Overview
The RoseMesh class describes a triangular mesh. It contains arrays of verticies, surface normals, and facets. Vertices and normals are identified by index and referenced by a facet. The topology of the mesh is reflected by sharing common vertices between facets. The RoseMeshFacet structure describes one facet.
The mesh functions are thread-safe, so they can be used while a mesh is still being built. Some functions have alternatives prefixed with an underscore (getVertex vs _getVertex) that avoid locking overhead but should only be used when a mesh is complete, or only one thread is accessing it.
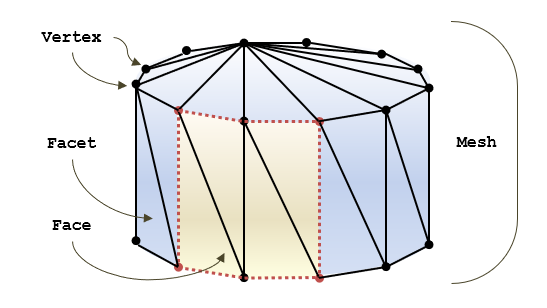
RoseMeshFacet (struct)
struct RoseMeshFacet { unsigned verts[3]; unsigned normals[3]; };
The RoseMeshFacet structure describes one triangle in a mesh. The verts member gives the three vertices of the facet and the normals member gives the surface normal at each vertex, enabling smooth shading. These are identified by a number so that they can be shared between facets. Use getVertex() to get the XYZ coordinates of a vertex and getNormal() to get the IJK components of a surface normal.
The order of verticies of the facet define the overall normal of
the facet by the right hand rule. If the facet normal is pointing
towards you, the vertices will appear in counter-clockwise order.
This is the common mathematical convention and is used when
computing getFacetNormal().
appendNullFacet()
void appendNullFacet( unsigned * idx = 0 );
The appendNullFacet() method appends a NULL facet to the mesh. This can be useful to maintain facet indicies when creating an updated version of a shell. This function is equivalent to createFacet() followed by deleteFacet().
If the optional idx parameter is set, it will be filled with the index of the NULL facet.
applyFaceBounds()
void applyFaceBounds( RoseBoundingBox& box, unsigned idx, const double * xform = 0 // double[16] ) const; void applyFaceBounds( RoseBoundingBox& box, unsigned idx, const RoseXform& xf ) const;
The applyFaceBounds() function extends a bounding box to include all of the facets in a face, possibly applying a transform to each vertex before adding it. Call clear() on the bounding box first if you want to overwrite the box with the face bounds.
applyFacetBounds()
void applyFacetBounds( RoseBoundingBox& box, unsigned idx, const double * xform = 0 // double[16] ) const; void applyFacetBounds( RoseBoundingBox& box, unsigned idx, const RoseXform& xf ) const; // Not thread safe, use with caution void _applyFacetBounds( RoseBoundingBox& box, unsigned idx, const double * xform = 0 ) const; void _applyFacetBounds( RoseBoundingBox& box, const RoseMeshFacet * f, const double * xform = 0 ) const;
The applyFacetBounds() function extends a bounding box to include the three vertices of a facet, possibly applying a transform to each coordinate before adding it. Call clear() on the bounding box first if you want to overwrite the box with the facet bounds.
applyMeshBounds()
void applyMeshBounds( RoseBoundingBox& box, const double * xform = 0 // double[16] ) const; void applyMeshBounds( RoseBoundingBox& box, const RoseXform& xf ) const;
The applyMeshBounds() function extends a bounding box to include all of the vertices in a mesh, possibly applying a transform to each coordinate before adding it. Call clear() on the bounding box first if you want to overwrite the box with the mesh bounds.
clear()
void clear();
The clear() function removes all data in a mesh. All vertex coordinates, surface normals, facets, faces, and face properties are deleted. This function is called by the destructor.
clearFaces()
void clearFaces();
The clearFaces() function removes all faces and face properties from the mesh. The facets, vertex coordinates, and surface normals are not changed.
clearFacetsOnly()
void clearFacetsOnly();
The clearFacetsOnly() method removes all facets, normals and vertices from the mesh. The faces and associated properties are not modified. This method should only be used if the facets will be recreated in the same order to maintain the indicies saved in the face descriptions.
createFace()
RoseMeshFace * createFace(); RoseMeshFace * createFace( unsigned first, unsigned count );
The createFace() function allocates a new RoseMeshFace object and optionally initializes it with facets. A face calls out a contiguous block of facets starting at first and continuing for count elements. The face object is owned by the mesh and will be deleted when the mesh is destroyed or cleared. If you do not specify the start index and count when creating the face, you can set them later with the setFirstFacet() and setFacetCount() face functions.
RoseMesh fs; // Create a face that contains facets 45,46,47 RoseMeshFace * face = fs.createFace(); face->setFirstFacet(45); face->setFacetCount(3); // same, specify all parameters when creating RoseMeshFace * face2 = fs.createFace(45, 3);
createFacet()
RoseMeshFacet * createFacet( unsigned * idx = NULL );
The createFacet() function creates a new facet in the mesh. If the idx parameter is passed in, it will be filled with the index of the newly create facet. The facet object is owned by the mesh and will be deleted when the mesh is destroyed or cleared.
createNormal()
unsigned createNormal( const double ijk[3] ); unsigned createNormal( const RoseDirection& n );
The createNormal() function creates a new normal in the facet set and initializes it with the i,j,k values. This function return the index of the newly created normal.
createVertex()
unsigned createVertex( const double xyz[3] ); unsigned createVertex( const RosePoint& pt );
The createVertex() function creates a new vertex in the facet set. This function returns the index of the newly created vertex.e
deleteFacet()
void deleteFacet( unsigned idx );
The deleteFacet() function removes the specified facet from the mesh. It simply writes null values to the vertices. The memory is not reclaimed until the mesh is cleared.
deleteNormal()
void deleteNormal( unsigned idx );
The deleteNormal() function removes the given surface normal from the mesh. It simply writes null values to the directions. The memory is not reclaimed until the mesh is cleared.
deleteVertex()
void deleteVertex( unsigned idx );
The deleteVertex() function deletes the given vertex from the mesh. It simply writes null values to the coordinates. The memory is not reclaimed until the mesh is cleared.
getAngUnit()
RoseUnit getAngUnit() const;
The getAngUnit() function is a convenience wrapper that returns the AngUnit angle unit found through getOptions(). This is usually initialized from context information in the source CAD data.
getDefaultColor()
unsigned getDefaultColor() const;
The getDefaultColor() function returns the default color
value for the mesh, typically from the source CAD data. This color is
applied if nothing more specific is found for a face or edge. This is
a 24-bit RGB encoding, of the form 0x00RRGGBB
. The
function returns ROSE_MESH_NULL_COLOR
to indicate no color
value.
getDefaultTransparent()
int getDefaultTransparent() const;
The getDefaultTransparent() function returns a code
previously set
with setDefaultTransparent() that
indicates whether the mesh should be rendered with, without, or ignore
transparency. The values
are ROSE_MESH_TRANSPARENT_IGNORE
,
ROSE_MESH_TRANSPARENT_YES
,
or ROSE_MESH_TRANSPARENT_NO
. The default is ignore.
getEdgeColor()
unsigned getEdgeColor( unsigned idx ) const;
The getEdgeColor() function returns the color value
associated with the edge at a given index. This is a 24-bit RGB
encoding, of the form 0x00RRGGBB
. The function
returns ROSE_MESH_NULL_COLOR
to indicate no color value or if
no edge information was found.
getEdgeCount()
unsigned getEdgeCount() const;
The getEdgeCount() function returns the number of edges in the mesh. Edges are only present if saved from original source data.
getEdgeInfo()
const RoseMeshEdgeInfo * getEdgeInfo( unsigned idx ) const;
The getEdgeInfo() function returns a pointer to an internal structure describing a edge curve on the mesh. Edges are only present if saved from original source data.
getEdgeObject()
virtual RoseObject * getEdgeObject ( unsigned idx ) const;
The getEdgeObject() function returns a source data object that describes a particular edge. If the mesh was created from STEP or IFC data, this will likely be a representation item. It may be null if the mesh was created from some other source or if edge information was not saved.
getEstimatedBounds()
const RoseBoundingBox & getEstimatedBounds();
The getEstimatedBounds() function returns a provisional bounding box based on the trim curves. This is available before the shell is fully rendered but may differ from the final box returned by applyMeshBounds() due to surfaces extending into the space beyond the trim curves.
getFace()
RoseMeshFace * getFace( unsigned idx ) const;
The getFace() function returns the face object at a given index. A face is an annotation, usually for traceability back to the original CAD geometry, that calls out a block of facets. A mesh may have no faces.
getFaceArea()
double getFaceArea( unsigned idx ) const;
The getFaceArea() function returns the area of the face calculated from the area of all facets that make up the face.
getFaceBuilder()
RoseMeshFaceBuilder * getFaceBuilder( unsigned idx );
The getFaceBuilder() function returns a pointer to an internal face builder object used to construct the mesh.
getFaceColor()
unsigned getFaceColor( unsigned idx ) const;
The getFaceColor() function returns the color value
associated with the face at a given index. This is a 24-bit RGB
encoding, of the form 0x00RRGGBB
.
The ROSE_MESH_NULL_COLOR
value indicates no color value. Change
this value with the setFaceColor()
function.
In STEP, IFC, or OpenGL color is often represented by floating point red, green, or blue components value from 0 to 1. Given an instance with red=1, green=.5 and blue=.25, this would be repredented as 0xff8040. The 0xff is red, the 0x80 is green, and the 0x40 is blue.
getFaceCount()
unsigned getFaceCount() const;
The getFaceCount() function returns the number of faces that are known by the mesh. See getFace() for more discussion.
getFaceDescription()
virtual RoseStringObject getFaceDescription( unsigned idx ) const;
The getFaceDescription() function returns a printable description of the face. For meshes created from STEP and IFC models, this is usually the entity id of the original instance as "#1234".
getFaceIndexFromObject()
unsigned getFaceIndexFromObject( RoseObject * obj ) const;
The getFaceIndexFromObject() function compares the given
object with the value of getObject() on each
face in the mesh. It returns returns the index of the first face that
has a matching value or ROSE_NOTFOUND
if there is no match.
getFaceInfo()
const RoseMeshFaceInfo * getFaceInfo( unsigned idx ) const;
The getFaceInfo() function returns extra mathematical surface and solver information from the original, unmeshed face surface. This also includes the edge loop information and original UV trim curves. This infomation is usually discarded after faceting to save space but can be kept by setting the RoseMeshOptions::setSaveFaceInfo() flag.
getFaceObject()
virtual RoseObject * getFaceObject ( unsigned idx ) const;
The getFaceObject() function returns a source data object that describes a particular face. If the mesh was created from STEP or IFC data, this will likely be a representation item. It may be null if the mesh was created from some other source.
getFacet()
RoseMeshFacet * getFacet( unsigned idx ); const RoseMeshFacet * getFacet( unsigned idx ) const;
The getFacet() function returns the facet object at a given index. For speed, the function does no range checking, so be sure to pass an index less than getFacetCount(). The return value may be null if the facet had been previously deleted. The const version returns a pointer to a read-only facet struct.
getFacetArea()
double getFacetArea ( unsigned idx ) const; double getFacetArea ( const RoseMeshFacet * facet ) const; // not thread safe, use with caution double _getFacetArea (unsigned idx) const;
The getFacetArea() function returns the area of a facet triangle.
getFacetCount()
unsigned getFacetCount() const; // not thread safe, use with caution unsigned _getFacetCount() const;
The getFacetCount() function returns the number of facets contained in the mesh.
getFacetNormal()
int getFacetNormal( double normal[3], unsigned f ) const; int getFacetNormal( RoseDirection& dir, unsigned f ) const; int getFacetNormal( double normal[3], const RoseMeshFacet * f ) const; int getFacetNormal( RoseDirection& dir, const RoseMeshFacet * f ) const; // not thread safe, use with caution int _getFacetNormal(double normal[3], unsigned idx) const; int _getFacetNormal(double normal[3], const RoseMeshFacet * f) const;
The getFacetNormal() function computes the surface normal of the plane holding the facet triangle. This may differ from the normals assigned to each vertex of the facet, which are used for shading in graphics display and are derived from some underlying mathematical surface. The vertex normals are available with the getFacetVertexNormal() function.
The function returns non-zero if it could successfully compute the
normal. It will return zero if the triangle is degenerate (all three
vertices are collinear) or if the facet has a null vertex. A normal
can not be calculated in these situations.
getFacetVertexNormal()
void getFacetVertexNormal( double n[3], const RoseMeshFacet * facet, unsigned vnorm_idx ) const; void getFacetVertexNormal( RoseDirection& result, const RoseMeshFacet * facet, unsigned vnorm_idx ) const; void getFacetVertexNormal( double n[3], unsigned facet_idx, unsigned vnorm_idx ) const; void getFacetVertexNormal( RoseDirection& result, unsigned facet_idx, unsigned vnorm_idx ) const;
The getFacetVertexNormal() function returns the surface normal at a given vertex of a facet, if one exists. Otherwise, it returns the normal of the facet plane, as computed by getFacetNormal().
getLenUnit()
RoseUnit getLenUnit() const;
The getLenUnit() function is a convenience wrapper that returns the LenUnit angle unit found through getOptions(). This is usually initialized from context information in the source CAD data.
getMeshArea()
double getMeshArea() const;
The getMeshArea() function returns the surface area of the mesh calculated from the area of all facets that make up the mesh. If the mesh is still under construction, it will return the area of the facets that have been computed so far.
getMeshVolume()
double getMeshVolume() const;
The getMeshVolume() function returns the volume of the mesh calculated from the facets that make up the mesh. If the mesh is still under construction, or not a closed shell, the volume is undefined.
getNormal()
void getNormal( double xyz[3], unsigned i ) const; void getNormal( RoseDirection& n, unsigned i ) const; // Not thread safe, use with caution const double * _getNormal(unsigned idx) const;
The getNormal() function returns the direction components of a surface normal. The function copy the values into an array of doubles or a direction object.
The non-thread safe version returns a pointer into the internal value buffer, which is volatile and may change if normals are added.
getNormalCount()
unsigned getNormalCount() const; // Not thread safe, use with caution unsigned _getNormalCount() const;
The getNormalCount() function returns the number of normals that are contained in the mesh. The index passed to the getNormal() function must be less than this value.
getObject()
RoseObject * getObject () const;
The getObject() function returns the source CAD object that describes the mesh. If the mesh was created from STEP or IFC data, this will likely be a representation item. It may be null if the mesh was created from some other source.
getOptions()
const RoseMeshOptions& getOptions() const; RoseMeshOptions& getOptions();
The getOptions() function returns the instance of RoseMeshOptions that describes tolerance and other settings used to create a mesh from CAD data. Each RoseMesh instance contains a separate copy of these options so a change in one mesh will not affect any other. Also, changing a value after the mesh has been created has no effect.
You can overwrite all options with the setOptions() function
// get rendering tolerance, use on const or non-const const RoseMesh * cfs; double tol = cfs->getOptions().getToleranceAbsolute(); // change rendering tolerance, only on non-const mesh ptr RoseMesh * fs; fs->getOptions().setToleranceAbsolute(0.01);
getOrigin()
typedef unsigned RoseMeshOrigin; #define ROSE_MESH_ORIGIN_EFFECTIVE 0 /* find first computed, body, other */ #define ROSE_MESH_ORIGIN_BODY 1 #define ROSE_MESH_ORIGIN_COMPUTED 2 #define ROSE_MESH_ORIGIN_MAXBUILTIN 255 RoseMeshOrigin getOrigin() const;
The getOrigin() function returns an integer value that
indicates where this mesh came from. This is used to distinguish
between multiple meshes that may be associated with a particular data
object. Several values are pre-defined and used by the meshing
software. Applications can use their own values larger
than MAXBUILTIN
.
The BODY
value indicates a mesh built from a STEP or
IFC geometry representation item, usually by faceting. Meshes have a
default origin of BODY
.
A COMPUTED
value indicates a mesh that was constructed
from inputs beyond a geometry item. In STEP, this may be the
BODY
mesh with parametric features removed, or in IFC it
may be the result of applying "voids" relations to a base shape.
The EFFECTIVE
value signals that calls
to rose_mesh_cache_find()
should return the first COMPUTED
mesh, BODY
mesh, or any other, in that order of precedence.
getProps()
const RosePropertyList * getProps( RosePropertyType t ) const; RosePropertyList * getProps( RosePropertyType t );
The getProps() function returns the list of properties attached to a mesh. You can search, add, or remove properties using separate functions. Each property declares a static find() function that returns an instance cast to the proper type, as well as a similar make() function that creates property if one is not present.
RoseMesh * fs; // return if present, null otherwise FooProp * p = FooProp::find(fs->getProps()); // return if present, create and return otherwise FooProp * p = FooProp::make(fs->getProps()); // Using functions on the property list and manually casting FooProp * p = (FooProp*) fs->getProps()->find(FooProp::type()); if (!p) { p = new FooProp; fs->getProps()->add(p); }
getRepObject()
RoseObject * getRepObject() const;
The getRepObject() function returns the source CAD object that contains and provides context for the mesh. If the mesh was created from STEP or IFC data, this will likely be a representation. It may be null if the mesh was created from some other source.
getTolerance()
double getTolerance() const;
The getTolerance() function is a convenience wrapper that returns the ToleranceAbsolute rendering tolerance found through getOptions(). This is the distance that the mesh is allowed to vary from the original mathematical surface..
getVertex()
void getVertex( double xyz[3], unsigned i ) const; void getVertex( RosePoint& pt, unsigned i ) const; // Not thread safe, use with caution const double * _getVertex(unsigned idx) const;
The getVertex() function copies the coordinates of a vertex of the mesh into an array of doubles or a point object.
The non-thread safe version returns a pointer into the internal value buffer, which is volatile and may change if vertices are added.
getVertexCount()
unsigned getVertexCount() const; // Not thread safe, use with caution unsigned _getVertexCount() const;
The getVertexCount() function returns the number of vertices that are contained in the mesh. The index passed to the getVertex() function must be less than this value.
isComplete()
int isComplete();
The isComplete() function returns true (nonzero) if all rendering jobs associated with the mesh have completed running. Typically, an advanced brep CAD solid will have a job for each face, but there may be other jobs for a sweep or CSG boolean. This can be used to test if a mesh is fully rendered before performing some operation with it.
When the mesh is complete, you can find more detailed status information by checking the result codes of the jobs as illustrated below:
RoseMesh * mesh; if (mesh->isComplete()) { // done, were there any problems building it? unsigned i,sz; for (i=0,sz=mesh->getJobCount(); i<sz; i++) { RoseMeshJob * job = mesh->getJob(i); // zero if job not done or cancelled // positive value indicates success // negative value indicates problems if (job->getResult() < 0) { // job had problems } } }
isOpen()
int isOpen() const;
The isOpen() function returns true if the mesh is not considered a watertight shell. Such meshes may have come from a non-manifold source or have missing faces. Graphics code may wish to shade both sides when drawing such meshes. This flag can be changed with the setIsOpen() function.
isTrimVisible()
int isTrimVisible() const;
The isTrimVisible() function returns a flag indicating a preference for whether display software should draw the edge curves on faces for this mesh, or just draw the shaded surfaces. This can be adjusted with the setIsTrimVisible() function.
lock() / unlock()
void lock(); void unlock();
The lock() function starts a critical section and unlock() ends it. There may be worker threads in the background, so it is important that all access to the mesh data be properly synchronized. Most of the data access functions in this class are synchronized, but you must manually synchronize if you access mesh data from a RoseMesh when the shell is not fully faceted.
There must be a call to unlock() for every call to lock, otherwise you may deadlock the mesher.
makeEdgeCurve()
virtual int makeEdgeCurve( RoseCurve & curve, unsigned idx, int full_loop ) = 0;
The makeEdgeCurve() function is a pure virtual function intended for subclasses to implement edge curve linearization algorithms specific to different kinds of mathematical representations and source data models.
makeFaceSurface()
virtual int makeFaceSurface( RoseSurface *& surf, int & sense, int & orientation, RoseMeshFaceBuilder * face )=0;
The makeFaceSurface() function is a pure virtual function intended for subclasses to implement meshing algorithms specific to different kinds of mathematical representations and source data models.
modifyNormal()
void modifyNormal( const double n[3], unsigned id ); void modifyNormal( const RoseDirection& n, unsigned id );
The modifyNormal() function changes the i,j,k components for an existing surface normal in the mesh. This changes the values in-place, so any facets that use the normal will be affected.
modifyVertex()
void modifyVertex( const double xyz[3], unsigned id ); void modifyVertex( const RosePoint& pt, unsigned id );
The modifyVertex() function changes the x,y,z coordinates for an existing vertex in the mesh. This changes the values in-place, so any facets that use the vertex will be affected.
setDefaultColor()
void setDefaultColor( unsigned rgb );
The setDefaultColor() function changes the default color
value for the mesh, which will be applied if nothing more specific is
found for a face or edge. This is a 24-bit RGB encoding, of the
form 0x00RRGGBB
. The ROSE_MESH_NULL_COLOR
value indicates no color value.
setDefaultTransparent()
void setDefaultTransparent(unsigned t); #define ROSE_MESH_TRANSPARENT_IGNORE 0 #define ROSE_MESH_TRANSPARENT_YES 1 #define ROSE_MESH_TRANSPARENT_NO 2
The setDefaultTransparent() function sets a flag indicating whether the mesh should be rendered with transparency, without, or should ignore the setting (the default).
setEdgeColor()
void setEdgeColor( unsigned rgb, unsigned idx );
The setEdgeColor() function changes the color value
associated with the edge at a given index. This is a 24-bit RGB
encoding, of the form 0x00RRGGBB
. This call is ignored
if no edge information is
present. The ROSE_MESH_NULL_COLOR
value indicates no
color value.
setFaceColor()
void setFaceColor( unsigned value, unsigned idx );
The setFaceColor() function changes the color value
associated with the face at a given index. This is a 24-bit RGB
encoding, of the form 0x00RRGGBB
.
The ROSE_MESH_NULL_COLOR
value indicates no color value.
In STEP, IFC, or OpenGL color is often represented by floating point red, green, or blue components value from 0 to 1. Given an instance with red=1, green=.5 and blue=.25, this would be repredented as 0xff8040. The 0xff is red, the 0x80 is green, and the 0x40 is blue.
setIsOpen()
void setIsOpen(int yn);
The setIsOpen() function changes the value of the isOpen() flag. This flag has no affect on the geometry or facets held by a mesh. It is simply an advisory note to display or other software that this mesh may not be watertight.
setIsTrimVisible()
void setIsTrimVisible(int yn);
The setIsTrimVisible() function changes the value of the isTrimVisible() flag. This flag is simply an advisory note to display software that edges for this mesh should not be drawn.
setObject()
void setObject ( RoseObject * obj );
The setObject() function sets the source CAD object for the mesh. For STEP or IFC geometry, this is usually a representation item. This value is used internally when building the mesh, but afterwards is just for traceability back to the source data. The getFaceObject() function returns similar links back to the face source data.
setOptions()
void setOptions( RoseMeshOptions &opts );
The setOptions() function copies an entire RoseMeshOptions object into the RoseMesh instance, overwriting any previous values. Each mesh has its own copy of the options, preventing a change in one mesh from affecting any others. Also, changing the options after the mesh has been created has no effect.
Individual options can also be changed. See getOptions() for more discussion.
setOrigin()
void setOrigin(RoseMeshOrigin o);
The setOrigin() function sets the origin value used to distinguish between different meshes associated with an object. See getOrigin() for more discussion.
setRepObject()
void setRepObject ( RoseObject * obj );
The setRepObject() function sets the source CAD object that contains and provides context for the mesh. For STEP or IFC data, this is usually a representation. This value is used internally when building the mesh, but afterwards is just for traceability back to the origin.