Make Fishhead 5-Axis Process
The sample program below creates a 5-Axis machining process for the Fishhead test part, with tolerances, setup and fixturing, tool geometry, and intermediate stage models.
Note: these programs will run, but saving the model requires a license key from STEP Tools.
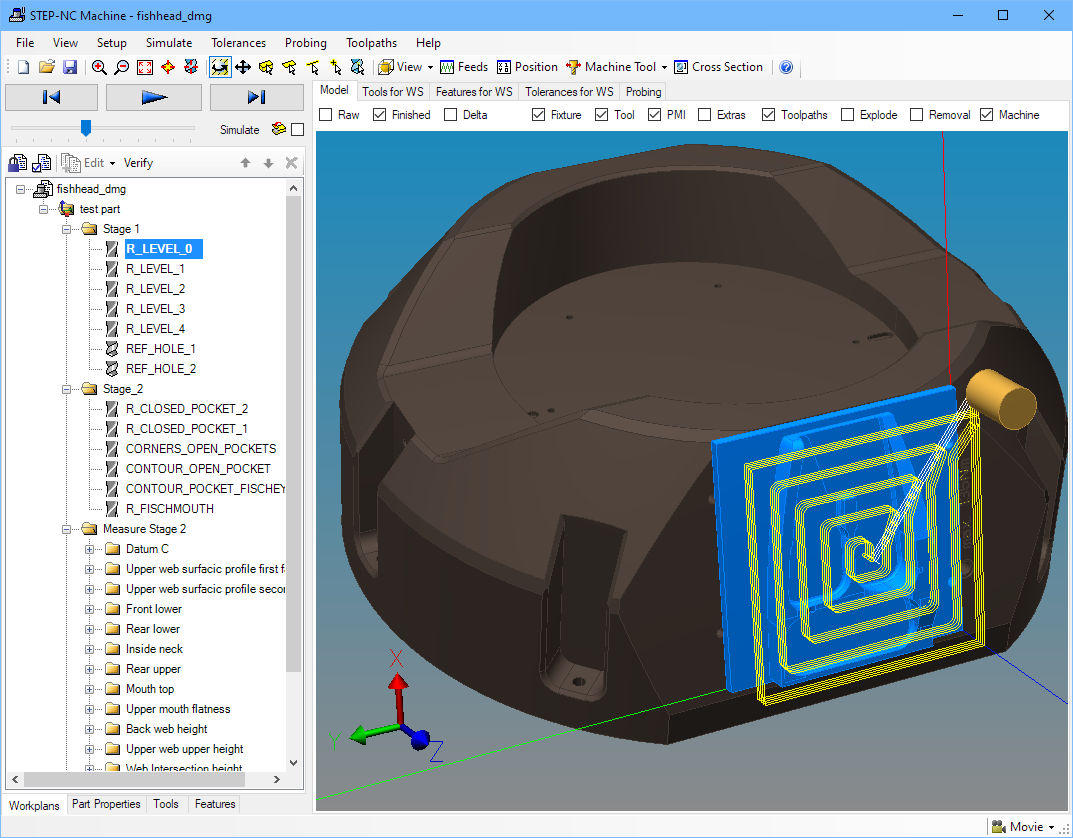
#! python # # Make Airbus Fishhead Digital Twin Model # import sys import os from steptools import step from steptools.step import AptAPI as apt from steptools.step import ToleranceAPI as tol step.verbose(False) apt.auto_workingstep_tool() apt.set_make_display_messages(False) apt.partno(test part) apt.millimeters() print(Creating Stage 1) apt.nest_workplan(Stage 1) apt.spindle_speed_cw(10026) apt.rapid() apt.read_ugs_aptcl(Stage_1.cls) plan_id = apt.get_current_workplan() stage_1_id = apt.executable_workpiece_tobe(plan_id,Airbus_Fish_Stage_1_To-Be.stp) apt.put_workpiece_placement(stage_1_id, 7.62, 161.925, 12.7) apt.end_workplan() apt.set_name_in_plan(plan_id, 0,R_LEVEL_0) apt.set_name_in_plan(plan_id, 1,R_LEVEL_1) apt.set_name_in_plan(plan_id, 2,R_LEVEL_2) apt.set_name_in_plan(plan_id, 3,R_LEVEL_3) apt.set_name_in_plan(plan_id, 4,R_LEVEL_4) apt.set_name_in_plan(plan_id, 5,REF_HOLE_1) apt.set_name_in_plan(plan_id, 6,REF_HOLE_2) apt.set_spindle_speed_for_feed_cw(9223.92, 10026) apt.set_spindle_speed_for_feed_cw(501.3, 5013) # Set workpiece models for main plan raw_id = apt.rawpiece(Airbus_Fish_Stage_1_As-Is.stp) apt.put_workpiece_placement(raw_id, 0, 0, 12.7) wp_id = apt.workpiece(Airbus_Fish_PMI_inches.stp) apt.put_workpiece_placement(wp_id, 7.62, 161.925, 12.7) tol.add_workpiece_material (wp_id,EN AW-7075 (3.4365 or AlZn5,5MgCu,ASM) tol.add_workpiece_hardness (wp_id, 30,ROCKWELL) print(Creating Stage 2) apt.nest_workplan(Stage_2) apt.spindle_speed_cw(1026) apt.rapid() apt.read_ugs_aptcl(Stage_2.cls) plan_id = apt.get_current_workplan() apt.set_name_in_plan(plan_id, 0,R_CLOSED_POCKET_2) apt.set_name_in_plan(plan_id, 1,R_CLOSED_POCKET_1) apt.set_name_in_plan(plan_id, 2,CORNERS_OPEN_POCKETS) apt.set_name_in_plan(plan_id, 3,CONTOUR_OPEN_POCKET) apt.set_name_in_plan(plan_id, 4,CONTOUR_POCKET_FISCHEYE) apt.set_name_in_plan(plan_id, 5,R_FISCHMOUTH) apt.set_spindle_speed_for_feed_cw(2291.76, 10862) # Set workpiece models for machining plan apt.executable_workpiece_reuse_asis(plan_id, stage_1_id) stage_2_id = apt.executable_workpiece_tobe(plan_id,Airbus_Fish_Stage_2_To-Be_PMI_R2016_INCH2.stp) apt.put_workpiece_placement(stage_2_id, 7.62, 161.925, 12.7) tol.set_tolerance_name_in_workpiece(stage_2_id, 0,Neck back perpendicularity) tol.set_tolerance_name_in_workpiece(stage_2_id, 1,Back web height) tol.set_tolerance_name_in_workpiece(stage_2_id, 2,Upper web height) tol.set_tolerance_name_in_workpiece(stage_2_id, 3,Web intersection height) tol.set_tolerance_name_in_workpiece(stage_2_id, 4,Front center web height) tol.set_tolerance_name_in_workpiece(stage_2_id, 5,Upper mouth flatness) tol.set_tolerance_name_in_workpiece(stage_2_id, 6,Datum C surface position) tol.set_tolerance_name_in_workpiece(stage_2_id, 7,Lower mouth surface position) tol.set_tolerance_name_in_workpiece(stage_2_id, 8,Rear lower surface position) tol.set_tolerance_name_in_workpiece(stage_2_id, 9,Neck front surface position) tol.set_tolerance_name_in_workpiece(stage_2_id, 10,Rear upper surface position) tol.set_tolerance_name_in_workpiece(stage_2_id, 11,Lower web outside surface position) tol.set_tolerance_name_in_workpiece(stage_2_id, 12,Lower mouth surface position) tol.set_tolerance_name_in_workpiece(stage_2_id, 13,Upper mouth surface position) tol.set_tolerance_name_in_workpiece(stage_2_id, 14,Lower rear surface position) tol.set_tolerance_name_in_workpiece(stage_2_id, 15,Upper web outside surface position) tol.set_tolerance_name_in_workpiece(stage_2_id, 16,Jaw surface position) tol.set_tolerance_name_in_workpiece(stage_2_id, 17,Upper rear surface position) apt.end_workplan() # Define probing operations for measuring plan print(Creating Measure Stage 2) apt.nest_workplan(Measure Stage 2) measure2_plan_id = apt.get_current_workplan() tol.plan_set_start_clear(15, 60) tol.plan_using_clear_at_start_end_only() apt.retract_plane(100) tol2_id = apt.get_id_from_uuid(c966dcba-fe85-48ee-bfc2-508560a8b8fb) face2_bag = tol.get_tolerance_face_all(tol2_id) tol.plan_plane_probing(measure2_plan_id, 1,Datum C, face2_bag[0], 2, 3) tol2_id = apt.get_id_from_uuid(dc162777-a542-4a6d-a44c-d1a12c452fd0) face2_bag = tol.get_tolerance_face_all(tol2_id) tol.plan_any_probing(measure2_plan_id, 2,Upper web surfacic profile first face, face2_bag[0], 10, 2) tol.plan_any_probing(measure2_plan_id, 3,Upper web surfacic profile second face, face2_bag[1], 4, 2) tol2_id = apt.get_id_from_uuid(ba600bd2-b7e3-467b-86e8-70c60d7a08ac) face2_bag = tol.get_tolerance_face_all(tol2_id) tol.plan_plane_probing(measure2_plan_id, 4,Front lower, face2_bag[0], 3, 3) tol2_id = apt.get_id_from_uuid(a3b6fe46-d52e-4b59-b167-f8ea57f44cf3) face2_bag = tol.get_tolerance_face_all(tol2_id) tol.plan_plane_probing(measure2_plan_id, 5,Rear lower, face2_bag[0], 2, 2) tol.plan_using_clear_always() tol2_id = apt.get_id_from_uuid(a7d279cb-03fe-4f8d-9da3-dde2d7d2852b) face2_bag = tol.get_tolerance_face_all(tol2_id) tol.plan_plane_probing(measure2_plan_id, 6,Inside neck, face2_bag[0], 2, 4) tol2_id = apt.get_id_from_uuid(df260d28-776b-4e01-af86-e0e2388b7cc6) face2_bag = tol.get_tolerance_face_all(tol2_id) tol.plan_plane_probing(measure2_plan_id, 8,Rear upper, face2_bag[0], 2, 2) apt.nest_workplan(Mouth top) mouth_plan_id = apt.get_current_workplan() tol2_id = apt.get_id_from_uuid(cdbe37f6-9da3-4b25-b6fb-f7aa8676c9a1) face2_bag = tol.get_tolerance_face_all(tol2_id) tol.plan_any_probing(mouth_plan_id, 1,Mouth top 1, face2_bag[0], 8, 2) tol.plan_any_probing(mouth_plan_id, 2,Mouth top 2, face2_bag[1], 2, 2) tol.plan_any_probing(mouth_plan_id, 3,Mouth top 3, face2_bag[2], 5, 2) tol.plan_any_probing(mouth_plan_id, 4,Mouth top 4, face2_bag[3], 5, 2) tol.plan_any_probing(mouth_plan_id, 5,Mouth top 5, face2_bag[4], 5, 2) tol.plan_any_probing(mouth_plan_id, 6,Mouth top 6, face2_bag[5], 2, 1) tol.plan_any_probing(mouth_plan_id, 7,Mouth top 7, face2_bag[11], 4, 1) tol.plan_any_probing(mouth_plan_id, 8,Mouth top 8, face2_bag[10], 1, 0.5) tol.plan_any_probing(mouth_plan_id, 9,Mouth top 9, face2_bag[9], 4, 1) tol.plan_any_probing(mouth_plan_id, 10,Mouth top 10, face2_bag[8], 1, 1) tol.plan_any_probing(mouth_plan_id, 11,Mouth top 11, face2_bag[7], 2, 1) tol.plan_any_probing(mouth_plan_id, 12,Mouth top 12, face2_bag[6], 2, 1) tol.plan_any_probing(mouth_plan_id, 13,Mouth top 13, face2_bag[12], 1, 1) tol.plan_any_probing(mouth_plan_id, 14,Mouth top 14, face2_bag[13], 1, 1) tol.plan_any_probing(mouth_plan_id, 15,Mouth top 15, face2_bag[14], 1, 0.5) tol.plan_any_probing(mouth_plan_id, 16,Mouth top 16, face2_bag[15], 3, 2) tol.plan_any_probing(mouth_plan_id, 17,Mouth top 17, face2_bag[16], 4, 2) apt.end_workplan() tol2_id = apt.get_id_from_uuid(b8faa9e6-7ed5-415d-a45a-b0a9dac0fea4) face2_bag = tol.get_tolerance_face_all(tol2_id) tol.plan_any_probing(measure2_plan_id, 10,Upper mouth flatness, face2_bag[0], 8, 5) tol2_id = apt.get_id_from_uuid(41e7d222-9acb-4f2e-b658-5a54627ac116) face2_bag = tol.get_tolerance_target_face_all(tol2_id) tol.plan_plane_probing(measure2_plan_id, 11,Back web height, face2_bag[0], 3, 2) tol2_id = apt.get_id_from_uuid(e0f502a8-bbd5-433e-8787-b791b6daf19c) face2_bag = tol.get_tolerance_target_face_all(tol2_id) tol.plan_any_probing(measure2_plan_id, 13,Upper web upper height, face2_bag[0], 10, 0.5) tol2_id = apt.get_id_from_uuid(0b830617-8376-4551-b2c9-27930612b3b2) face2_bag = tol.get_tolerance_target_face_all(tol2_id) tol.plan_any_probing(measure2_plan_id, 14,Web Intersection height, face2_bag[0], 6, 1) tol2_id = apt.get_id_from_uuid(8db82bc9-b275-412b-804f-5a4661227539) face2_bag = tol.get_tolerance_target_face_all(tol2_id) tol.plan_set_delta_uv(0, 0) tol.plan_plane_probing(measure2_plan_id, 15,Front center web height, face2_bag[0], 2, 3) apt.end_workplan() print(Creating Stage 3) apt.nest_workplan(Stage 3) apt.rapid() apt.read_ugs_aptcl(Stage_3.cls) plan_id = apt.get_current_workplan() raw_id = apt.executable_workpiece_asis(plan_id,Airbus_Fish_Stage_2_To-Be_with_base.step) apt.put_workpiece_placement(raw_id, 7.62, 161.925, 12.7) stage_3_id = apt.executable_workpiece_tobe(plan_id,Airbus_Fish_Stage_3_To-Be.stp) apt.put_workpiece_placement(stage_3_id, 7.62, 161.925, 12.7) apt.end_workplan() apt.set_name_in_plan(plan_id, 0,OUT_5XWALL_HIGH_STAGE_3) apt.set_name_in_plan(plan_id, 1,OUT_5XWALL_SHORT_STAGE_3) apt.set_name_in_plan(plan_id, 2,LONG_ANGLED_FACE_HIGH_WALL_STAGE_3) apt.set_name_in_plan(plan_id, 3,SHORT_ANGLED_FACE_HIGH_WALL_STAGE_3) apt.set_name_in_plan(plan_id, 4,RADIUS_FRONT_STAGE_3) apt.set_name_in_plan(plan_id, 5,FISHEYE_STAGE_3) apt.set_name_in_plan(plan_id, 6,IN_SHORT_ANGLED_FACE_STAGE_3) apt.set_name_in_plan(plan_id, 7,IN_LONG_ANGLED_STAGE_3) apt.set_name_in_plan(plan_id, 8,R_CENTER_ANGLED_FACE_STAGE_3) apt.set_name_in_plan(plan_id, 9,ROUGH_CORNER) apt.set_name_in_plan(plan_id, 10,IN_WALL_ANGLED_OPEN_STAGE_3) apt.set_name_in_plan(plan_id, 11,IN_FLOOR_ANGLED_OPEN_STAGE_3) apt.set_name_in_plan(plan_id, 12,IN_WALL_ANGLED_CLOSED_A_STAGE_3) apt.set_name_in_plan(plan_id, 13,IN_FLOOR_ANGLED_CLOSED_A_STAGE_3) apt.set_name_in_plan(plan_id, 14,IN_WALL_ANGLED_FISHEYE_STAGE_3) apt.set_name_in_plan(plan_id, 15,IN_FLOOR_ANGLED_FISHEYE_STAGE_3) apt.set_name_in_plan(plan_id, 16,IN_WALL_ANGLED_CLOSED_B_STAGE_3) apt.set_name_in_plan(plan_id, 17,IN_FLOOR_ANGLED_CLOSED_B_STAGE_3) apt.set_name_in_plan(plan_id, 18,FLOOR_FISHMOUTH_STAGE_3) apt.set_name_in_plan(plan_id, 19,IN_FLOOR_POCKET_OPEN_STAGE_3) apt.set_name_in_plan(plan_id, 20,IN_FLOOR_A_POCKET_FISHEYE_STAGE_3) apt.set_name_in_plan(plan_id, 21,IN_FLOOR_B_POCKET_FISHEYE_STAGE_3) apt.set_name_in_plan(plan_id, 22,IN_FLOOR_POCKET_PIN_STAGE_3) apt.set_spindle_speed_for_feed_cw(2291.76, 10862) apt.set_spindle_speed_for_feed_cw(1909.92, 11937) print(Creating Stage 4) apt.nest_workplan(Stage 4) apt.rapid() apt.read_ugs_aptcl(Stage_4.cls) # Set workpiece models for machining plan plan_id = apt.get_current_workplan() apt.executable_workpiece_reuse_asis(plan_id, stage_3_id) apt.executable_workpiece_reuse_tobe(plan_id, wp_id) apt.end_workplan() apt.set_name_in_plan(plan_id, 0,OUT_5XWALL_HIGH) apt.set_name_in_plan(plan_id, 1,OUT_5XWALL_HIGH_F) apt.set_name_in_plan(plan_id, 2,OUT_5XWALL_SHORT) apt.set_name_in_plan(plan_id, 3,OUT_WALL_STRAIGHT) apt.set_name_in_plan(plan_id, 4,OUT_FISHMOUTH) apt.set_name_in_plan(plan_id, 5,LONG_ANGLED_FACE_HIGH_WALL_SF) apt.set_name_in_plan(plan_id, 6,LONG_ANGLED_FACE_HIGH_WALL_F) apt.set_name_in_plan(plan_id, 7,TOP_HIGH_WALL) apt.set_name_in_plan(plan_id, 8,TOP_LOW_WALL_A) apt.set_name_in_plan(plan_id, 9,RADIUS_FRONT) apt.set_name_in_plan(plan_id, 10,TOP_LOW_WALL_B) apt.set_name_in_plan(plan_id, 11,SHORT_ANGLED_FACE_HIGH_WALL) apt.set_name_in_plan(plan_id, 12,TOP_FISHEYE) apt.set_name_in_plan(plan_id, 13,WALL_FISHEYE) apt.set_name_in_plan(plan_id, 14,TOP_CENTER_A) apt.set_name_in_plan(plan_id, 15,IN_LONG_ANGLED_FACE) apt.set_name_in_plan(plan_id, 16,IN_SHORT_ANGLED_FACE) apt.set_name_in_plan(plan_id, 17,CENTRE_ANGLED_FACE) apt.set_name_in_plan(plan_id, 18,CENTRE_TOP_A) apt.set_name_in_plan(plan_id, 19,CENTRE_TOP_B) apt.set_name_in_plan(plan_id, 20,CENTRE_TOP_C) apt.set_name_in_plan(plan_id, 21,CENTRE_TOP_D) apt.set_name_in_plan(plan_id, 22,CENTRE_TOP_D_COPY) apt.set_name_in_plan(plan_id, 23,FLOOR_FISHMOUTH) apt.set_name_in_plan(plan_id, 24,IN_WALL_POCKET_FISHEYE) apt.set_name_in_plan(plan_id, 25,IN_WALL_POCKET_OPEN) apt.set_name_in_plan(plan_id, 26,IN_WALL_POCKET_CLOSED_1) apt.set_name_in_plan(plan_id, 27,IN_WALL_POCKET_CLOSED_2) apt.set_name_in_plan(plan_id, 28,IN_WALL_ANGLED_FISHEYE_SF) apt.set_name_in_plan(plan_id, 29,IN_WALL_ANGLED_FISHEYE_F) apt.set_name_in_plan(plan_id, 30,IN_WALL_ANGLED_OPEN_SF) apt.set_name_in_plan(plan_id, 31,IN_WALL_ANGLED_OPEN_F) apt.set_name_in_plan(plan_id, 32,IN_FLOOR_ANGLED_OPEN_POCKET) apt.set_name_in_plan(plan_id, 33,IN_WALL_ANGLED_CLOSED_POCKET_1) apt.set_name_in_plan(plan_id, 34,IN_WALL_ANGLED_CLOSED_POCKET_2) apt.set_name_in_plan(plan_id, 35,IN_FLOOR_POCKET_CLOSED_1) apt.set_name_in_plan(plan_id, 36,IN_FLOOR_POCKET_CLOSED_2) apt.set_name_in_plan(plan_id, 37,IN_FLOOR_POCKET_FISHEYE) apt.set_name_in_plan(plan_id, 38,IN_FLOOR_POCKET_OPEN) apt.set_name_in_plan(plan_id, 39,IN_FLOOR_FISHMOUTH_PIN) apt.set_name_in_plan(plan_id, 40,IN_FLOOR_FISHMOUTH_CONTOUR) apt.set_name_in_plan(plan_id, 41,DEBUR_A) apt.set_name_in_plan(plan_id, 42,DEBUR_B) apt.set_name_in_plan(plan_id, 43,DEBUR_C) apt.set_name_in_plan(plan_id, 44,DEBUR_E) apt.set_name_in_plan(plan_id, 45,DEBUR_F) apt.set_name_in_plan(plan_id, 46,DEBUR_G) apt.set_name_in_plan(plan_id, 47,DEBUR_H) apt.set_name_in_plan(plan_id, 48,DEBUR_I) apt.set_name_in_plan(plan_id, 49,DEBUR_J) apt.set_name_in_plan(plan_id, 50,DEBUR_K) apt.set_name_in_plan(plan_id, 51,DEBUR_L) apt.set_name_in_plan(plan_id, 52,DEBUR_M) apt.set_name_in_plan(plan_id, 53,DEBUR_N) apt.set_name_in_plan(plan_id, 54,DEBUR_O) apt.set_name_in_plan(plan_id, 55,DEBUR_P) apt.set_spindle_speed_for_feed_cw(2864.88, 7958) apt.set_spindle_speed_for_feed_cw(9549.6, 9549) # Define probing operations for measuring plan print(Creating Measure stage 4) apt.nest_workplan(Measure stage 4) measure_plan_id = apt.get_current_workplan() tol.plan_set_start_clear(15, 60) tol.plan_using_clear_at_start_end_only() apt.retract_plane(100) tol_id = apt.get_id_from_uuid(24ce6e4d-7ce5-4241-bf13-a4c7f4ebc5a6) face_bag = tol.get_tolerance_target_face_all(tol_id) tol.plan_any_probing(measure_plan_id, 1,Top web thickness first face, face_bag[0], 5, 3) face_bag = tol.get_tolerance_origin_face_all(tol_id) tol.plan_any_probing(measure_plan_id, 2,Top web thickness second face, face_bag[0], 5, 3) tol_id = apt.get_id_from_uuid(09a905e5-da9c-44ab-aa9b-48689aafcd59) face_bag = tol.get_tolerance_target_face_all(tol_id) tol.plan_any_probing(measure_plan_id, 3,Front web thickness first face, face_bag[0], 5, 3) face_bag = tol.get_tolerance_origin_face_all(tol_id) tol.plan_any_probing(measure_plan_id, 4,Front web thickness second face, face_bag[0], 5, 3) tol_id = apt.get_id_from_uuid(4a802bdf-88ba-4d94-889e-4606a17b650b) face_bag = tol.get_tolerance_target_face_all(tol_id) tol.plan_any_probing(measure_plan_id, 5,Rear web thickness first face, face_bag[0], 5, 1) face_bag = tol.get_tolerance_origin_face_all(tol_id) tol.plan_any_probing(measure_plan_id, 6,Rear web thickness second face, face_bag[0], 5, 1) tol_id = apt.get_id_from_uuid(f9552ef3-ba13-490c-8b55-8c6562c2ab9b) face_bag = tol.get_tolerance_origin_face_all(tol_id) tol.plan_any_probing(measure_plan_id, 7,Fish eye top, face_bag[0], 15, 0.5) tol_id = apt.get_id_from_uuid(f9552ef3-ba13-490c-8b55-8c6562c2ab9b) face_bag = tol.get_tolerance_target_face_all(tol_id) tol.plan_any_probing(measure_plan_id, 8,Upper web upper height, face_bag[0], 15, 1) tol_id = apt.get_id_from_uuid(2038fe7f-4990-4a5d-9d19-6e0626e38809) face_bag = tol.get_tolerance_origin_face_all(tol_id) tol.plan_set_delta_uv(2, 0) tol.plan_plane_probing(measure_plan_id, 9,Front web height, face_bag[0], 4, 3) tol_id = apt.get_id_from_uuid(e95b2341-93f4-44a8-8b6f-e36d1e82feab) face_bag = tol.get_tolerance_origin_face_all(tol_id) tol.plan_plane_probing(measure_plan_id, 10,Back web height, face_bag[0], 5, 3) tol_id = apt.get_id_from_uuid(0098e447-15ab-44ac-b360-3d5fd4f7dcd3) face_bag = tol.get_tolerance_origin_face_all(tol_id) tol.plan_set_delta_uv(0, 0) tol.plan_plane_probing(measure_plan_id, 11,Front center web height, face_bag[0], 2, 6) tol_id = apt.get_id_from_uuid(15e5b82d-b448-4e1c-a218-71c9c8796e49) face_bag = tol.get_tolerance_origin_face_all(tol_id) tol.plan_plane_probing(measure_plan_id, 12,Front upper height, face_bag[0], 4, 4) tol_id = apt.get_id_from_uuid(952ebe43-c024-4cd8-a1ac-2079dab0add0) face_bag = tol.get_tolerance_origin_face_all(tol_id) tol.plan_plane_probing(measure_plan_id, 13,Rear upper height, face_bag[0], 2, 2) tol_id = apt.get_id_from_uuid(3e80ee27-e27e-4064-8106-44b400797b43) face_bag = tol.get_tolerance_origin_face_all(tol_id) tol.plan_plane_probing(measure_plan_id, 14,Rear lower height, face_bag[0], 2, 2) tol_id = apt.get_id_from_uuid(547b6d79-f773-4db8-9a0e-2194ee5d37b2) face_bag = tol.get_tolerance_origin_face_all(tol_id) tol.plan_plane_probing(measure_plan_id, 15,Front lower height, face_bag[0], 2, 2) tol_id = apt.get_id_from_uuid(04d1431a-9535-4ef6-a615-f00315a87548) face_bag = tol.get_tolerance_origin_face_all(tol_id) tol.plan_plane_probing(measure_plan_id, 16,Web Intersection, face_bag[0], 12, 3) tol_id = apt.get_id_from_uuid(1f78537f-87ce-4dca-9c8c-a2c9a32fa248) face_bag = tol.get_tolerance_origin_face_all(tol_id) tol.plan_plane_probing(measure_plan_id, 17,Eye Bulge, face_bag[0], 5, 5) tol_id = apt.get_id_from_uuid(3c9773de-5015-4c05-86b4-0e35a5bfd96f) face_bag = tol.get_tolerance_face_all(tol_id) tol.plan_cylinder_probing(measure_plan_id, 18,Fish Eye cylinder, face_bag[0], 1, 8) tol_id = apt.get_id_from_uuid(5457a338-5b91-4c5f-bf62-658fab83cbe0) face_bag = tol.get_tolerance_face_all(tol_id) tol.plan_plane_probing(measure_plan_id, 19,Neck Flatness, face_bag[0], 2, 4) tol_id = apt.get_id_from_uuid(d37d68b4-4030-4792-8438-879be508a1a3) face_bag = tol.get_tolerance_face_all(tol_id) tol.plan_bspline_probing(measure_plan_id, 20,Upper web surfacic profile first face, face_bag[0], 3, 2) tol.plan_bspline_probing(measure_plan_id, 21,Upper web surfacic profile second face, face_bag[1], 5, 2) apt.set_tool_length(1, 140.098) apt.set_tool_length(3, 199.406) apt.set_tool_length(4, 174.037) apt.set_tool_length(2, 256.423) # Boost speed of stage 1 simulation apt.set_tool_radius(1, 0) # probe apt.set_tool_length(-99, 189.007) pb_id = apt.geometry_for_tool_number(DMG_probe_longer.step, -99) apt.put_workpiece_placement(pb_id, 0, 0, 186.007) apt.generate_all_tool_geometry() apt.end_workplan() outer_plan_id = apt.get_current_workplan() # Fixture G apt.workplan_setup ( outer_plan_id, -161.52, 407.959, 61.924, 0, 0.9659258, 0.258819, 0, -0.258819, 0.9659528) # Fixture F # apt.workplan_setup( # outer_plan_id, # 161.52, -407.959, 61.924, # 0, -0.9659258, 0.258819, # 0, 0.258819, 0.9659528) apt.fixture(Fixture_with_datums.stp) apt.save_project(fishhead_dmg)
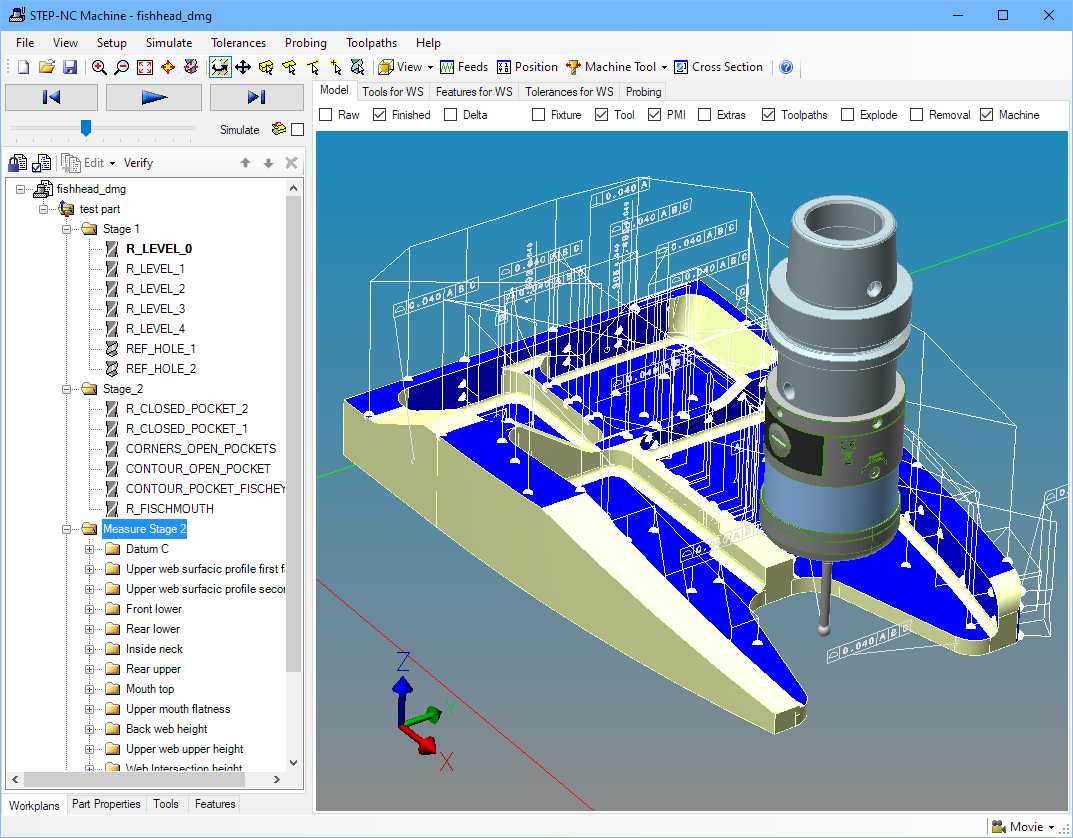