ); void rose_mesh_xml_save_fragment( const char * fname, RoseMeshXMLOpts * opts, const RoseMesh * shell, rose_uint_vector * facets );
The rose_mesh_xml_save_fragment() function is an internal utility used primarily for debugging. It saves a collection of facets as a <fragment> element in an XML stream or separate file.
rose_mesh_xml_save_header()
void rose_mesh_xml_save_header( RoseXMLWriter * xml, const RoseMesh * shell, const char * id, unsigned color=ROSE_MESH_NULL_COLOR ); void rose_mesh_xml_save_header( RoseXMLWriter * xml, RoseMeshXMLOpts * opts, const RoseMesh * shell, const char * id, unsigned color=ROSE_MESH_NULL_COLOR );
The rose_mesh_xml_save_header() function is an internal utility used by rose_mesh_xml_save(). It simply opens a <shell> element in an XML stream. See rose_mesh_xml_save_footer() for the companion close element operation.
rose_mesh_xml_save_no_faces()
void rose_mesh_xml_save_no_faces( RoseXMLWriter * xml, const RoseMesh * shell, const char * id=0, unsigned color=ROSE_MESH_NULL_COLOR ); void rose_mesh_xml_save_no_faces( RoseXMLWriter * xml, RoseMeshXMLOpts * opts, const RoseMesh * shell, const char * id=0, unsigned color=ROSE_MESH_NULL_COLOR ); void rose_mesh_xml_save_no_faces( RoseOutputStream * out, const RoseMesh * shell, const char * id=0, unsigned color=ROSE_MESH_NULL_COLOR ); void rose_mesh_xml_save_no_faces( RoseOutputStream * out, RoseMeshXMLOpts * opts, const RoseMesh * shell, const char * id=0, unsigned color=ROSE_MESH_NULL_COLOR ); void rose_mesh_xml_save_no_faces( const char * fname, const RoseMesh * shell, const char * id=0, unsigned color=ROSE_MESH_NULL_COLOR ); void rose_mesh_xml_save_no_faces( const char * fname, RoseMeshXMLOpts * opts, const RoseMesh * shell, const char * id=0, unsigned color=ROSE_MESH_NULL_COLOR );
The rose_mesh_xml_save_no_faces() function writes a complete mesh using the Mesh XML File Format. The version that takes a filename or output stream writes a complete XML document while the version that takes an XML writer object just writes the <shell> element to the stream as part of a larger structure.
This function is similar to rose_mesh_xml_save(), but ignores all face data and simply writes all facets in a single collection.