Overview
In the STEP schema, semantic tolerances use the geometric_tolerance definition. The schema has subtypes for each different constraint, plus more subtypes for extra parameters. A tolerance instance must use a combination of these subtypes depending on the extra parameters that are used.
These AND/OR combinations are usually handled with an extra C++ class for each combination. This is not practical for the tolerance schema which, because of the "mix-in" types for each extra tolerance parameter, would require almost 260 extra C++ classes! The functions below create tolerances and access parameters in a much simpler way.
The make function takes a bit flag to indicate what extra tolerance parameters to support. Each parameter is handled by a subtype so you must choose them at create time. The make function handles all AND/OR combination details and returns the result as a stp_geometric_tolerance pointer.
The get functions just return null if an object can not
hold the extra tolerance parameter. The put functions return
a status value of ROSE_OK
(zero) if it succeeds, or a nonzero
error code if the tolerance object is null or can not hold the
parameter. There are also add functions to simplify appending a value
to sets of datums or sets of modifiers.
RoseDesign * d; stp_shape_aspect * callout; stp_geometric_tolerance * gt = stix_tol_make( d, STIX_TOL_FLATNESS + STIX_TOL_WITH_MODS ); gt-> name(flatness tolerance with modifiers); gt-> description(""); gt-> magnitude(stix_measure_make_length(d, 0.2, roseunit_mm)); stix_tol_put_aspect(gt, callout); stix_tol_add_modifier( gt,stp_geometric_tolerance_modifier_maximum_material_requirement );
Datum Systems
Geometric tolerances can reference a datum system, which contains an ordered list of datums, possibly with modifiers. One datum system can be referenced by many tolerances and is an instance of the STEP datum_system definition.
Use the stix_tol_make_datum_system() function to create a datum system instance, then associate it with a tolerance using the stix_tol_put_datums() and stix_tol_get_datums() functions.
Add datum to a system with the stix_tol_add_datum() function, and then apply any modifiers to the datum with the stix_tol_add_datum_modifier() function.
Iterate over datums and their modifiers using the stix_tol_get_datum() and stix_tol_get_datum_modifier() functions. These functions understand datums that are attached using the current AP242 datum system, but will also work the older AP214 style datum reference. See the function documentation for code examples.
RoseDesign * d; stp_shape_aspect * callout; stp_product_definition_shape * shape = callout->of_shape(); stp_datum * d1 = stix_tol_make_datum(A, shape); stp_datum * d2 = stix_tol_make_datum(B, shape); stp_datum * d3 = stix_tol_make_datum(C, shape); // datum system A(M) B C stp_datum_system * sys = stix_tol_make_datum_system(shape); stix_tol_add_datum(sys, d1); stix_tol_add_datum_modifier(sys, d1, stp_simple_datum_reference_modifier_maximum_material_requirement ); stix_tol_add_datum(sys, d2); stix_tol_add_datum(sys, d3); stp_geometric_tolerance * gt = stix_tol_make( d, STIX_TOL_POSITION + STIX_TOL_WITH_DATUMS ); gt-> name(position tolerance with datums); gt-> magnitude(stix_measure_make_length(d, 0.2, roseunit_mm)); stix_tol_put_aspect(gt, callout); stix_tol_put_datums(gt, sys);
StixTolType Values
typedef unsigned long StixTolType; // geometric types with no or optional datums #define STIX_TOL_GEOMETRIC_MASK ((StixTolType) 0x7FFF) #define STIX_TOL_CYLINDRICITY ((StixTolType) 0x01) #define STIX_TOL_FLATNESS ((StixTolType) 0x02) #define STIX_TOL_LINE_PROFILE ((StixTolType) 0x04) #define STIX_TOL_POSITION ((StixTolType) 0x08) #define STIX_TOL_ROUNDNESS ((StixTolType) 0x10) #define STIX_TOL_STRAIGHTNESS ((StixTolType) 0x20) #define STIX_TOL_SURFACE_PROFILE ((StixTolType) 0x40) // geometric types with datums #define STIX_TOL_ANGULARITY ((StixTolType) 0x80) #define STIX_TOL_CIRCULAR_RUNOUT ((StixTolType) 0x100) #define STIX_TOL_COAXIALITY ((StixTolType) 0x200) #define STIX_TOL_CONCENTRICITY ((StixTolType) 0x400) #define STIX_TOL_PARALLELISM ((StixTolType) 0x800) #define STIX_TOL_PERPENDICULARITY ((StixTolType) 0x1000) #define STIX_TOL_SYMMETRY ((StixTolType) 0x2000) #define STIX_TOL_TOTAL_RUNOUT ((StixTolType) 0x4000) // other tolerance characteristics #define STIX_TOL_WITH_DATUMS ((StixTolType) 0x8000) #define STIX_TOL_WITH_MODS ((StixTolType) 0x10000) #define STIX_TOL_WITH_MAX ((StixTolType) 0x20000) #define STIX_TOL_ORIG_MODIFIER ((StixTolType) 0x40000) #define STIX_TOL_WITH_UNIT ((StixTolType) 0x80000) #define STIX_TOL_WITH_AREA_UNIT ((StixTolType) 0x100000) #define STIX_TOL_UNEQ_DISPOSED ((StixTolType) 0x200000)
The StixTolType typedef and associated values provide a simple way to describe a tolerance type and any extra parameters that may be associated with it. The values are bit flags that can be combined by addition or logical OR. Each of the values corresponds to a geometric tolerance subtype. A tolerance object is normally one of the geometric subtypes (the first two groups above) and may include one or more subtypes from the last group to add additional parameters. The additional subtypes are combined as a complex instance.
Pass these values to the stix_tol_make() function to create a new tolerance of a particular type. Call the stix_tol_type() function to find the flags for an existing tolerance.
The STIX_TOL_GEOMETRIC_MASK
can isolate the geometric
tolerance flags from the other types, which is useful for switch
statements based on the tolerance type. The
stix_tol_geometric_types()
function also does this bit masking. The EXPRESS types corresponding
to each definition are listed below.
stix_tol_add_common_datum()
stp_general_datum_reference * stix_tol_add_common_datum( stp_datum_system * sys, stp_datum * dat1, stp_datum * dat2 ); stp_general_datum_reference * stix_tol_add_common_datum( stp_geometric_tolerance * gt, stp_datum * dat1, stp_datum * dat2 );
The stix_tol_add_common_datum() function adds a pair of datums to the list of datums kept by a datum system. It returns a pointer to the reference object on success or NULL if an error occurred. If you need a common datum across three elements (A-B-C), create the common datum with the first two and then add the third with stix_tol_add_datum().
Passing in a tolerance adds the common datum to the system referenced by that tolerance, which may be shared with other tolerances. A datum system will be created and added to the tolerance if needed. The function returns null if the tolerance can not accept datums.
Use stix_tol_get_datum_ref() to find the reference object specific to each datum when applying modifiers.
stp_geometric_tolerance * gt; stp_datum * dat_a; stp_datum * dat_b; stp_datum * dat_c; // add common datum for A-B stp_general_datum_reference * comdat = stix_tol_add_common_datum(gt, dat_a, dat_b); // add a modifier to A in the common datum stix_tol_add_datum_modifier( stix_tol_get_datum_ref(comdat,dat_a), stp_simple_datum_reference_modifier_free_state ); // make the common datum A-B-C stix_tol_add_datum(comdat, dat_c);
stix_tol_add_datum()
stp_general_datum_reference * stix_tol_add_datum( stp_datum_system * sys, stp_datum * dat ); stp_general_datum_reference * stix_tol_add_datum( stp_geometric_tolerance * gt, stp_datum * dat ); stp_general_datum_reference * stix_tol_add_datum( stp_general_datum_reference * ref, stp_datum * dat );
The stix_tol_add_datum() function appends a datum to the list of datums kept by a datum system. It returns a pointer to the reference object on success or NULL if the datum system is null. The reference object can be used to apply modifiers to the datum.
Passing in a tolerance adds the datum to the system referenced by that tolerance, which may be shared with other tolerances. The stix_tol_make_datums() function will be called to create and associate a system with the tolerance if necessary. The function returns null if the tolerance can not accept datums.
You can also add datums to an existing datum reference to extend a common datum as discussed in stix_tol_add_common_datum()
RoseDesign * d; stp_shape_aspect * callout; // make a tolerance and datum stp_geometric_tolerance * gt = stix_tol_make(d, STIX_TOL_SURFACE_PROFILE + STIX_TOL_WITH_DATUMS); gt-> name(surface profile tolerance with datum); gt-> magnitude(stix_measure_make_length(d, 0.07, roseunit_mm)); stix_tol_put_aspect(gt, callout); stp_datum * d1 = stix_tol_make_datum(A, callout->of_shape()); // append the datum stp_general_datum_reference * ref = stix_tol_add_datum (gt,d1); // add a simple modifier to the datum stix_tol_add_datum_modifier( ref, stp_simple_datum_reference_modifier_maximum_material_requirement ); // Alternatively, we could just give the tolerance and datum, and the // function would find where to put the modifier stix_tol_add_datum_modifier( gt, d1, stp_simple_datum_reference_modifier_maximum_material_requirement ); // ------------------------ // This will produce the following STEP FILE DATA #10=( GEOMETRIC_TOLERANCE('surface profile tolerance with datum',$,#11,#100) GEOMETRIC_TOLERANCE_WITH_DATUM_REFERENCE((#12)) SURFACE_PROFILE_TOLERANCE() ); #11=LENGTH_MEASURE_WITH_UNIT(LENGTH_MEASURE(0.07),#200); #12=DATUM_SYSTEM('',$,#100,.F.,(#13)); #13=DATUM_REFERENCE_COMPARTMENT('',$,#100,.F.,#14, (SIMPLE_DATUM_REFERENCE_MODIFIER(.MAXIMUM_MATERIAL_REQUIREMENT.))); #14=DATUM('',$,#100,.F.,'A'); #100=PRODUCT_DEFINITION_SHAPE( /* for some product */ ); #200=( LENGTH_UNIT() NAMED_UNIT(*) SI_UNIT(.MILLI.,.METRE.) );
stix_tol_add_datum_modifier()
// Add a simple datum modifier like MMC or LMC RoseStatus stix_tol_add_datum_modifier( stp_datum_system * sys, stp_datum * dat, stp_simple_datum_reference_modifier mod ); RoseStatus stix_tol_add_datum_modifier( stp_geometric_tolerance * gt, stp_datum * dat, stp_simple_datum_reference_modifier mod ); RoseStatus stix_tol_add_datum_modifier( stp_general_datum_reference * ref, stp_simple_datum_reference_modifier mod ); // Add a datum modifier with associated length RoseStatus stix_tol_add_datum_modifier( stp_datum_system * sys, stp_datum * dat, stp_datum_reference_modifier_type mod, double length_value, RoseUnit u ); RoseStatus stix_tol_add_datum_modifier( stp_geometric_tolerance * gt, stp_datum * dat, stp_datum_reference_modifier_type mod, double length_value, RoseUnit u ); RoseStatus stix_tol_add_datum_modifier( stp_general_datum_reference * ref, stp_datum_reference_modifier_type mod, double length_value, RoseUnit u );
The stix_tol_add_datum_modifier() function adds a modifier to a datum where it is referenced by a datum system. A simple modifier like MMC or LMC can be added, or a modifier with a length value. The modifiers are described by enumerations and there are separate enums for the simple and with-value ones.
If a tolerance is supplied, the modifier will be added to the system referenced by that tolerance, which may be shared with other tolerances.
There are several overload versions of this function. You can pass the reference object or you can pass the tolerance and datum and the function will find it. There are also separate versions that take a simple modifier or a modifier with a length and unit.
stp_geometric_tolerance * gt; stp_datum * dat; // add a simple MMC modifier to the datum stix_tol_add_datum_modifier( gt, dat, stp_simple_datum_reference_modifier_maximum_material_requirement ); // Alternatively, if we had the reference object stp_general_datum_reference * ref = stix_tol_get_datum_ref(gt,dat); stix_tol_add_datum_modifier( ref, stp_simple_datum_reference_modifier_maximum_material_requirement ); // add a 0.5mm projected distance modifier to the datum stix_tol_add_datum_modifier( gt, dat, stp_datum_reference_modifier_type_projected, 0.5, roseunit_mm );
stix_tol_add_modifier()
RoseStatus stix_tol_add_modifier( stp_geometric_tolerance * gt, stp_geometric_tolerance_modifier val );
The stix_tol_add_modifier() function appends a modifier to
the list of modifier enum values kept by a tolerance. It returns zero
(ROSE_OK
) on success or a nonzero error status code if the
tolerance is null or can not accept modifiers.
Alternatively, you could use stix_tol_get_modifiers() to get the set of modifiers from a tolerance and append directly to it.
stp_geometric_tolerance * gt; if (stix_tol_add_datum(gt,stp_geometric_tolerance_modifier_free_state)) printf(Could not add modifier!\n); // // An alternative approach working directly with the set // SetOfstp_geometric_tolerance_modifier * mods = stix_tol_get_modifiers(gt); if (!mods) printf(Could not add modifier!\n); mods->add(stp_geometric_tolerance_modifier_free_state);
stix_tol_geometric_type()
StixTolType stix_tol_geometric_type( stp_geometric_tolerance * gt ); StixTolType stix_tol_geometric_type( StixTolType gt );
The stix_tol_geometric_type() function returns just the geometric portion of the StixTolType flags for a tolerance. You can use the return value in a switch statement to handle the different types geometric constraints.
This is equivalent to a logical AND of
the stix_tol_type() value
and STIX_TOL_GEOMETRIC_MASK
.
stp_geometric_tolerance * gt; switch (stix_tol_geometric_type(gt)) { case STIX_TOL_ANGULARITY: case STIX_TOL_CIRCULAR_RUNOUT: case STIX_TOL_COAXIALITY: case STIX_TOL_CONCENTRICITY: case STIX_TOL_CYLINDRICITY: case STIX_TOL_FLATNESS: case STIX_TOL_LINE_PROFILE: case STIX_TOL_PARALLELISM: case STIX_TOL_PERPENDICULARITY: case STIX_TOL_POSITION: case STIX_TOL_ROUNDNESS: case STIX_TOL_STRAIGHTNESS: case STIX_TOL_SURFACE_PROFILE: case STIX_TOL_SYMMETRY: case STIX_TOL_TOTAL_RUNOUT: printf(normal tolerance\n); break; default: printf(missing type or nonsense\n); break; }
stix_tol_get_area_type()
stp_area_unit_type stix_tol_get_area_type( stp_geometric_tolerance * gt );
The stix_tol_get_area_type() function returns the shape of
the per unit basis area of a tolerance. This value is the "area_type"
attribute of a tolerance that is an instance of
the geometric
tolerance with defined area unit type. The function
returns stp_area_unit_type_NULL
if the tolerance is null
or not of the correct type.
This value controls the meaning of the stix_tol_get_unit_size() and stix_tol_get_second_unit_size() values. If the area is rectangular, both values are used. If the area is square or circular, only the first value is used.
stp_geometric_tolerance * gt; switch (stix_tol_get_area_type(gt)) { case stp_area_unit_type_NULL: printf(Null or not an area unit tolerance\n); break; case stp_area_unit_type_circular: printf(Area Unit Type: circle\n); break; case stp_area_unit_type_rectangular: printf(Area Unit Type: rect\n); break; case stp_area_unit_type_square: printf(Area Unit Type: square\n); break; default: printf(Area Unit Type: UNKNOWN\n); break; }
stix_tol_get_aspect()
stp_shape_aspect * stix_tol_get_aspect( stp_geometric_tolerance * gt );
The stix_tol_get_aspect() function returns the shape aspect held by the toleranced shape aspect field of a geometric tolerance. The function will return null if the field holds a different type of value, such as a product definition shape or a dimension.
You can use stix_tol_get_pds() to get the product definition shape reachable from this field, either directly or through a shape aspect or dimension.
stix_tol_get_datum()
stp_datum * stix_tol_get_datum( stp_geometric_tolerance * gt, unsigned idx ); stp_datum * stix_tol_get_datum( stp_datum_system * sys, unsigned idx ); stp_datum * stix_tol_get_datum( stp_general_datum_reference * ref, unsigned idx ); stp_datum * stix_tol_get_datum( stp_general_datum_reference * ref );
The stix_tol_get_datum() function returns the datum at a given index associated with a tolerance, datum system, or common datum. It returns null if the index is out of range or if no datums are present. It will also return null if a given index refers to a common datum consisting of several individual datums.
The version without an index gets the datum value from a simple reference, or null if the reference contains nested, common datums.
Use stix_tol_get_datum_count() to find the bounds when looping over datums. See stix_tol_get_datum_modifier() for code examples that loop over all of the modifiers on a datum.
If a tolerance is supplied, the function will examine the datum system referenced by the tolerance. This function also works on older AP214 style datum references that pre-date the introduction of datum systems. When looping over data that uses this older datum_reference mechanism, the function will sort the references by the precedence field.
unsigned i,sz; stp_geometric_tolerance * gt; for (i=0, sz=stix_tol_get_datum_count(gt); i<sz; i++) { stp_datum * dat = stix_tol_get_datum(gt,i); if (!dat) { printf(common datum\n); continue; } printf(Datum #%lu (%s)\n, dat->entity_id(), (dat->identification()? dat->identification():null) ); }
stix_tol_get_datum_count()
unsigned stix_tol_get_datum_count( stp_datum_system * sys ); unsigned stix_tol_get_datum_count( stp_geometric_tolerance * gt ); unsigned stix_tol_get_datum_count( stp_general_datum_reference * ref );
The stix_tol_get_datum_count() function returns the number of datums associated with a datum system or common datum. The function returns zero if a general datum reference is a simple datum, with no nested common datums. See stix_tol_get_datum() for an example using this count to loop over all of the datums.
If a tolerance is supplied, the function will examine the datum system referenced by the tolerance. This function also works on older AP214 style datum references that pre-date the introduction of datum systems.
stix_tol_get_datum_modifier()
stp_simple_datum_reference_modifier stix_tol_get_datum_modifier( stp_datum_system * sys, stp_datum * dat, unsigned idx ); stp_simple_datum_reference_modifier stix_tol_get_datum_modifier( stp_geometric_tolerance * gt, stp_datum * dat, unsigned idx ); stp_simple_datum_reference_modifier stix_tol_get_datum_modifier( stp_general_datum_reference * ref, unsigned idx );
The stix_tol_get_datum_modifier() function returns the simple modifier at a specific index in the collection of modifiers associated with a datum. The function returns null if the modifier at the index is "with length" or if the index is otherwise out of range.
A datum can have simple modifiers and modifiers with a value, so code will iterate over stix_tol_get_datum_modifier_count() number of elements and call stix_tol_get_datum_modifier_with_value() if the simple enum is null.
The code below shows how to iterate over all of the modifiers for a datum. The function also recognizes the limit condition modifer in old ap214-style datum references and will automatically translate MMC and LMC into the corresponding simple modifier enum.
stp_geometric_tolerance * gt; stp_datum * dat; unsigned i,sz; for (i=0,sz=stix_tol_get_datum_modifier_count(gt,dat); i<sz; i++) { stp_simple_datum_reference_modifier mod = stix_tol_get_datum_modifier(gt,dat,i); switch (mod) { case stp_simple_datum_reference_modifier_any_cross_section: case stp_simple_datum_reference_modifier_any_longitudinal_section: case stp_simple_datum_reference_modifier_basic: case stp_simple_datum_reference_modifier_contacting_feature: case stp_simple_datum_reference_modifier_degree_of_freedom_constraint_u: case stp_simple_datum_reference_modifier_degree_of_freedom_constraint_v: case stp_simple_datum_reference_modifier_degree_of_freedom_constraint_w: case stp_simple_datum_reference_modifier_degree_of_freedom_constraint_x: case stp_simple_datum_reference_modifier_degree_of_freedom_constraint_y: case stp_simple_datum_reference_modifier_degree_of_freedom_constraint_z: case stp_simple_datum_reference_modifier_distance_variable: case stp_simple_datum_reference_modifier_free_state: case stp_simple_datum_reference_modifier_least_material_requirement: case stp_simple_datum_reference_modifier_line: case stp_simple_datum_reference_modifier_major_diameter: case stp_simple_datum_reference_modifier_maximum_material_requirement: case stp_simple_datum_reference_modifier_minor_diameter: case stp_simple_datum_reference_modifier_orientation: case stp_simple_datum_reference_modifier_pitch_diameter: case stp_simple_datum_reference_modifier_plane: case stp_simple_datum_reference_modifier_point: case stp_simple_datum_reference_modifier_translation: // adapt as needed to handle the different modifiers printf (%s\n, stix_tol_get_datum_modifier_name(mod)); continue; case stp_simple_datum_reference_modifier_NULL: default: break; // not a simple mod, handle below } // Get modifier with length value instead stp_datum_reference_modifier_with_value * modval = stix_tol_get_datum_modifier_with_value(gt,dat,i); if (!modval) continue; switch (modval->modifier_type()) { case stp_datum_reference_modifier_type_circular_or_cylindrical: case stp_datum_reference_modifier_type_distance: case stp_datum_reference_modifier_type_projected: case stp_datum_reference_modifier_type_spherical: // adapt as needed to handle the different modifiers printf (%s %g %s\n, stix_tol_get_datum_with_value_modifier_name(modval->modifier_type()), stix_measure_get_length(modval->modifier_value()), stix_unit_get_name(modval->modifier_value()) ); break; default: printf (unknown modifier\n); break; } }
stix_tol_get_datum_modifier_count()
unsigned stix_tol_get_datum_modifier_count( stp_datum_system * sys, stp_datum * dat ); unsigned stix_tol_get_datum_modifier_count( stp_geometric_tolerance * gt, stp_datum * dat ); unsigned stix_tol_get_datum_modifier_count( stp_general_datum_reference * ref );
The stix_tol_get_datum_modifier_count() function returns the number of modifiers associated with a datum usage. You can loop over the modifiers with the stix_tol_get_datum_modifier() function.
If a tolerance is supplied, the function will examine the datum system referenced by the tolerance. This function also works on older AP214 style datum references that pre-date the introduction of datum systems.
stix_tol_get_datum_modifier_name()
const char * stix_tol_get_datum_modifier_name ( stp_simple_datum_reference_modifier mod );
The stix_tol_get_datum_modifier_name() function returns a descriptive string for a simple datum modifier enumeration value. Use stix_tol_get_datum_modifier_value() to go from a string value to the enumeration.
stix_tol_get_datum_modifier_value()
stp_simple_datum_reference_modifier stix_tol_get_datum_modifier_value ( const char * nm );
The stix_tol_get_datum_modifier_value() function finds the
simple datum modifier enumeration value that matches a given string.
The function
returns stp_simple_datum_reference_modifier_NULL
if the
string is not recognized.
stix_tol_get_datum_modifier_with_value()
stp_datum_reference_modifier_with_value * stix_tol_get_datum_modifier_with_value( stp_datum_system * sys, stp_datum * dat, unsigned idx ); stp_datum_reference_modifier_with_value * stix_tol_get_datum_modifier_with_value( stp_geometric_tolerance * gt, stp_datum * dat, unsigned idx ); stp_datum_reference_modifier_with_value * stix_tol_get_datum_modifier_with_value( stp_general_datum_reference * ref, unsigned idx );
The stix_tol_get_datum_modifier_with_value() function returns the modifier with length value at a specific index in the collection of modifiers associated with a datum. The function returns null If the modifier at the index is not of the "with length" variety, or if the index is otherwise out of range.
Since a datum can have simple modifiers and modifiers with a value, code will typically loop over all of them and call this function only if the simple modifier function returns null. Refer to stix_tol_get_datum_modifier() for an example.
If a tolerance is supplied, the function will examine the datum system referenced by the tolerance.
stix_tol_get_datum_ref()
stp_general_datum_reference * stix_tol_get_datum_ref( stp_datum_system * sys, unsigned idx ); stp_general_datum_reference * stix_tol_get_datum_ref( stp_geometric_tolerance * gt, unsigned idx ); stp_general_datum_reference * stix_tol_get_datum_ref( stp_general_datum_reference * ref, unsigned idx ); stp_general_datum_reference * stix_tol_get_datum_ref( stp_datum_system * sys, stp_datum * dat ); stp_general_datum_reference * stix_tol_get_datum_ref( stp_geometric_tolerance * gt, stp_datum * dat ); stp_general_datum_reference * stix_tol_get_datum_ref( stp_general_datum_reference * ref, stp_datum * dat ); stp_general_datum_reference * stix_tol_get_datum_ref( stp_datum_system * sys, const char * nm ); stp_general_datum_reference * stix_tol_get_datum_ref( stp_geometric_tolerance * gt, const char * nm ); stp_general_datum_reference * stix_tol_get_datum_ref( stp_general_datum_reference * ref, const char * nm );
The stix_tol_get_datum_ref() function searches the datum system associated with datum system or a datum reference for a reference object that attaches a datum. The functions take an index for simple traversal, or can search by datum instance or value of the identification field. The string is not case sensitive. Passing in a tolerance will search the datum system returned by stix_tol_get_datums().
This function returns null if the datum is not associated with the tolerance.
The reference object also holds modifiers for the datum. In most cases, the reference object is a datum reference compartment, but may be a datum reference element when used in a common datum. The function returns the general_datum_reference supertype.
stix_tol_get_datum_set()
SetOfstp_datum_system_or_reference * stix_tol_get_datum_set( stp_geometric_tolerance * gt );
The stix_tol_get_datum_set() function returns the raw datum collection. Use stix_tol_get_datums() instead to get the datum system for a tolerance.
The function returns a null pointer if the tolerance is null or not of the correct type, otherwise the function will create and return an empty collection if needed. The set usually has one entry, a SELECT type that contains a a datum_system. The datum system has a list of compartments that contain a datum.
Data made before AP242, had one or more entries that go to a datum_reference and the order was determined by a separate priority field.
stix_tol_get_datum_with_value_modifier_name()
const char * stix_tol_get_datum_with_value_modifier_name ( stp_datum_reference_modifier_type mod );
The stix_tol_get_datum_with_value_modifier_name() function returns a descriptive string for a datum modifier enumeration value. This type of enumeration describes modifiers that have an associated length value. Use stix_tol_get_datum_with_value_modifier_value() to go from a string value to the enumeration.
stix_tol_get_datum_with_value_modifier_value()
stp_datum_reference_modifier_type stix_tol_get_datum_with_value_modifier_value ( const char * nm );
The stix_tol_get_datum_with_value_modifier_value() function
finds the "with value" datum modifier enumeration value that matches a
given string. The function
returns stp_datum_reference_modifier_type_NULL
if the
string is not recognized.
stix_tol_get_datums()
stp_datum_system * stix_tol_get_datums( stp_geometric_tolerance * gt );
The stix_tol_get_datums() function returns the datum system instance that holds datums for a tolerance. This is present only if a tolerance is an instance of the geometric tolerance with datum reference type. The function returns a null pointer if the tolerance is null, not of the correct type, or otherwise unset.
The datums are stored in the constituents list of the datum system. Each one is wrapped in a datum reference compartment that can also hold modifiers. You can nest two datum_reference_elements in a compartment to indicate a common datum.
stp_datum_system * sys = stix_tol_get_datums(gt); if (!sys) { printf(No Datums\n); return; } ListOfstp_datum_reference_compartment * dats = sys->constituents(); for (unsigned i=0; i<<dats->size(); i++) { stp_datum_reference_compartment * cpt = dats->get(i); RoseObject * obj = rose_get_nested_object(cpt->base()) if (obj->isa(ROSE_DOMAIN(stp_datum))) { stp_datum * ref = ROSE_CAST(stp_datum,obj); // single datum, look at compartment for modifiers } if (obj->isa(ROSE_DOMAIN(ListOfstp_datum_reference_element))) { ListOfstp_datum_reference_element * refs = ROSE_CAST(ListOfstp_datum_reference_element,obj); // common datum, look at each element } }
stix_tol_get_displacement()
stp_length_measure_with_unit * stix_tol_get_displacement( stp_geometric_tolerance * gt );
The stix_tol_get_displacement() function returns the length value of the "displacement" attribute if a tolerance is an instance of the unequally disposed geometric tolerance type. The function returns a null pointer if the tolerance is null, not of the correct type, or the value has not been set.
The stix_tol_has_unequal() function tests if a tolerance can have a displacement value.
// Print unequal displacement value if a tolerance has one. // This assumes that we have called stix_unit_tag() after // reading the file stp_geometric_tolerance * gt; stp_length_measure_with_unit * lmwu = stix_tol_get_displacement(gt); if (lmwu) { printf(Unequal Disposition: %g %s\n, stix_measure_get_value(lmwu), stix_unit_get_name (lmwu) ); }
stix_tol_get_limit_condition()
stp_limit_condition stix_tol_get_limit_condition( stp_geometric_tolerance * gt );
The stix_tol_get_limit_condition() function returns the deprecated value of the "modifier" attribute used by instances of the modified_geometric_tolerance type.
This parameter only appears in data sets that predate AP242. Use the stix_tol_get_modifier() function instead. It automatically detects the older data and returns the new value for MMC or LMC.
stix_tol_get_maximum_upper_tolerance()
stp_length_measure_with_unit * stix_tol_get_maximum_upper_tolerance( stp_geometric_tolerance * gt );
The stix_tol_get_maximum_upper_tolerance() function returns the value of the "maximum_upper_tolerance" attribute if a tolerance is an instance of the geometric tolerance with maximum tolerance type. The function returns a null pointer if the tolerance is null, not of the correct type, or the value has not been set.
The modifier set of a tolerance that has a max value must also include the "maximum material requirement" or "least material requirement" value.
stix_tol_get_modifier()
stp_geometric_tolerance_modifier stix_tol_get_modifier( stp_geometric_tolerance * gt, unsigned idx );
The stix_tol_get_modifier() function returns the modifier at a specific index in the collection of modifiers associated with a tolerance. The function returns null if the index is out of range.
The code below shows how to iterate over all of the modifiers for a datum. The function also recognizes the limit condition modifer in old AP214-style tolerances and will automatically translate MMC and LMC into the corresponding modifier enum.
stp_geometric_tolerance * gt; unsigned i,sz; for (i=0,sz=stix_tol_get_modifier_count(gt); i<sz; i++) { stp_geometric_tolerance_modifier mod = stix_tol_get_modifier(gt,i); switch (mod) { case stp_geometric_tolerance_modifier_NULL: case stp_geometric_tolerance_modifier_any_cross_section: case stp_geometric_tolerance_modifier_common_zone: case stp_geometric_tolerance_modifier_each_radial_element: case stp_geometric_tolerance_modifier_free_state: case stp_geometric_tolerance_modifier_least_material_requirement: case stp_geometric_tolerance_modifier_line_element: case stp_geometric_tolerance_modifier_major_diameter: case stp_geometric_tolerance_modifier_maximum_material_requirement: case stp_geometric_tolerance_modifier_minor_diameter: case stp_geometric_tolerance_modifier_not_convex: case stp_geometric_tolerance_modifier_pitch_diameter: case stp_geometric_tolerance_modifier_reciprocity_requirement: case stp_geometric_tolerance_modifier_separate_requirement: case stp_geometric_tolerance_modifier_statistical_tolerance: case stp_geometric_tolerance_modifier_tangent_plane: printf(%s, stix_tol_get_modifier_name(mod)); break; } }
stix_tol_get_modifier_count()
unsigned stix_tol_get_modifier_count( stp_geometric_tolerance * gt );
The stix_tol_get_modifier_count() function returns the number of modifiers associated with a tolerance. You can loop over the modifiers with the stix_tol_get_modifier() function.
stix_tol_get_modifier_name()
const char * stix_tol_get_modifier_name ( stp_geometric_tolerance_modifier mod );
The stix_tol_get_modifier_name() function returns a descriptive string for a tolerance modifier enumeration value. Use stix_tol_get_modifier_value() to go from a string value to the enumeration.
stix_tol_get_modifier_value()
stp_geometric_tolerance_modifier stix_tol_get_modifier_value ( const char * nm );
The stix_tol_get_modifier_value() function finds the
tolerance modifier enumeration value that matches a given string. The
function returns stp_geometric_tolerance_modifier_NULL
if the string is not recognized.
stix_tol_get_modifiers()
SetOfstp_geometric_tolerance_modifier * stix_tol_get_modifiers( stp_geometric_tolerance * gt );
The stix_tol_get_modifiers() function returns the value of the "modifiers" attribute if a tolerance is an instance of the geometric tolerance with modifiers type. The function returns a null pointer if the tolerance is null or not of the correct type, otherwise the function will create and return an empty set if needed.
The example below shows one way to iterate over the values of the set.
SetOfstp_geometric_tolerance_modifier * mods = stix_tol_get_modifiers(gt); if (!mods) { printf(No Modifiers\n); return; } for (unsigned i=0; i<<mods->size(); i++) { stp_geometric_tolerance_modifier mod = mods->get(i); switch (mod) { case stp_geometric_tolerance_modifier_NULL: case stp_geometric_tolerance_modifier_any_cross_section: case stp_geometric_tolerance_modifier_common_zone: case stp_geometric_tolerance_modifier_each_radial_element: case stp_geometric_tolerance_modifier_free_state: case stp_geometric_tolerance_modifier_least_material_requirement: case stp_geometric_tolerance_modifier_line_element: case stp_geometric_tolerance_modifier_major_diameter: case stp_geometric_tolerance_modifier_maximum_material_requirement: case stp_geometric_tolerance_modifier_minor_diameter: case stp_geometric_tolerance_modifier_not_convex: case stp_geometric_tolerance_modifier_pitch_diameter: case stp_geometric_tolerance_modifier_reciprocity_requirement: case stp_geometric_tolerance_modifier_separate_requirement: case stp_geometric_tolerance_modifier_statistical_tolerance: case stp_geometric_tolerance_modifier_tangent_plane: printf(%s, stix_tol_get_modifier_name(mod)); break; } }
stix_tol_get_pds()
stp_product_definition_shape * stix_tol_get_pds( stp_geometric_tolerance * gt );
The stix_tol_get_pds() function returns the product definition shape (PDS) from the toleranced_shape_aspect field of the tolerance. A tolerance is usually attached directly to a product through a shape aspect, but may be associated directly with a product definition shape, size or location dimension. The function will search whatever type the tolerance is applied to for the underlying PDS.
stix_tol_get_second_unit_size()
stp_length_measure_with_unit * stix_tol_get_second_unit_size( stp_geometric_tolerance * gt );
The stix_tol_get_second_unit_size() function returns the "second_unit_size" length measure if a tolerance is an instance of the geometric tolerance with defined area unit type. The function returns a null pointer if the tolerance is null, not of the correct type, or the value has not been set.
Use this with stix_tol_get_unit_size to get both dimensions of a rectangular unit basis area. This value is not used for other area types. See stix_tol_get_area_type() for the area type.
Both stix_tol_has_unit() and stix_tol_has_area_unit() will return if the tolerance is of an area unit type.
// Print area dimensions if a tolerance has one. // This assumes that we have called stix_unit_tag() after // reading the file stp_geometric_tolerance * gt; stp_length_measure_with_unit * xdim = stix_tol_get_unit_size(gt); stp_length_measure_with_unit * ydim = stix_tol_get_second_unit_size(gt); printf(Area Unit: %g X %g %s\n, stix_measure_get_value(xdim), stix_measure_get_value(ydim), stix_unit_get_name (xdim) );
stix_tol_get_unit_size()
stp_length_measure_with_unit * stix_tol_get_unit_size( stp_geometric_tolerance * gt );
The stix_tol_get_unit_size() function returns the "unit_size" length measure if a tolerance is an instance of the geometric tolerance with defined unit type. The function returns a null pointer if the tolerance is null, not of the correct type, or the value has not been set.
The stix_tol_has_unit() function will return if this value is available. See stix_tol_get_second_unit_size() for an example.
stix_tol_has_area_unit()
int stix_tol_has_area_unit(stp_geometric_tolerance * gt); int stix_tol_has_area_unit(StixTolType gt);
The stix_tol_has_area_unit() function returns true if a tolerance instance inherits from geometric tolerance with defined area unit. The function tests a stix_tol_type() value against the STIX_TOL_WITH_AREA_UNIT bit flag.
If true, stix_tol_get_second_unit_size(), stix_tol_put_second_unit_size(), stix_tol_get_area_type(), and stix_tol_put_area_type() can associate a length and area shape with the tolerance. The stix_tol_has_unit() function will also return true because area unit is a subtype of defined unit.
stix_tol_has_datums()
int stix_tol_has_datums(stp_geometric_tolerance * gt); int stix_tol_has_datums(StixTolType gt);
The stix_tol_has_datums() function returns true if a tolerance instance inherits from geometric tolerance with datum reference. The function tests a stix_tol_type() value against the STIX_TOL_WITH_DATUMS bit flag.
If true, stix_tol_get_datums() and stix_tol_put_datums() can associate a datum system with the tolerance.
stp_geometric_tolerance * gt; if (stix_tol_has_datums(gt)) printf(Tolerance can have datums\n); // can also work with the toltype directly StixTolType typ = stix_tol_type(gt); if (stix_tol_has_datums(typ)) printf(Tolerance can have datums\n); if (typ & STIX_TOL_WITH_DATUMS) printf(Tolerance can have datums\n);
stix_tol_has_max()
int stix_tol_has_max(stp_geometric_tolerance * gt); int stix_tol_has_max(StixTolType gt);
The stix_tol_has_max() function returns true if a tolerance instance inherits from geometric tolerance with maximum tolerance. The function tests a stix_tol_type() value against the STIX_TOL_WITH_MAX bit flag.
If true, stix_tol_get_maximum_upper_tolerance() and stix_tol_put_maximum_upper_tolerance() can associate an upper length with the tolerance. The stix_tol_has_modifiers() function will also return true because maximum tolerance is a subtype of tolerance with modifiers.
stix_tol_has_modifiers()
int stix_tol_has_modifiers(stp_geometric_tolerance * gt); int stix_tol_has_modifiers(StixTolType gt);
The stix_tol_has_modifiers() function returns true if a tolerance instance inherits from geometric tolerance with modifiers. The function tests a stix_tol_type() value against the STIX_TOL_WITH_MODS bit flag.
If true, stix_tol_get_modifiers(), stix_tol_add_modifier(), and stix_tol_put_modifiers() can associate modifier enum values with the tolerance.
stix_tol_has_orig_modifier()
int stix_tol_has_orig_modifier(stp_geometric_tolerance * gt); int stix_tol_has_orig_modifier(StixTolType gt);
The stix_tol_has_orig_modifier() function returns true if a tolerance instance inherits from the deprecated modified geometric tolerance type. The function tests a stix_tol_type() value against the STIX_TOL_ORIG_MODIFIER bit flag.
If true, stix_tol_get_limit_condition() and stix_tol_put_limit_condition() can associate a limit enum value with the tolerance.
stix_tol_has_unequal()
int stix_tol_has_unequal(stp_geometric_tolerance * gt); int stix_tol_has_unequal(StixTolType gt);
The stix_tol_has_unequal() function returns true if a tolerance instance inherits from the unequally disposed geometric tolerance. The function tests a stix_tol_type() value against the STIX_TOL_UNEQ_DISPOSED bit flag.
If true, stix_tol_get_displacement() and stix_tol_put_displacement() can associate a displacement length with the tolerance.
stix_tol_has_unit()
int stix_tol_has_unit(stp_geometric_tolerance * gt); int stix_tol_has_unit(StixTolType gt);
The stix_tol_has_unit() function returns true if a tolerance instance inherits from geometric tolerance with defined unit. The function tests a stix_tol_type() value against the STIX_TOL_WITH_UNIT bit flag.
If true, stix_tol_get_second_unit_size() and stix_tol_put_second_unit_size() associate a length with the tolerance.
stix_tol_make()
stp_geometric_tolerance * stix_tol_make( RoseDesign * d, StixTolType bitflag );
The stix_tol_make() function creates a new geometric tolerance instance with the correct EXPRESS subtypes for the traits requested by the StixTolType flags. The flags can be combined using simple addition or logical OR.
The function will create a complex instance, if needed, for the requested types. An EXPRESS combination with many types may not have a direct C++ class match, but the resulting instance is always usable as a stp_geometric_tolerance pointer, as shown in the examples below.
The function will set the required name attribute to an empty string. Use the stix_measure_make_length() function to create the numeric value for the magnitude field of the tolerance. All other parameters have "stix_tol_get/put" utility functions.
Associate the tolerance with a shape_aspect callout using the stix_tol_put_aspect() function, or you can manually set the "toleranced shape aspect" field if you want to associate it with one of the other allowable things.
A simple line profile tolerance is shown below:
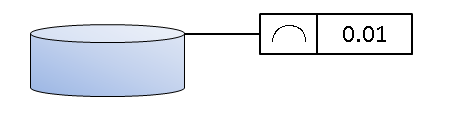
RoseDesign * d; stp_geometric_tolerance * gt; stp_shape_aspect * callout; // a 0.01mm line profile tolerance gt = stix_tol_make(d, STIX_TOL_LINE_PROFILE); gt-> name(plain line profile tolerance); gt-> magnitude(stix_measure_make_length(d, 0.01, roseunit_mm)); stix_tol_put_aspect(gt,callout); // ------------------------ // this will produce the following STEP FILE DATA #10=LINE_PROFILE_TOLERANCE('plain line profile tolerance',$,#11,#100); #11=LENGTH_MEASURE_WITH_UNIT(LENGTH_MEASURE(0.01),#40); #40=( LENGTH_UNIT() NAMED_UNIT(*) SI_UNIT(.MILLI.,.METRE.)); #100=PRODUCT_DEFINITION_SHAPE( /* for some product */ );
A slightly more complex example creates a position tolerance that can also have associated datum references.
// a 0.01mm position tolerance that can have datums gt = stix_tol_make(d, STIX_TOL_POSITION + STIX_TOL_WITH_DATUMS); gt-> name(position tolerance with datums); gt-> magnitude(stix_measure_make_length(d, 0.05, roseunit_mm)); stix_tol_put_aspect(gt,callout); // ------------------------ // this will produce the following STEP FILE DATA #20=( GEOMETRIC_TOLERANCE('position tolerance with datums',$,#21,#100) GEOMETRIC_TOLERANCE_WITH_DATUM_REFERENCE($) POSITION_TOLERANCE() ); #21=LENGTH_MEASURE_WITH_UNIT(LENGTH_MEASURE(0.05),#40);
Finally, a large example that creates a position tolerance with all possible additional parameters and initializes them to some values. Most of the values are length measures, but we also create the beginnings of a datum reference. Note that this combination may not make physical sense, but it is simply to demonstrate how to work with each of the extra parameters.
Note when creating the tolerance, "with_max" implies "with_mods",
so a separate flag for modifiers was omitted for simplicity. The area
unit and defined unit flags are a similar case. It would not cause
any problems to include the extra flags, but they are not needed.
// a 0.07mm position tolerance with everything. gt = stix_tol_make( d, STIX_TOL_SURFACE_PROFILE + STIX_TOL_WITH_DATUMS + STIX_TOL_WITH_MAX + // also a subtype of with_modifiers STIX_TOL_WITH_AREA_UNIT + // also a subtype of with_defined_unit STIX_TOL_UNEQ_DISPOSED); gt-> name(surface profile tolerance with everything); gt-> magnitude(stix_measure_make_length(d, 0.07, roseunit_mm)); stix_tol_put_aspect(gt,callout); // add a datum stp_datum * d1 = stix_tol_make_datum(A, callout->of_shape()); stix_tol_add_datum (gt,d1); // modifier and maximum stix_tol_add_modifier( gt,stp_geometric_tolerance_modifier_maximum_material_requirement ); stix_tol_put_maximum_upper_tolerance( gt, stix_measure_make_length(d, 0.1, roseunit_mm) ); // set a rectangular 1x2 per unit basis for the tolerance stix_tol_put_unit_size( gt, stix_measure_make_length(d, 1.0, roseunit_mm) ); stix_tol_put_second_unit_size( gt, stix_measure_make_length(d, 2.0, roseunit_mm) ); stix_tol_put_area_type(gt, stp_area_unit_type_rectangular); // displacement for an unequally disposed tolerance stix_tol_put_displacement( gt, stix_measure_make_length(d, 0.01, roseunit_mm) ); // ------------------------ // this will produce the following STEP FILE DATA #30=( GEOMETRIC_TOLERANCE('surface profile tolerance with everything',$,#31,#100) GEOMETRIC_TOLERANCE_WITH_DATUM_REFERENCE((#36)) GEOMETRIC_TOLERANCE_WITH_DEFINED_AREA_UNIT(.RECTANGULAR.,#32) GEOMETRIC_TOLERANCE_WITH_DEFINED_UNIT(#33) GEOMETRIC_TOLERANCE_WITH_MAXIMUM_TOLERANCE(#34) GEOMETRIC_TOLERANCE_WITH_MODIFIERS((.MAXIMUM_MATERIAL_REQUIREMENT.)) SURFACE_PROFILE_TOLERANCE() UNEQUALLY_DISPOSED_GEOMETRIC_TOLERANCE(#35) ); #31=LENGTH_MEASURE_WITH_UNIT(LENGTH_MEASURE(0.07),#40); #32=LENGTH_MEASURE_WITH_UNIT(LENGTH_MEASURE(2.0),#40); #33=LENGTH_MEASURE_WITH_UNIT(LENGTH_MEASURE(1.0),#40); #34=LENGTH_MEASURE_WITH_UNIT(LENGTH_MEASURE(0.1),#40); #35=LENGTH_MEASURE_WITH_UNIT(LENGTH_MEASURE(0.01),#40); #36=DATUM_SYSTEM('',$,#100,.F.,(#37)); #37=DATUM_REFERENCE_COMPARTMENT('',$,#100,.F.,#38,$); #38=DATUM('',$,#100,.F.,'A');
stix_tol_make_datum()
stp_datum * stix_tol_make_datum( const char * id, stp_product_definition_shape * shape );
The stix_tol_make_datum() function creates a new datum instance and associates it with the given shape property. The datum is created in the same design as the supplied shape. The function returns NULL if the shape is NULL.
stp_product_definition_shape * shape; // make a datum called "A" stp_datum * dat = stix_tol_make_datum(A, shape); // equivalent low-level code stp_datum * dat = pnewIn(shape->design()) stp_datum; dat-> identification(A); dat-> name(); // required by WR4 dat-> product_definitional(0); // required by WR3 dat-> of_shape(shape);
stix_tol_make_datum_system()
stp_datum_system * stix_tol_make_datum_system( stp_product_definition_shape * shape );
The stix_tol_make_datum_system() function creates a new datum system instance and associates it with the given shape property. The datum system is created in the same design as the supplied shape. The function returns NULL if the shape is NULL.
After you have created a datum system, you can assign datums to it with stix_tol_add_datum() and then associate it with a tolerance using stix_tol_put_datums().
stp_product_definition_shape * shape; // make a datum system and add some datums stp_datum_system * sys = stix_tol_make_datum_system(shape); stp_datum * d_a = stix_tol_make_datum(A, shape); stp_datum * d_b = stix_tol_make_datum(B, shape); stp_datum * d_c = stix_tol_make_datum(C, shape); stix_tol_add_datum(sys, d_a); stix_tol_add_datum(sys, d_b); stix_tol_add_datum(sys, d_c); // associate with a geometric tolerance // equivalent low-level create code stp_datum_system * sys = pnewIn(shape->design()) stp_datum_system; sys-> name(); sys-> product_definitional(0); // required by WR1 sys-> of_shape(shape);
stix_tol_make_datums()
stp_datum_system * stix_tol_make_datums( stp_geometric_tolerance * gt );
The stix_tol_make_datums() function is a convenience function that returns the existing datum system associated with a tolerance if one is present, or creates and associates a new datum system if one is not present. Use stix_tol_make_datum_system() instead to create single datum system and share it between several tolerances.
It will return null if the tolerance pointer is null or if the tolerance can not hold datums.
stix_tol_put_area_type()
RoseStatus stix_tol_put_area_type( stp_geometric_tolerance * gt, stp_area_unit_type val );
The stix_tol_put_area_type() function sets the value of the "area_type" attribute to an enum value. The tolerance must include the geometric tolerance with defined area unit type, which you can test using the stix_tol_has_area_unit() function. When creating a new instance, include the STIX_TOL_WITH_AREA_UNIT flag.
The function return a status value of ROSE_OK
(zero) if it
succeeds, or a nonzero error code if the tolerance pointer is null or
can not hold the parameter.
See stix_tol_put_second_unit_size() for an example and more information.
stix_tol_put_aspect()
RoseStatus stix_tol_put_aspect( stp_geometric_tolerance * gt, stp_shape_aspect * sa );
The stix_tol_put_aspect() function assigns the shape aspect held by the toleranced shape aspect field of a geometric tolerance.
This field can hold one of several different types of values. The stix_tol_put_pds() function will overwrite the value with a product definition shape.
stix_tol_put_datum_set()
RoseStatus stix_tol_put_datum_set( stp_geometric_tolerance * gt, SetOfstp_datum_system_or_reference * val );
The stix_tol_put_datum_set() function changes the raw datum collection to a new set of values. Use stix_tol_put_datums() instead to assign the datum system object associated with a tolerance.
The function return a status value of ROSE_OK
(zero) if it
succeeds, or a nonzero error code if the tolerance pointer is null or
can not hold the parameter.
See stix_tol_get_datum_set() for more discussion on the raw datum collection.
stix_tol_put_datums()
RoseStatus stix_tol_put_datums( stp_geometric_tolerance * gt, stp_datum_system * val );
The stix_tol_put_datums() function sets the datum system associated with a tolerance. The tolerance must include the geometric tolerance with datums type, which you can test using the stix_tol_has_datums() function.
When creating a new instance, some tolerances always allow datums (angularity, circular runout, coaxiality, concentricity, parallelism, perpendicularity, symmetry, or total runout), while others do only if you include the STIX_TOL_WITH_DATUMS flag. This function completely replaces the datum system.
Use stix_tol_add_datum() to add
datums incrementally. The function return a status value
of ROSE_OK
(zero) if it succeeds, or a nonzero error code if
the tolerance pointer is null or can not hold the parameter.
RoseDesign * d; stp_geometric_tolerance * gt; stp_datum_system * sys; // completely replace the existing set if (stix_tol_put_datums(gt,sys)) printf(Could not set value!!\n);
stix_tol_put_displacement()
RoseStatus stix_tol_put_displacement( stp_geometric_tolerance * gt, stp_length_measure_with_unit * mv );
The stix_tol_put_displacement() function sets the value of the "displacement" attribute to a length measure. The tolerance must include the unequally disposed geometric tolerance type, which you can test using the stix_tol_has_unequal() function. When creating a new instance, include the STIX_TOL_UNEQ_DISPOSED flag.
The function return a status value of ROSE_OK
(zero) if it
succeeds, or a nonzero error code if the tolerance pointer is null or
can not hold the parameter.
RoseDesign * d; stp_geometric_tolerance * gt; stp_length_measure_with_unit * lmwu; // make a new measure value of 123mm lmwu = stix_measure_make_length(d, 123, roseunit_mm); if (stix_tol_put_displacement(gt,lmwu)) printf(Could not set value!!\n);
stix_tol_put_limit_condition()
RoseStatus stix_tol_put_limit_condition( stp_geometric_tolerance * gt, stp_limit_condition val );
The stix_tol_put_limit_condition() function sets the deprecated "modifier" attribute to a limit condition enum value. The tolerance must include the modified geometric tolerance type, which you can test using the stix_tol_has_orig_modifier() function. You should create all new data with the the STIX_TOL_WITH_MODS option, but if you need to create older style data for some reason, the STIX_TOL_ORIG_MODIFIER flag will create it.
The function return a status value of ROSE_OK
(zero) if it
succeeds, or a nonzero error code if the tolerance pointer is null or
can not hold the parameter.
stix_tol_put_maximum_upper_tolerance()
RoseStatus stix_tol_put_maximum_upper_tolerance( stp_geometric_tolerance * gt, stp_length_measure_with_unit * mv );
The stix_tol_put_maximum_upper_tolerance() function sets the "maximum_upper_tolerance" attribute to a length measure. The tolerance must include the geometric tolerance with maximum tolerance type, which you can test using the stix_tol_has_max() function. When creating a new instance, include the STIX_TOL_WITH_MAX flag. A tolerance with a max value can have modifiers, one of which must be "maximum material requirement" or "least material requirement".
The function return a status value of ROSE_OK
(zero) if it
succeeds, or a nonzero error code if the tolerance pointer is null or
can not hold the parameter.
RoseDesign * d; stp_geometric_tolerance * gt; stp_length_measure_with_unit * lmwu; // make a new measure value of 0.5in lmwu = stix_measure_make_length(d, 0.5, roseunit_in); if (stix_tol_put_maximum_upper_tolerance(gt,lmwu)) printf(Could not set value!!\n); if (stix_tol_add_modifier(gt,stp_geometric_tolerance_modifier_maximum_material_requirement)) printf(Could not set modifier!!\n);
stix_tol_put_modifiers()
RoseStatus stix_tol_put_modifiers( stp_geometric_tolerance * gt, SetOfstp_geometric_tolerance_modifier * val );
The stix_tol_put_modifiers() function sets the value of the "modifiers" attribute to a new set of enumeration values. The tolerance must include the geometric tolerance with modifiers type, which you can test using the stix_tol_has_modifiers() function. When creating a new instance, include the STIX_TOL_WITH_MODS flag.
Typically, this function is only needed when completely replacing a set of modifiers. To add modifiers, just call stix_tol_add_modifier() or use stix_tol_get_modifiers() and add to the set.
The function return a status value of ROSE_OK
(zero) if it
succeeds, or a nonzero error code if the tolerance pointer is null or
can not hold the parameter.
RoseDesign * d; stp_geometric_tolerance * gt; SetOfstp_geometric_tolerance_modifier * mods; mods = pnewIn(d) SetOfstp_geometric_tolerance_modifier; mods->add(stp_geometric_tolerance_modifier_maximum_material_requirement); // completely replace the existing set if (stix_tol_put_modifiers(gt,mods)) printf(Could not set value!!\n);
stix_tol_put_pds()
RoseStatus stix_tol_put_pds( stp_geometric_tolerance * gt, stp_product_definition_shape * pds );
The stix_tol_put_pds() function sets the toleranced_shape_aspect field of the tolerance to hold a product definition shape. The stix_tol_put_aspect() function is more commonly used to apply the tolerance to a shape aspect.
If you want to attach a tolerance to a size or location dimension, you will need to set the "toleranced shape aspect" field manually.
stix_tol_put_second_unit_size()
RoseStatus stix_tol_put_second_unit_size( stp_geometric_tolerance * gt, stp_length_measure_with_unit * mv );
The stix_tol_put_second_unit_size() function sets the value of the "second_unit_size" attribute to a length measure. The tolerance must include the geometric tolerance with defined area unit type, which you can test using the stix_tol_has_area_unit() function. When creating a new instance, include the STIX_TOL_WITH_AREA_UNIT flag.
The function return a status value of ROSE_OK
(zero) if it
succeeds, or a nonzero error code if the tolerance pointer is null or
can not hold the parameter.
Use stix_tol_put_unit_size() to put the other dimension of the area and stix_tol_put_area_type() to describe the shape of the area.
RoseDesign * d; stp_geometric_tolerance * gt; stp_length_measure_with_unit * lmwu; // make a new measure value of 25mm lmwu = stix_measure_make_length(d, 25, roseunit_mm); // Use the same measure for both dimensions (25X25) if (stix_tol_put_unit_size(gt,lmwu)) printf(Could not set value!!\n); if (stix_tol_put_second_unit_size(gt,lmwu)) printf(Could not set value!!\n); if (!stix_tol_put_area_type(gt, stp_area_unit_type_square)) printf(Could not set area type!!\n);
stix_tol_put_unit_size()
RoseStatus stix_tol_put_unit_size( stp_geometric_tolerance * gt, stp_length_measure_with_unit * mv );
The stix_tol_put_unit_size() function sets the value of the "unit_size" attribute to a length measure. The tolerance must include the geometric tolerance with defined unit type, which you can test using the stix_tol_has_unit() function. When creating a new instance, include the STIX_TOL_WITH_UNIT flag.
The function return a status value of ROSE_OK
(zero)
if it succeeds, or a nonzero error code if the tolerance pointer is
null or can not hold the parameter.
RoseDesign * d; stp_geometric_tolerance * gt; stp_length_measure_with_unit * lmwu; // make a new measure value of 25mm lmwu = stix_measure_make_length(d, 25, roseunit_mm); if (stix_tol_put_unit_size(gt,lmwu)) printf(Could not set value!!\n);
stix_tol_remove_all_datums()
RoseStatus stix_tol_remove_all_datums( stp_datum_system * sys, );
The stix_tol_remove_all_datums() function removes all of the datum references from a datum system. The function returns ROSE_OK if any datum was removed sucessfully, or a nonzero error code if the system did not have any references.
If a tolerance is supplied, the datums will be removed from the datum system referenced by that tolerance, which may be shared with other tolerances.
stix_tol_remove_datum()
RoseStatus stix_tol_remove_datum( stp_datum_system * sys, stp_datum * dat ); RoseStatus stix_tol_remove_datum( stp_datum_system * sys, stp_general_datum_reference * ref ); RoseStatus stix_tol_remove_datum( stp_geometric_tolerance * gt, stp_datum * dat ); RoseStatus stix_tol_remove_datum( stp_geometric_tolerance * gt, stp_general_datum_reference * ref ); RoseStatus stix_tol_remove_datum( stp_general_datum_reference * ref, stp_datum * dat );
The stix_tol_remove_datum() function removes a datum reference from a datum system or common datum. The function returns ROSE_OK if the datum was removed sucessfully, or a nonzero error code if the datum was not referenced or if any of the pointers were null.
If a tolerance is supplied, the datum will be removed from the datum system referenced by that tolerance, which may be shared with other tolerances.
stix_tol_remove_datum_modifier()
RoseStatus stix_tol_remove_datum_modifier( stp_datum_system * sys, stp_datum * dat, stp_simple_datum_reference_modifier mod ); RoseStatus stix_tol_remove_datum_modifier( stp_geometric_tolerance * gt, stp_datum * dat, stp_simple_datum_reference_modifier mod ); RoseStatus stix_tol_remove_datum_modifier( stp_general_datum_reference * ref, stp_simple_datum_reference_modifier mod ); RoseStatus stix_tol_remove_datum_modifier( stp_datum_system * sys, stp_datum * dat, stp_datum_reference_modifier_type mod ); RoseStatus stix_tol_remove_datum_modifier( stp_geometric_tolerance * gt, stp_datum * dat, stp_datum_reference_modifier_type mod ); RoseStatus stix_tol_remove_datum_modifier( stp_general_datum_reference * ref, stp_datum_reference_modifier_type mod );
The stix_tol_remove_datum_modifier() function removes a modifier from a datum usage. The modifier does not need to exist on the datum usage. When removing a "with value" modifier, it will be moved to the trash along with the associated measure. The unit is not moved to the trash.
If a tolerance is supplied, the modifier will be removed from the datum system referenced by that tolerance, which may be shared with other tolerances. This function also works on older AP214 style datum references that pre-date the introduction of datum systems.
Use stix_tol_remove_datum_modifiers() to remove all of the modifiers at once.
stix_tol_remove_datum_modifiers()
RoseStatus stix_tol_remove_datum_modifiers( stp_datum_system * sys, stp_datum * dat ); RoseStatus stix_tol_remove_datum_modifiers( stp_geometric_tolerance * gt, stp_datum * dat ); RoseStatus stix_tol_remove_datum_modifiers( stp_general_datum_reference * ref );
The stix_tol_remove_datum_modifiers() function removes all modifiers from a datum usage. Any "with value" modifiers are moved to the trash along with their associated measure. The unit is not moved to the trash.
If a tolerance is supplied, the modifiers will be removed from the datum system referenced by that tolerance, which may be shared with other tolerances. This function also works on older AP214 style datum references that pre-date the introduction of datum systems.
stix_tol_remove_modifier()
RoseStatus stix_tol_remove_modifier( stp_geometric_tolerance * gt, stp_geometric_tolerance_modifier val );
The stix_tol_remove_modifier() function removes a single modifier from the set of modifiers associated with the tolerance.
stix_tol_remove_modifiers()
RoseStatus stix_tol_remove_modifiers( stp_geometric_tolerance * gt );
The stix_tol_remove_modifiers() function removes all modifiers from a tolerance. This simply empties the set of modifiers associated with the tolerance.
stix_tol_type()
StixTolType stix_tol_type(stp_geometric_tolerance * gt);
The stix_tol_type() function returns a StixTolType set of bit flags that describe the tolerance type and any extra parameters that can be associated with it. Typically a tolerance will have one geometric type (flatness, surface profile, position, etc) and may have other flags for datums, modifiers, or defined units.
The bit flags combine all of the type information in a single value that is easy to manipulate. The stix_tol_geometric_type() function strips everything but the geometric type for easy use in switch statements. There are also several "stix_tol_has" functions for testing whether a tolerance can hold certain parameters.
This function is for convenience. Alternatively, you can test for EXPRESS types using the isa() function.
StixTolType typ = stix_tol_type(gt); if (typ & STIX_TOL_WITH_DATUMS) printf(Tolerance can have datums\n); // same thing, just as an inline function if (stix_tol_has_datums(typ)) printf(Tolerance can have datums\n); // same thing, just with the EXPRESS types if (gt-> isa(ROSE_DOMAIN(stp_geometric_tolerance_with_datum_reference))) printf(Tolerance can have datums\n);