Overview
The Adaptive class is a cursor for STEP-NC process that can iterate through the process and pause at various events, such as a position change, beginning of a toolpath, end of an operation.
As with the Finder class, references to the low-level STEP-NC objects are handled as integers based on the Part 21 file IDs. The documentation for the STEP-NC Control Library describes a lower level C++ version of this API, and includes more background discussion.
The Adaptive class is available in both .NET and Node environments.
Basic Use
Create a Finder and read some data, then create a cursor and initialize with StartProject(). The cursor iterates over the STEP-NC file most recently read by Finder.
Advance through the process by calling Next() within a loop. Each call moves forward to the next process event. There are many different process events. By default, the cursor will only stop on a tool move event, but you can use SetWanted() to control the exact set of events that you will see.
At each iteration, you can examine the event, test conditions with the "GetActive" functions, and take action to emit CNC control codes. The Print Workingsteps and Print Moves programs show several events.
With a Tool Move event, use GetActiveType() to see if it is a linear move, arc, helix, etc. The GetMoveEnd() function will return position information that you can inspect with the "GetPos" and "GetArc" series of functions, such as GetPosXYZ() and GetPosDirZ().
The cursor keeps its location as a stack of process frames. The "GetActive" functions look at the top-most elements in the stack, but you can examine the whole thing with the "GetFrame" series of functions. Each stack frame holds pointers to STEP objects, a type, a status, a numeric position number for things like a workplan element index or a curve T parameter, coordinate system transforms, and a set of positions.
CtlEvent (supporting enum)
The CtlEvent enumeration identifies each of the different the process conditions (Tool move, workingstep change, etc) encountered by the cursor. These are described separately.
CtlPos (supporting type)
typedef UInt CtlPos typedef Int CtlPosType int CtlPos int CtlPosType
The CtlPos is an integer type that is a handle for position information held by the cursor. Use GetMoveEnd() to get the position handle for the end of a move, then use GetPosXYZ(), GetPosDirZ(), and related functions to get the location, tool axis, and other parameters.
The handles returned by GetMoveArc() and GetMoveProbe() are special positions held by certain types of process elements. These have their own series of access functions with the GetArc or GetProbe prefix.
The cursor knows about several other types of position, which can be addressed by CtlPosType integer constants. See GetPosType for more information. The STEP-NC Control Library also has more discussion on cursor positions.
CtlStatus (supporting enum)
The CtlStatus enumeration identifies the state of each stack frame as you advance through the process with repeated calls to Next(). This state is only useful to application code that is trying to customize the behavior of the traversal at a very detailed level. The STEP-NC Control Library has more discussion on this type.
CtlType (supporting enum)
The CtlType enumeration identifies each of the different kinds of STEP-NC elements that can appear on the process stack. Applications can use this for fine-grained decisions beyond what a CtlEvent provides, such as distinguishing between a move event that is a linear move, an arc move, or a helix move.
These are described separately.
ErrorMsg()
System::String^ ErrorMsg(); string ErrorMsg();
The ErrorMsg() function returns a string description of
an error condition. The Next() function
returns CtlEvent.ERROR
when an error has occurred.
Event()
CtlEvent Event();
The Event() function returns the result of the most recent call to Next(). This is a convienience for code that has access to the process object but not the return value of the last call.
GetActiveAngUnit()
RoseUnit GetActiveAngUnit();
The GetActiveAngUnit() function returns the angle unit in effect at the current location in the process. This comes from the top frame in the process stack. You can investigate the angle unit in effect at other points in the process stack using the GetFrameAngUnit() function.
GetActiveAux()
System::Int64 GetActiveAux( System::Int64 num ); int GetActiveAux( int num (optional, default zero) );
The GetActiveAux() function returns an auxillary object from the top of the process stack. These vary depending on the process element but reference additional related objects, such as the toolpath axis curves, workplan setup, etc. A stack frame can hold at most five objects and the num parameter gives the index into this array.
You can investigate the auxillary objects in effect at other points in the process stack using the GetFrameAux() function.
GetActiveExec()
System::Int64 GetActiveExec(); int GetActiveExec();
The GetActiveExec() function returns the executable (program structure element like workingstep, workplan, selective, or NC function) that is closest to the top of the process stack. If you are working on an operation or toolpath event, this is likely to be a workingstep and GetActiveWorkingstep() will give you the same object.
GetActiveFeature()
System::Int64 GetActiveFeature(); int GetActiveFeature();
The GetActiveFeature() function returns the feature definition associated with a workingstep, or null if the process stack does not contain a workingstep.
GetActiveLenUnit()
RoseUnit GetActiveLenUnit();
The GetActiveLenUnit() function returns the length unit in effect at the current location in the process. This comes from the top frame in the process stack. You can investigate the angle unit in effect at other points in the process stack using the GetFrameLenUnit() function.
GetActiveMfun()
System::Int64 GetActiveMfun(); int GetActiveMfun();
The GetActiveMfun() function returns the machine functions instance that governs the current position within the process. The machine functions describes coolant state and other services provided by the machine tool. An operation or toolpath can specify a machine functions instance. If on the operation, it will apply to all toolpaths. If on a toolpath, it will override any settings from the operation.
The GetMoveIsCoolant(), GetMoveIsMistCoolant(), and GetMoveIsThruCoolant() functions return the coolant settings from the active machine functions.
The current location in the process is the top of the process stack. You can investigate the machine functions instance in effect elsewhere in the process stack using the GetFrameMfun() function.
GetActiveObj()
System::Int64 GetActiveObj(); int GetActiveObj();
The GetActiveObj() function returns the STEP object associated with the current location in the process. This object is related to the type of the process element returned by the GetActiveType() function. For example, a CtlType.EXEC_WORKSTEP type means that the object was recognized as a workingstep.
The current location in the process is the top of the process stack. You can investigate the object associated with other points in the process stack using the GetFrameObj() function. Other functions, like GetActiveWorkplan(), GetActiveOperation(), or GetActiveToolpath(), search the process stack for a particular type of object.
GetActiveOperation()
System::Int64 GetActiveOperation(); int GetActiveOperation();
The GetActiveOperation() function returns the operation element if one exists on the process stack, or zero if not present. Operations are not nested, so there is at most one operation somewhere on the stack.
GetActiveParam()
double GetActiveParam();
The GetActiveParam() function returns the numeric parameter associated with the GetActiveObj() at the current location in the process. This is used with some types of object to track location along a curve, or position within a sequence. For example, when the object is a workplan, this will indicate where in the list of elements the cursor is.
This comes from the top frame in the process stack. You can investigate the parameter value in effect at other points in the process stack using the GetFrameParam() function.
GetActivePos()
CtlPos GetActivePos( CtlPosType t, CtlCsys cs );
The GetActivePos() function searches for coordinate information associated with the current location in the process. The function takes a position type and coordinate system to search for. The GetMoveEnd() and GetMoveStart() functions search for specific position types.
This comes from the top frame in the process stack. You can search for coordinate information held by other points in the process stack using the GetFramePos() function.
GetActiveProject()
System::Int64 GetActiveProject(); int GetActiveProject();
The GetActiveProject() function returns the project object if one exists on the process stack, or zero if not present. If it exists, the project will be at the bottom of the stack. If the cursor is set with StartExec() to traverse an individual element, the project will be zero.
GetActiveStatus()
CtlStatus GetActiveStatus();
The GetActiveStatus() function returns the state of the process element at the current location in the process. This state changes as the cursor advances through process elements and is primarily for internal use by the cursor.
The current location in the process is the top of the process stack. You can investigate the state of other elements in the process stack using the GetFrameStatus() function.
GetActiveTech()
System::Int64 GetActiveTech(); int GetActiveTech();
The GetActiveTech() function the technology instance that governs the current position within the process. The technology describes process parameters such as feedrate and spindle speed. An operation or toolpath can specify a technology instance. If on the operation, it will apply to all toolpaths. If on a toolpath, it will override any settings from the operation.
The GetMoveFeed() and GetMoveSpindle() functions return the feed and speed settings from the active technology. Rapid feed is signaled by a flag on individual toolpaths, which is returned by the GetMoveIsRapid() function.
The current location in the process is the top of the process stack. You can investigate the technology instance in effect elsewhere in the process stack using the GetFrameTech() function.
GetActiveTool()
System::Int64 GetActiveTool(); int GetActiveTool();
The GetActiveTool() function returns the most recent tool instance that was requested by by the process. As the cursor advances through the process, it will encounter operations that call for a tool, but it will also pass through workplans, NC functions and other constructs that do not explicitly call for a tool.
The active tool only changes when an operation explicitly requests a different one, or upon a tool change/unload nc function. The cursor can return the tool change event CtlEvent.TOOL_CHANGE when this value changes, or you can simply compare the active tool with a known value each time you process a move event.
GetActiveToolpath()
System::Int64 GetActiveToolpath(); int GetActiveToolpath();
The GetActiveToolpath() function returns the toolpath element if one exists on the process stack, or zero if not present. Toolpaths are not nested, so there is at most one toolpath somewhere on the stack.
GetActiveType()
CtlType GetActiveType();
The GetActiveType() function returns the type of the process element at the current location in the process. Combining the event and type gives more detail about the process. For example, a CtlEvent.MOVE event with a CtlType.MOVE_ARC type means that the process is describing a circular arc motion.
The current location in the process is the top of the process stack. You can investigate the type of the process elements elsewhere in the process stack using the GetFrameType() function.
GetActiveWorkingstep()
System::Int64 GetActiveWorkingstep() int GetActiveWorkingstep()
The GetActiveWorkingstep() function returns the workingstep element if one exists on the process stack, or zero if not present. Workingsteps are not nested, so there is at most one workingstep somewhere on the stack.
GetActiveWorkplan()
System::Int64 GetActiveWorkplan(); int GetActiveWorkplan();
The GetActiveWorkplan() function returns the workplan that is closest to the top of the process stack. If you are traversing a project, there should always be a main workplan.
GetActiveXform()
array<double>^ GetActiveXform(); double[16] GetActiveXform();
The GetActiveXform() function returns the coordinate system transform at the current location in the process. This includes any stacked setup transforms or workingstep toolpath placement.
The return value is an array of 16 doubles as a 4x4 transform matrix that follows GL usage. The the CAD Math documentation has a more detailed description of the transform array.
You can investigate the transform elsewhere in the process stack using the GetFrameXform() function.
GetArcAll()
object GetArcAll( CtlPos p, RoseUnit len_unit, (optional, default as-is) RoseUnit ang_unit (optional, default deg) ); returns: { Type: CtlPosType, Csys: CtlCsys, Center: double[3], Axis: double[3], Radius: double, Angle: double, Height: double, IsCW: boolean, IsFullCircle: boolean, IsOver180: boolean, LenUnit: RoseUnit, AngUnit: RoseUnit, }
The GetArcAll() function is a node-specific function that returns an object containing all values associated with an arc movement position identifier. The properties for values that are not present are set to undefined. This may be more convenient than many calls to GetArcCenter(), GetArcAxis(), GetArcRadius(), etc.
If a length or length and angle unit is given, the values will be converted as requested.
console.log (ctl.GetArcAll(p)); { Type: 2, // arc move position Csys: 0, // WCS Center: [ 0, 2, 0 ], Axis: [ 0, 0, 1 ], Radius: 2, Angle: 270, Height: undefined, IsCW: false, IsFullCircle: false, IsOver180: true, LenUnit: 5, // inch (RoseUnit.inch) AngUnit: 21 } // degree (RoseUnit.deg)
GetArcAngle()
double GetArcAngle( CtlPos p ); double GetArcAngle( CtlPos p, RoseUnit u ); double GetArcAngle( CtlPos p, RoseUnit u (optional, default degrees) );
The GetArcAngle() function returns the angle parameter of an arc or helix move. It expects the position handle returned from the GetMoveArc() function. The value is normally in degrees and ranges from 0-360. You can get the value in radians by passing RoseUnit.rad as the second parameter. See GetArcCenter() for a full example.
GetArcAxis()
System::Boolean GetArcAxis( [System::Runtime::InteropServices::Out] double %i, [System::Runtime::InteropServices::Out] double %j, [System::Runtime::InteropServices::Out] double %k, CtlPos p ); double[3] GetArcAxis( CtlPos p );
The GetArcAxis() function returns the normalized IJK components for the axis of rotation of an arc or helix move. The position parameter should be a value from the GetMoveArc() function. This value will always be present for an arc move.
This axis defines the plane that the arc turns in. The axis is defined such that the curve extends in a counter clockwise rotation from the start point to the end point. The GetArcIsCW function compares this axis with the direction of motion to determine whether the toolpath is turning clockwise or counter clockwise.
The return value should always be true. The values are copied into the three return parameters.
The values are returned as a three element array.
GetArcCenter()
System::Boolean GetArcCenter ( [System::Runtime::InteropServices::Out] double %x, [System::Runtime::InteropServices::Out] double %y, [System::Runtime::InteropServices::Out] double %z, CtlPos p ); System::Boolean GetArcCenter ( [System::Runtime::InteropServices::Out] double %x, [System::Runtime::InteropServices::Out] double %y, [System::Runtime::InteropServices::Out] double %z, CtlPos p, RoseUnit u ); double[3] GetArcCenter( CtlPos p, RoseUnit u (optional, default as-is) );
The GetArcCenter() function returns the center point associated with an arc or helix. The position parameter should be a value from the GetMoveArc() function. If a length unit is given, the coordinates will be converted to that unit. This value will always be present for an arc move.
The return value should always be true. The values are copied into the three return parameters.
The values are returned as a three element array.
The example below shows how to get values associated with an arc move. A helix move is handled the same way.
If ctl.Event = CtlEvent.MOVE AndAlso ctl.GetActiveType = CtlType.MOVE_ARC Then Dim arc As CtlPos = ctl.GetMoveArc Dim end As CtlPos = ctl.GetMoveEnd Dim a, b, c As Double ctl.GetPosXYZ(a, b, c, end) Console.WriteLine(ARC MOVE TO: {0}, {1}, {2}, a, b, c) ctl.GetArcCenter(a, b, c, arc) Console.Write(CENTER AT: {0}, {1}, {2}, a, b, c) If ctl.GetArcIsCW(arc) Then Console.Write(DIRECTION: CW) Else Console.Write(DIRECTION: CCW) End If Console.WriteLine(RADIUS: {0}, ctl.GetArcRadius(arc)) Console.WriteLine(ANGLE: {0}, ctl.GetArcAngle(arc)) If ctl.GetArcIsFullCircle(arc) Then Console.WriteLine(full circle) End If End If
GetArcHeight()
double GetArcHeight( CtlPos p ); double GetArcHeight( CtlPos p, RoseUnit u ); double GetArcHeight( CtlPos p, RoseUnit u (optional, default as-is) );
The GetArcHeight() function only applies to helical moves (CtlType.MOVE_HELIX) and returns the separation between the start and end point of the helix, measured along the tool axis. The value may be positive or negative if the helix is moving up or down with respect to the tool.
If a length unit is given, the coordinates will be converted to that unit. The position parameter should be a value from the GetMoveArc() function. See GetArcCenter() for a full example.
GetArcIsCW()
System::Boolean GetArcIsCW(CtlPos p); boolean GetArcIsCW(CtlPos p);
The GetArcIsCW() function returns true if an arc move is in the clockwise direction and false if it is in the counter clockwise direction. This is an easy test for G2 or G3 When generating G-Code. The position parameter should be a value from the GetMoveArc() function. See GetArcCenter() for a full example.
GetArcIsFullCircle()
System::Boolean GetArcIsFullCircle(CtlPos p); boolean GetArcIsFullCircle(CtlPos p);
The GetArcIsFullCircle() function returns true if an arc move is a complete 360 degree circle and false otherwise. The position parameter should be a value from the GetMoveArc() function. See GetArcCenter() for a full example.
GetArcIsOver180()
System::Boolean GetArcIsOver180(CtlPos p); boolean GetArcIsOver180(CtlPos p);
The GetArcIsOver180() function returns true if an arc move is larger than 180 degrees circle and false if it is 180 deg or less. The position parameter should be a value from the GetMoveArc() function. See GetArcCenter() for a full example.
GetArcRadius()
double GetArcRadius( CtlPos p ); double GetArcRadius( CtlPos p, RoseUnit u ); double GetArcRadius( CtlPos p, RoseUnit u (optional, default as-is) );
The GetArcRadius() function returns the radius of an arc move. If a length unit is given, the coordinates will be converted to that unit. The position parameter should be a value from the GetMoveArc() function. See GetArcCenter() for a full example.
GetDwellTime()
double GetDwellTime () double GetDwellTime (RoseUnit u)
The GetDwellTime() function only applies to dwell events (DWELL) generated by feedstop toolpaths (TP_FEEDSTOP) and returns the duration of the pause.
If an optional time unit is given, the value will be converted to that unit.
GetDwellTimeUnit()
RoseUnit GetDwellTimeUnit()
The GetDwellTimeUnit() function returns the time unit in effect for a given dwell event.
GetFrameAngUnit()
RoseUnit GetFrameAngUnit(System::Int64 stack_pos); RoseUnit GetFrameAngUnit(int stack_pos);
The GetFrameAngUnit() function returns the angle unit in effect at a given location in the process stack. Use GetActiveAngUnit to get the angle unit on the top of the stack.
GetFrameAux()
System::Int64 GetFrameAux( System::Int64 stack_pos, System::Int64 num ); int GetFrameAux( int stack_pos, int num );
The GetFrameAux() function returns an auxillary object from a given location in the process stack. These objects vary depending on the process element but reference additional related objects, such as the toolpath axis curves, workplan setup, etc. A stack frame can hold at most five objects and the "num" parameter gives the index into this array. Use GetActiveAux to get the auxillary object on the top of the stack.
GetFrameLenUnit()
RoseUnit GetFrameLenUnit(System::Int64 stack_pos); RoseUnit GetFrameLenUnit(int stack_pos);
The GetFrameLenUnit() function returns the length unit in effect at a given location in the process stack. Use GetActiveLenUnit to get the length unit on the top of the stack.
GetFrameMfun()
System::Int64 GetFrameMfun(System::Int64 stack_pos); int GetFrameMfun(int stack_pos);
The GetFrameMfun() function returns the machine functions instance that that is in effect at a given location in the process stack. Use GetActiveMfun to get the machine functions instance for the top of the stack.
GetFrameObj()
System::Int64 GetFrameObj(System::Int64 stack_pos); int GetFrameObj(int stack_pos);
The GetFrameObj() function returns the STEP object associated with a given location in the process stack. This object is related to the type of the process element returned by the GetFrameType() function. For example, a CtlType.EXEC_WORKSTEP type means that the object was recognized as a workingstep.
Use GetActiveObj to get the STEP object on the top of the stack.
GetFrameParam()
double GetFrameParam(System::Int64 stack_pos); double GetFrameParam(int stack_pos);
The GetFrameParam() function returns the numeric parameter associated with the GetFrameObj() at a given location in the process stack. This is used with some types of object to track location along a curve, or position within a sequence. For example, when the object is a workplan, this will indicate where in the list of elements the cursor is.
Use GetActiveParam to get the parameter value on the top of the stack.
GetFramePos()
CtlPos GetFramePos( System::Int64 stack_pos, CtlPosType t, CtlCsys cs );
The GetFramePos() function searches for coordinate information at a given location in the process stack. The function takes a position type and coordinate system to search for. Use GetActivePos to look for a position on the top of the stack.
GetFrameStatus()
CtlStatus GetFrameStatus(System::Int64 stack_pos); CtlStatus GetFrameStatus(int stack_pos);
The GetFrameStatus() function returns the state of the process element at a given location in the process stack. This state changes as the cursor advances through process elements and is primarily for internal use by the cursor. Use GetActiveStatus to get the status on the top of the stack.
GetFrameTech()
System::Int64 GetFrameTech(System::Int64 stack_pos); int GetFrameTech(int stack_pos);
The GetFrameTech() function returns the technology instance that that is in effect at a given location in the process stack. Use GetActiveTech to get the technology instance for the top of the stack.
GetFrameType()
CtlType GetFrameType(System::Int64 stack_pos); CtlType GetFrameType(int stack_pos);
The GetFrameType() function returns the type of the process element at a given location in the process stack. Use GetActiveType to get the type on the top of the stack.
GetFrameXform()
array<double>^ GetFrameXform(System::Int64 stack_pos); double[16] GetFrameXform(int stack_pos);
The GetFrameXform() function returns the coordinate system transform at a given location in the process stack. This includes any stacked setup transforms or workingstep toolpath placement. Use GetActiveXform to get the transform on the top of the stack.
The return value is an array of 16 doubles as a 4x4 transform matrix that follows GL usage. The the CAD Math documentation has a more detailed description of the transform array.
GetLastPos()
CtlPos GetLastPos();
The GetLastPos() function returns the endpoint of the last move event. This position value will always be in the working coordinate system (CtlCsys.WCS).
GetLastRawPos()
CtlPos GetLastRawPos();
The GetLastRawPos() function returns the endpoint of the last move event in the raw coordinate system (CtlCsys.RAW). A move will always have a WCS position, but this may return zero if the move did not have a RAW position.
GetMoveArc()
CtlPos GetMoveArc(); CtlPos GetMoveArc( CtlCsys cs ); CtlPos GetMoveArc( CtlCsys cs (optional, default WCS) );
The GetMoveArc() function searches for an "arc" position (CTL_POS_ARC) associated with a CtlEvent.MOVE event. Only CtlType.ARC and CtlType.HELIX moves have this information. Use the GetArcCenter(), GetArcAxis(), GetArcRadius(), and GetArcAngle() functions to get the numeric values for an arc. The GetArcIsCW(), GetArcIsOver180(), and GetArcIsFullCircle() flags are also useful.
The function with no arguments searches for an arc position in the working coordinate system (CtlCsys.WCS). The second version searches for a value in the given coordinate system.
GetMoveEnd()
CtlPos GetMoveEnd(); CtlPos GetMoveEnd( CtlCsys cs ); CtlPos GetMoveEnd( CtlCsys cs (optional, default WCS) );
The GetMoveEnd() function is a special version of GetActivePos() that searches for an "end" position (CTL_POS_END) associated with the current location in the process.
The function with no arguments searches for a position in the working coordinate system (CtlCsys.WCS). The second version searches for a value in the given coordinate system.
GetMoveFeed()
double GetMoveFeed(); double GetMoveFeed( RoseUnit u ); double GetMoveFeed( RoseUnit u (optional, default as-is) );
The GetMoveFeed() function returns the feed rate in effect at a the current location in the process. If an unit argument is given, the value will be converted to that unit. The feed value is controlled by the active technology instance. Use GetMoveFeedUnit() to find the native unit for the feed value.
Toolpaths may also override the feed rate with a "rapid" flag, which you can check using the GetMoveIsRapid() function.
if ctl.getMoveIsRapid() Then Console.WriteLine (RAPID) Else Console.WriteLine (FEED {0}, ctl.GetMoveFeed()) End If
GetMoveFeedUnit()
RoseUnit GetMoveFeedUnit();
The GetMoveFeedUnit() function returns the native unit for the value provided by GetMoveFeed().
GetMoveIsCoolant()
System::Boolean GetMoveIsCoolant(); boolean GetMoveIsCoolant();
The GetMoveIsCoolant() function returns true if flood coolant has been requested at the current location in the process. The coolant settings are controlled by the active machine functions instance. Mist and through spindle coolant can also be requested.
GetMoveIsMistCoolant()
System::Boolean GetMoveIsMistCoolant(); boolean GetMoveIsMistCoolant();
The GetMoveIsMistCoolant() function returns true if mist coolant has been requested at the current location in the process. See GetMoveIsCoolant() for more discussion of coolant settings.
GetMoveIsRapid()
System::Boolean GetMoveIsRapid(); boolean GetMoveIsRapid();
The GetMoveIsRapid() function returns true if rapid motion has been requested at the current location in the process. This is not controlled by the technology instance - instead, toolpaths can override the feed rate with a "rapid" flag.
GetMoveIsThruCoolant()
System::Boolean GetMoveIsThruCoolant(); boolean GetMoveIsThruCoolant();
The GetMoveIsThruCoolant() function returns true if through-spindle coolant has been requested at the current location in the process. See GetMoveIsCoolant() for more discussion of coolant settings.
GetMoveProbe()
CtlPos GetMoveProbe(); CtlPos GetMoveProbe( CtlCsys cs ); CtlPos GetMoveProbe( CtlCsys cs (optional, default WCS) );
The GetMoveProbe() function returns a "probe" position (CTL_POS_PROBE) if a probing operation (type CtlType.OP_PROBE) exists on the process stack. The operation need not be at the top of the stack, the function will search the stack for it. With no arguments, the function searches for a position in the working coordinate system (CtlCsys.WCS), although a different value can be provided.
This position contains parameters describing the start, end, and probing direction, expected value, and variable name. Use GetProbeStart(), GetProbeEnd(), GetProbeDirection(), GetProbeExpected(), and GetProbeVar() to retrieve these values.
GetMoveSpindle()
double GetMoveSpindle(); double GetMoveSpindle( RoseUnit u ); double GetMoveSpindle( RoseUnit u (optional, default as-is) );
The GetMoveSpindle() function returns the spindle speed in effect at a the current location in the process. If a unit is given, the value will be converted to that unit. The function returns ROSE_NULL_REAL if no value is defined. A value of zero indicates spindle stopped. A positive value indicates counter-clockwise rotation and a negative value indicates clockwise rotation.
The spindle speed value is controlled by the active technology instance. Use GetMoveSpindleUnit() to find the native unit for the spindle value.
GetMoveSpindleUnit()
RoseUnit GetMoveSpindleUnit();
The GetMoveSpindleUnit() function the native unit for the value provided by GetMoveSpindle().
GetMoveStart()
CtlPos GetMoveStart(); CtlPos GetMoveStart( CtlCsys cs ); CtlPos GetMoveStart( CtlCsys cs (optional, default WCS) );
The GetMoveStart() function is a special version of GetActivePos() that searches for a "start" position (CTL_POS_START) associated with the current location in the process. With no arguments, the function searches for a position in the working coordinate system (CtlCsys.WCS), although a different value can be provided.
GetPosAll()
object GetPosAll( CtlPos p, RoseUnit len_unit (optional, default as-is) ); returns: { Type: CtlPosType, Csys: CtlCsys, XYZ: double[3], XYZObj: int, DirZ: double[3], DirZObj: int, DirX: double[3], DirXObj: int, DirSnorm: double[3], DirSnormObj: int, DirMove: double[3], SpeedRatio: double, SpeedRatioObj: int, XsectParms: object, // See GetPosXsectParms() XsectObj: int, Param: double, LenUnit: RoseUnit, AngUnit: RoseUnit, }
The GetPosAll() function is a node-specific function that returns an object containing all values associated with a position identifier. The properties for values that are not present are set to undefined. This may be more convenient than many calls to GetPosXYZ(), GetPosDirZ(), GetPosDirSnorm(), etc.
If a length unit is given, the values will be converted to that unit.
console.log (ctl.GetPosAll(p)); { Type: 0, // move end position Csys: 0, // WCS XYZ: [ -2, 2, 0 ], XYZObj: 851, DirZ: undefined, DirZObj: 0, DirX: undefined, DirXObj: 0, DirSnorm: undefined, DirSnormObj: 0, DirMove: [ 0, -1, 0 ], SpeedRatio: undefined, SpeedRatioObj: 0, XsectParms: undefined, XsectParmsObj: 0, Param: 1, LenUnit: 5, // inch (RoseUnit.inch) AngUnit: 21 } // degree (RoseUnit.deg)
GetPosAngUnit()
RoseUnit GetPosAngUnit(CtlPos p);
The GetPosAngUnit() function returns the angle unit associated with the position values.
GetPosCsys()
CtlCsys GetPosCsys(CtlPos p); enum CtlCsys { WCS = 0, // apply all transforms PART, // apply wstep xforms but not setup xforms RAW // apply no transforms } var CtlCsys = { WCS: 0, /* apply all transforms */ PART: 1, /* apply wstep xforms but not setup xforms */ RAW: 2 /* apply no transforms */ };
The GetPosCsys() function returns the coordinate system associated with the position values. This value distinguishes between several version of the same point with different transforms applied.
GetPosDefaultDirX()
System::Boolean GetPosDefaultDirX( [System::Runtime::InteropServices::Out] double %i, [System::Runtime::InteropServices::Out] double %j, [System::Runtime::InteropServices::Out] double %k, CtlPos p ); double[3] GetPosDefaultDirX( CtlPos p );
The GetPosDefaultDirX() function returns the normalized IJK components of a default tool reference direction that can be used if GetPosDirX() does not find a value. This default is the same reference direction (X axis) computed when initializing a STEP axis placement with just the Z axis given by GetPosDirZ() or GetPosDefaultDirZ().
The function always returns true and the values are copied into the return parameters.
The direction is returned as a three element array.
GetPosDefaultDirZ()
System::Boolean GetPosDefaultDirZ( [System::Runtime::InteropServices::Out] double %i, [System::Runtime::InteropServices::Out] double %j, [System::Runtime::InteropServices::Out] double %k, CtlPos p ); double[3] GetPosDefaultDirZ( CtlPos p );
The GetPosDefaultDirZ() function returns the normalized IJK components of a default tool axis direction that can be used if GetPosDirZ() does not find a value. This default is computed by starting with (0,0,1) and then applying any toolpath and setup transforms that are in effect.
The function always returns true and the values are copied into the return parameters.
The direction is returned as a three element array.
GetPosDirMove()
System::Boolean GetPosDirMove( [System::Runtime::InteropServices::Out] double %i, [System::Runtime::InteropServices::Out] double %j, [System::Runtime::InteropServices::Out] double %k, CtlPos p ); double[3] GetPosDirMove( CtlPos p );
The GetPosDirMove() function returns the normalized IJK components for the direction of motion along the toolpath associated with a position identifier. This value is calculated from the toolpath.
If the value is present, the function will return true and the values are copied into the return parameters.
If the value is present, it is returned as a three element array, otherwise the function returns undefined.
GetPosDirSnorm()
System::Boolean GetPosDirSnorm( [System::Runtime::InteropServices::Out] double %i, [System::Runtime::InteropServices::Out] double %j, [System::Runtime::InteropServices::Out] double %k, CtlPos p ); double[3] GetPosDirSnorm( CtlPos p );
The GetPosDirSnorm() function returns the normalized IJK components for the surface normal direction associated with a position identifier. This points out of the workpiece material. In a toolpath, this value comes from the "surface normal" curve property.
If the value is present, the function will return true and the values are copied into the return parameters.
If the value is present, it is returned as a three element array, otherwise the function returns undefined.
GetPosDirSnormObj()
System::Int64 GetPosDirSnormObj(CtlPos p); int GetPosDirSnormObj(CtlPos p);
The GetPosDirSnormObj() function returns the STEP object, if any, that is the source of the surface normal for the given position.
GetPosDirX()
System::Boolean GetPosDirX( [System::Runtime::InteropServices::Out] double %i, [System::Runtime::InteropServices::Out] double %j, [System::Runtime::InteropServices::Out] double %k, CtlPos p ); double[3] GetPosDirX( CtlPos p );
The GetPosDirX() function returns the normalized IJK components for the tool reference direction associated with a position identifier. This points from the tool tip along the X axis, usually towards the front of a non-rotating tool. In a toolpath, this value comes from the "tool reference direction" curve.
If the value is present, the function will return true and the values are copied into the return parameters.
If the value is present, it is returned as a three element array, otherwise the function returns undefined.
GetPosDirXObj()
System::Int64 GetPosDirXObj(CtlPos p); int GetPosDirXObj(CtlPos p);
The GetPosDirXObj() function returns the STEP object, if any, that is the source of the tool reference direction for the given position.
GetPosDirZ()
System::Boolean GetPosDirZ( [System::Runtime::InteropServices::Out] double %i, [System::Runtime::InteropServices::Out] double %j, [System::Runtime::InteropServices::Out] double %k, CtlPos p ); double[3] GetPosDirZ( CtlPos p );
The GetPosDirZ() function returns the normalized IJK components for the tool axis direction associated with a position identifier. This points from the tool tip back along the tool towards the machine. In a toolpath, this value comes from the "tool axis" curve.
If the value is present, the function will return true and the values are copied into the return parameters.
If the value is present, it is returned as a three element array, otherwise the function returns undefined.
GetPosDirZObj()
System::Int64 GetPosDirZObj(CtlPos p); int GetPosDirZObj(CtlPos p);
The GetPosDirZObj() function returns the STEP object, if any, that is the source of the tool axis direction for the given position.
GetPosIsEqual()
System::Boolean GetPosIsEqual( CtlPos p1, CtlPos p2 ); boolean GetPosIsEqual( CtlPos p1, CtlPos p2 );
The GetPosIsEqual() function compares two positions to see if they are equal. This only compares the position, tool axis, and tool reference directions. This is useful for comparing the start and end positions of a linear move. The last move on one toolpaths curve normally shares the same point as the start of the next curve.
If ctl.GetActiveType() = CtlType.MOVE Then If ctl.GetPosIsEqual(ctl.GetMoveEnd(), ctl.GetLastPos()) Then '' Skip moves where start and end positions are the '' same. Happens at toolpath connections, composite '' curves, and so on. End If End If if (ctl.GetActiveType() === CtlType.MOVE) { if (ctl.GetPosIsEqual(ctl.GetMoveEnd(), ctl.GetLastPos())) { // Skip moves where start and end positions are the // same. Happens at toolpath connections, composite // curves, and so on. } }
GetPosLenUnit()
RoseUnit GetPosLenUnit(CtlPos p);
The GetPosLenUnit() function returns the length unit associated with the position values.
GetPosParam()
double GetPosParam(CtlPos p);
The GetPosParam() function returns the numeric parameter associated with the position. This normally describes the location along the most recent curve on the process stack that the point came from. The parameter value from GetActiveParam() or GetFrameParam() usually describes the parameter value at the end of a move, whereas a position might describe the start, end, or via points in the middle of a move.
GetPosSpeedRatio()
System::Boolean GetPosSpeedRatio( [System::Runtime::InteropServices::Out] double %num, CtlPos p ); double GetPosSpeedRatio( CtlPos p );
The GetPosSpeedRatio() function the speed override value if one is associated with a position identifier. This single number is a multiplier for the feedrate. A value of one indicates the programmed feed, two indicates double the base feed, etc. In a toolpath, this value comes from the "speed profile" curve property.
If the value is present, the function will return true and the values are copied into the return parameter.
If the value is present, it is returned, otherwise the function returns undefined.
GetPosSpeedRatioObj()
System::Int64 GetPosSpeedRatioObj(CtlPos p); int GetPosSpeedRatioObj(CtlPos p);
The GetPosSpeedRatioObj() function returns the STEP object, if any, that is the source of the speed profile ratio for the position.
GetPosType()
System::Int64 GetPosType(CtlPos p); int GetPosType(CtlPos p); literal long CTL_POS_END = 0; literal long CTL_POS_START = 1; literal long CTL_POS_ARC = 2; literal long CTL_POS_VIA1 = 3; literal long CTL_POS_VIA2 = 4; literal long CTL_POS_PROBE = 5; literal long CTL_POS_RETRACT = 6;
The GetPosType() function returns an integer value that identifies the role of the position. Common values are CTL_POS_END, CTL_POS_START, and CTL_POS_ARC.
GetPosXYZ()
System::Boolean GetPosXYZ( [System::Runtime::InteropServices::Out] double %x, [System::Runtime::InteropServices::Out] double %y, [System::Runtime::InteropServices::Out] double %z, CtlPos p, ); System::Boolean GetPosXYZ( [System::Runtime::InteropServices::Out] double %x, [System::Runtime::InteropServices::Out] double %y, [System::Runtime::InteropServices::Out] double %z, CtlPos p, RoseUnit u ); double[3] GetPosXYZ( CtlPos p, RoseUnit u (optional, default as-is) );
The GetPosXYZ() function returns the XYZ cartesian coordinates associated with a position identifier. If a length unit is given, the value will be converted to that unit. In a toolpath, this value comes from the "basic curve" property.
If the value is present, the function will return true and the values are copied into the return parameters.
If the value is present, it is returned as a three element array, otherwise the function returns undefined.
GetPosXYZObj()
System::Int64 GetPosXYZObj(CtlPos p); int GetPosXYZObj(CtlPos p);
The GetPosXYZObj() function returns the STEP object, if any, that is the source of the location information for the position.
GetPosXsectParms()
System::Boolean GetPosXsectParms( [System::Runtime::InteropServices::Out] double %admax, [System::Runtime::InteropServices::Out] double %rdmax, [System::Runtime::InteropServices::Out] double %xmaxofs, [System::Runtime::InteropServices::Out] double %ymaxofs, [System::Runtime::InteropServices::Out] double %csa, [System::Runtime::InteropServices::Out] double %xcgofs, [System::Runtime::InteropServices::Out] double %ycgofs, CtlPos p, RoseUnit u ); object GetPosXsectParms( CtlPos p, RoseUnit u (optional, default as-is) ); returns: { admax: double, rdmax: double, xmaxofs: double, ymaxofs: double, csa: double, xcgofs: double, ycgofs: double }
The GetPosXsectParms() function returns the set of cross section parameters associated with a position identifier. In a toolpath, this value comes from the "cross section area parameters" property.
If the value is present, the function will return true and the values are copied into the return parameters.
If the value is present, it is returned as an object containing each of the parameters, otherwise the function returns undefined.
These parameters describe a 2D profile in the plane normal to the feed direction.
- admax is the maximum axial depth of the tool contact cross section. The axial depth is measured parallel to the tool axis. For turning, this is measured parallel to the spindle axis.
- rdmax is the maximum radial depth of the tool contact cross section. The radial depth is perpendicular to both tool axis and feed direction.
- xmaxofs is the location along the X axis where the maximum radial depth measure is located. This is the radial offset of the origin (lower left corner) of tool contact cross section measured from the tool tip.
- ymaxofs is the location along the Y axis where the maximum axial depth measure is located. This is the axial offset of the origin (lower left corner) of tool contact cross section measured from the tool tip.
- csa is the total cross section area of the tool engagement.
- xcgofs is the radial offset of the center of gravity of this cross section area. It is measured from the tool tip.
- ycgofs is the axial offset of the center of gravity of this cross section area. It is measured from the tool tip.
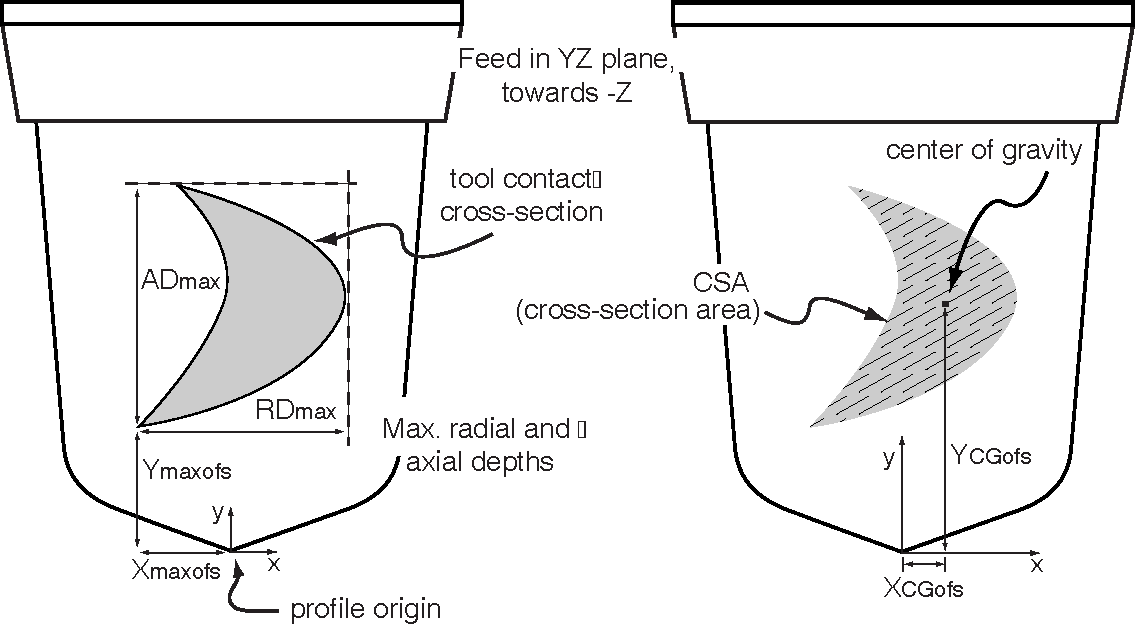
GetPosXsectObj()
System::Int64 GetPosXsectObj(CtlPos p); int GetPosXsectObj(CtlPos p);
The GetPosXsectObj() function returns the STEP object, if any that is the source of the cross section parameter information for the position.
GetProbeDirection()
System::Boolean GetProbeDirection ( [System::Runtime::InteropServices::Out] double %i, [System::Runtime::InteropServices::Out] double %j, [System::Runtime::InteropServices::Out] double %k, CtlPos p ); double[3] GetProbeDirection( CtlPos p );
The GetProbeDirection() function finds the measurement direction of a probing operation and copies the normalized IJK components into the provided array. The position parameter should be a value from the GetMoveProbe() function. The function should always return a nonzero value.
GetProbeDirectionObj()
System::Int64 GetProbeDirectionObj(CtlPos p); int GetProbeDirectionObj(CtlPos p);
The GetProbeDirectionObj() function returns the STEP direction object, if any, that is the source of the measurement direction for the probing parameters.
GetProbeEnd()
System::Boolean GetProbeEnd( [System::Runtime::InteropServices::Out] double %x, [System::Runtime::InteropServices::Out] double %y, [System::Runtime::InteropServices::Out] double %z, CtlPos p ); System::Boolean GetProbeEnd( [System::Runtime::InteropServices::Out] double %x, [System::Runtime::InteropServices::Out] double %y, [System::Runtime::InteropServices::Out] double %z, CtlPos p, RoseUnit u ); double[3] GetProbeEnd( CtlPos p, RoseUnit u (optional, default as-is) );
The GetProbeEnd() function finds the expected ending position of a probing operation and copies the XYZ coordinates into the provided array. If an optional length unit is given, the value will be converted to that unit. The position parameter should be a value from the GetMoveProbe() function. The function should always return a nonzero value.
GetProbeExpected()
double GetProbeExpected( CtlPos p ); double GetProbeExpected( CtlPos p, RoseUnit u ); double GetProbeExpected( CtlPos p, RoseUnit u (optional, default as-is) );
The GetProbeExpected() function returns the expected distance of travel for a probing operation. If an optional length unit is given, the value will be converted to that unit. The position parameter should be a value from the GetMoveProbe() function.
GetProbeExpectedObj()
System::Int64 GetProbeExpectedObj(CtlPos p); int GetProbeExpectedObj(CtlPos p);
The GetProbeExpectedObj() function returns the STEP measure object, if any, that is the source of the expected distance of travel for the probing operation parameters.
GetProbeStart()
System::Boolean GetProbeStart( [System::Runtime::InteropServices::Out] double %x, [System::Runtime::InteropServices::Out] double %y, [System::Runtime::InteropServices::Out] double %z, CtlPos p ); System::Boolean GetProbeStart( [System::Runtime::InteropServices::Out] double %x, [System::Runtime::InteropServices::Out] double %y, [System::Runtime::InteropServices::Out] double %z, CtlPos p, RoseUnit u ); double[3] GetProbeStart( CtlPos p, RoseUnit u (optional, default as-is) );
The GetProbeStart() function finds the starting position of a probing operation and copies the XYZ coordinates into the provided array. If an optional length unit is given, the value will be converted to that unit. The position parameter should be a value from the GetMoveProbe() function. The function should always return a nonzero value.
GetProbeStartObj()
System::Int64 GetProbeStartObj(CtlPos p); int GetProbeStartObj(CtlPos p);
The GetProbeStartObj() function returns the STEP axis placement object, if any, that is the source of the start position for the probing operation parameters.
GetProbeVar()
System::String^ GetProbeVar( CtlPos p ); string GetProbeVar( CtlPos p );
The GetProbeVar() function returns the string name of the STEP-NC variable associated with the probing operation. The position parameter should be a value from the GetMoveProbe() function. !-- ============================== -->
GetProbeVarObj()
System::Int64 GetProbeVarObj(CtlPos p); int GetProbeVarObj(CtlPos p);
The GetProbeVarObj() function returns the STEP expression object, if any, that is the source of the variable name in the probing operation. The position parameter should be a value from the GetMoveProbe() function.
GetProbeWorkpiece()
System::Int64 GetProbeWorkpiece(CtlPos p); int GetProbeWorkpiece(CtlPos p);
The GetProbeWorkpiece() function returns the STEP workpiece associated with the probing operation. The position parameter should be a value from the GetMoveProbe() function.
GetStackPosOfType()
System::Int64 GetStackPosOfType( CtlType t ); int GetStackPosOfType( CtlType t );
The GetStackPosOfType() function searches the stack for the most recent frame of a given type and returns the position. The function returns GetStackSize() if the value is not found.
GetStackSize()
System::Int64 GetStackSize(); int GetStackSize();
The GetStackSize() function returns the number of elements currently on the process stack. As the cursor traverses a process, it pushes these "stack frame" elements onto a stack to keep track of the location within the process.
The top of the stack contains the information relevent to the current location within the process. These values are available through the various "GetActive" functions. You can also examine the information in individual stack frames with the "GetFrame" series of functions.
The example below prints the stack of STEP objects that give the path through the process to the current location of the cursor.
GetVisitAllExecs()
System::Boolean GetVisitAllExecs(); boolean GetVisitAllExecs();
The GetVisitAllExecs() function describes how the cursor picks executables to visit on a traversal. Use SetVisitAllExecs() to change the value.
The default value is false, which indicates that the cursor examines the "enabled" flag on each executables and skips any that are marked as disabled. It will only visit one member of a selective. It will pick one if the selective has multiple enabled elements.
A value of true indicates that the cursor ignores the "enabled" flag and visits all executables that are reachable from the starting object. This includes all members of a selective.
GetWanted()
System::Boolean GetWanted(CtlEvent e); boolean GetWanted(CtlEvent e);
The GetWanted() function returns a boolean value indicating whether the cursor will stop for a particular event.
By default, cursors stop on every toolpath movement
(CtlEvent.MOVE
), but you can change this with
the SetWanted()
function. See CtlEvent for a full list of
events.
Next()
CtlEvent Next();
The Next() function advances the cursor until the next event
of interest. The return value indicates an event, or a zero value
(CtlEvent.DONE
) when finished. The return value is also
available by calling Event().
By default, the cursor will return on every toolpath movement
(CtlEvent.MOVE
), but this can be changed to return at the
start or end of every workplan, operation, toolpath, etc.
See CtlEvent for a full list of events.
the function returns CtlEvent.ERROR
if a problem is
seen. The ErrorMsg() function returns a
description of the problem.
ctl.StartProject() While ctl.Next() <> Adaptive.CtlEvent.DONE '' process the events in ctl.Event() End While ctl.StartProject(); while (ctl.Next()) { /* process the events in ctl.Event() */ }
Reset()
void Reset();
The Reset() function clears the process stack and resets the cursor. You must call StartProject() to begin another traversal.
SetVisitAllExecs()
void SetVisitAllExecs(System::Boolean yn); void SetVisitAllExecs(boolean yn);
The SetVisitAllExecs() function controls how the cursor picks executables to visit on a traversal.
The default value is false, the cursor examines the "enabled" flag on each executables and skips any that are marked as disabled. It will only visit one member of a selective. It will pick one if the selective has multiple enabled elements.
When true, the cursor ignores the "enabled" flag and visits all executables that are reachable from the starting object. This includes all members of a selective.
SetWanted()
void SetWanted( CtlEvent e ); void SetWanted( CtlEvent e, System::Boolean val ); void SetWanted( CtlEvent e boolean val (optional, default true) );
The SetWanted() function sets a boolean value indicating whether the cursor will stop for a particular event. The first parameter is the event and the second one is the boolean value, which defaults to true.
The SetWantedAll() function can turn all events on or off with a single call. See CtlEvent for a full list of events.
The following example turns some events on and off.
'' turn on all events for executables ctl.SetWanted(CtlEvent.EXEC_WORKPLAN_START) ctl.SetWanted(CtlEvent.EXEC_WORKPLAN_NEXT) ctl.SetWanted(CtlEvent.EXEC_WORKPLAN_END) ctl.SetWanted(CtlEvent.EXEC_SELECT_START) ctl.SetWanted(CtlEvent.EXEC_SELECT_END) ctl.SetWanted(CtlEvent.EXEC_WORKSTEP_START) ctl.SetWanted(CtlEvent.EXEC_WORKSTEP_END) ctl.SetWanted(CtlEvent.EXEC_OTHER_START) ctl.SetWanted(CtlEvent.EXEC_OTHER_END) ctl.SetWanted(CtlEvent.EXEC_NCFUN) // turn off notification for NC functions ctl.SetWanted(CtlEvent.EXEC_NCFUN, false)
SetWantedAll()
void SetWantedAll(System::Boolean val); void SetWantedAll(boolean val);
The SetWantedAll() function sets a boolean value for all known events indicating whether the cursor will stop for that event. Use SetWanted() to control an individual event. See CtlEvent for a full list of events.
ctl.SetWantedAll(true); '' turn all events on ctl.SetWantedAll(false); '' turn all events off
StartExec()
void StartExec( System::Int64 mpe ); void StartExec( int mpe );
The StartObject() function initializes the cursor to begin traversing the given process executable. This will overwrite any traversal that was in progress, but does not change or reset any of the events selected by SetWanted().
StartProject()
void StartProject();
The StartProject() function initializes the cursor to begin traversing the STEP-NC project in the last file opened by Finder. This will overwrite any traversal that was in progress, but does not change or reset any of the events selected by SetWanted().