Overview
Status messages, errors, and warnings issued by the ROSE library are handled by the RoseErrorReporter class. By default, these are printed to the console, but you can provide your own custom code. or use the ST-Developer Message Window to view messages in a separate dialog.
There is only one reporter object, given by the ROSE.error_reporter() function.Each message is described by a RoseError struct, with a severity, numeric code, and descriptive text. The RoseErrorContext class holds thresholds that control the display of messages, and can also hold a table of RoseError messages for lookup by numeric code. The rose_ec() context holds predefined errors for ROSE library functions, and the rose_io_ec() context holds predefined errors for STEP file parsing.
Messages are normally printed as they occur, but the RoseErrorStats class can save counts and other information for summary display. Applications can also use the RoseErrorTrace class to give additional trace information like file names or line numbers.
Turning All Messages On/Off
The ROSE library prints informational messages as it reads and writes files, loads schemas, and matches EXPRESS types to C++ types. For example, the following banner is printed whenever ROSE library is first initialized:
ST-DEVELOPER System Release v20 Copyright (c) 1991-2025 by STEP Tools Inc. All Rights Reserved
Each message has a RoseStatus severity value. Informational messages have the lowest severity (ROSE_OK = 0) while warnings and errors have progressively severities. You can turn off informational messages by setting the report_threshold() to a higher value or by using the ROSE.quiet() shortcut. For example:
// No info messages, only print warnings and errors ROSE.error_reporter-> report_threshold (ROSE_STATUS_WARNING); ROSE.quiet(1); // does the same thing // Show everything, including info messages ROSE.error_reporter-> report_threshold (ROSE_OK); ROSE.quiet(0); // does the same thing
Messages are normally printed to to stdout, but you can use RoseErrorReporter::error_file() to send messages to a different file handle. If you want greater control, you can provide a RoseErrorReporter::report_fn() hook function to display the message . The following example formats a message and displays it in a Windows dialog box.
void report_in_a_box( RoseErrorReporter * rpt, RoseErrorContext * cx, const RoseError * err, va_list ap ) { RoseStringObject msg; rose_error_format_sprintf (msg, rpt, cx, err, ap); CWnd * cw = AfxGetMainWnd(); HWND mw = NULL; if (cw) mw = cw->m_hWnd; MessageBox (mw, msg.as_const(), "WARNING", MB_ICONSTOP); } // set the hook function ROSE.error_reporter()-> report_fn (report_in_a_box);
Turning Individual Messages On/Off
You can turn the reporting of individual messages off and on by calling the RoseErrorContext disable() and enable() functions and passing in one of the predefined error codes.
For general errors, call the functions on the rose_ec() context.
// avoid the warning about typecasting failures rose_ec()->disable(ROSE_EC_BAD_OBJ_CAST); [ ... your code here ... ] rose_ec()->enable(ROSE_EC_BAD_OBJ_CAST);
For file I/O errors, call the functions on the rose_io_ec() context.
// avoid the warning about extra fields rose_io_ec()->disable(ROSE_IO_FIELD_COUNT); [ ... your code here ... ] rose_io_ec()->enable(ROSE_IO_FIELD_COUNT);
Reporting Messages
An application can define its own messages using an RoseErrorContext or use the ad-hoc message(), warning(), error(), and fatal() functions as shown below. A trailing newline is added automatically by the functions.
You can also give the error reporter a RoseErrorTrace object to add trace information like file names or line numbers to your messages.
ROSE.warning (Could not open file %s., filename); rose_ec()-> warning (Could not open file %s., filename); ROSE.error (Out of memory!); rose_ec()-> error (Out of memory!); ROSE.report (ROSE_EC_NO_DESIGN, designname); rose_ec()-> report (ROSE_EC_NO_DESIGN, designname);
The RoseErrorReporter::status() function tracks the maximum severity of all issues reported. You can also give the error reporter a RoseErrorStats object to collect fine-grained information about the errors and warnings that are seen.
ST-Developer Message Window
ST-Developer for Windows contains the rose_logwin extension to display messages from GUI applications using a window to hold a cumulative messages log. The messages can be cleared, copied, and saved, and the window can be dismissed when not needed.
The the rose_logwin extension provides its own Windows message pump in a dedicated thread, and can be used by any type of ROSE C++ application: MFC, raw Windows API, or even a console application. The screendump below shows the message window and some diagnostic messages from a sample application.
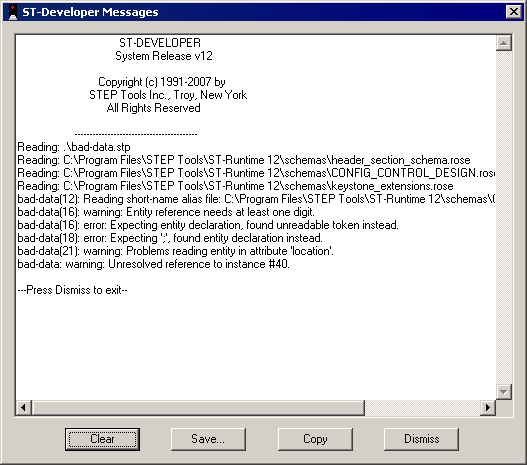
To use this, link against the rose_logwin.lib library, include the rose_logwin.h file, then call rose_logwin_init() at the start of your program to register the message window with the ROSE library.
After this call, all ROSE messages are redirected to the window. No other changes are required. Calling rose_logwin_wait() at the end of your program will block until the user dismisses the message box. This gives the user a chance to view the messages, particularly in short-lived programs.
The following example shows how to initialize the message window
#include <rose.h> #include <rose_logwin.h> int main (int argc, char ** argv) { rose_logwin_init(); /* your code */ rose_logwin_wait(); }
The roselog DLL must be in your path for the log window to appear. It is installed in the ST-Developer bin directory, which should be in your path. If the DLL cannot be found, rose_logwin_init() will return zero and use the default error handling.
General Errors
These are the issues and messages reported during general ROSE library operations. The RoseError structures for these are kept by the rose_ec() context. The preprocessor defines for these codes are prefixed with ROSE_EC and are defined in rose_errors.h.
Error Code | Severity | Message String | Comments |
---|---|---|---|
ROSE_EC_ACTIVATE_IN_READ | error | activate() called while design being read. | |
ROSE_EC_BAD_ALIAS | warning | Cannot find longname '%s' in schema %s. | |
ROSE_EC_BAD_ALIASFILE | warning | Unable to open short name file: %s. | |
ROSE_EC_BAD_ALIAS_SYNTAX | warning | Short name syntax error %s:%d. | |
ROSE_EC_BAD_ATT | warning | Attribute %s.%s not found. | |
ROSE_EC_BAD_ATT_DOM | warning | Attribute %s.%s not in domain %s. | |
ROSE_EC_BAD_BINCHAR | warning | Bad hex character '%c' for binary value. Using '0'. | |
ROSE_EC_BAD_BINSTART | warning | Bad first character '%c' for binary value. Expecting 0-3. | |
ROSE_EC_BAD_CLASS | warning | Class %s not found. | |
ROSE_EC_BAD_DOMAIN | warning | Domain %s not found. | |
ROSE_EC_BAD_HASH | error | Could not find hash insertion point. | |
ROSE_EC_BAD_NODETYPE | error | Unknown RoseNodeType %d found. | |
ROSE_EC_BAD_OBJ_CAST | warning | Cannot typecast %s object to %s | |
ROSE_EC_BAD_SCHEMA | warning | Schema %s not found. | |
ROSE_EC_BEST_FIT | info | %s.%s: Best-fit class is "%s". | |
ROSE_EC_BEST_FIT_MANY | info | %s.%s: Several possible best-fit classes. | |
ROSE_EC_BEST_FIT_NODETYPE | warning | %s.%s: Will best-fit, I/O type mismatch. | |
ROSE_EC_BEST_FIT_NONE | warning | %s.%s: No best-fit class available. | |
ROSE_EC_BOUNDS_VIOLATION | error | Bounds violation ... index(%d) >= size(%d) | |
ROSE_EC_COPY_DSTBAD | warning | copy() destination not a supertype of source | |
ROSE_EC_DICT_BAD_LISTS | error | Dictionary lists are not the same size | |
ROSE_EC_DICT_BAD_VALUE | warning | Value is not allowed in dictionary | |
ROSE_EC_DOMAIN_ACTIVE | error | Domain '%s' is active. | |
ROSE_EC_DOMAIN_INACTIVE | error | Domain '%s' is not active. | |
ROSE_EC_DOMAIN_INITFAIL | error | Failed to initialize domain '%s'. | |
ROSE_EC_DOMAIN_PRIM | error | Domain '%s' is a primitive type. | |
ROSE_EC_EXTRA_OBJECTS | warning | Design contains extra '%s' objects. | |
ROSE_EC_FOREIGN_SECTION | warning | %s: Section is from a different design, ignored. | |
ROSE_EC_FREAKY_ENUM | error | Found enum of size (%d). Expected: %d %d or %d | |
ROSE_EC_FREAKY_PRIM | error | Found primitive value of size (%d). Expected: %d %d or %d | |
ROSE_EC_GEN_DOMAIN | warning | Generating domain %s - %s. | |
ROSE_EC_GEN_DUP_SCHEMA | warning | Generating schema - ignoring schema '%s' is in search path. | |
ROSE_EC_GEN_NULL_SCHEMA | warning | Generating schema - schema name must not be null. | |
ROSE_EC_GEN_SCHEMA | warning | Generating schema - creating schema '%s'. | |
ROSE_EC_INVALID_METHOD | error | %s: Invalid method on '%s' object. | |
ROSE_EC_IN_MEMORY | warning | Design %s is already in memory | |
ROSE_EC_MUST_OVERRIDE | error | %s::%s: Function must be overridden. | |
ROSE_EC_MUTATE_ATTDIFF | warning | mutate() '%s' attributes have different types, ignoring. | |
ROSE_EC_MUTATE_NONENT | error | mutate() with non-ENTITY types (%s / %s) | |
ROSE_EC_MUTATE_NP | error | mutate() on non-persistent object | |
ROSE_EC_NESTED_TRAVERSAL | error | Traversal in progress, nested traversals not allowed. | |
ROSE_EC_NO_AGGATT | error | Aggregate class '%s' has no attribute | |
ROSE_EC_NO_ATT | warning | %s: No attribute specified. | |
ROSE_EC_NO_ATTFORADDR | error | getAttribute: Domain '%s' has no attribute at offset %d. | |
ROSE_EC_NO_ATT_DOMAIN | error | Attribute %s.%s has no domain | |
ROSE_EC_NO_CLASS_DOMAIN | error | Class '%s' has no domain | |
ROSE_EC_NO_COPY_CTOR | warning | No copy ctors for 'best-fit' classes. '%s' copied as a '%s'. | |
ROSE_EC_NO_CTOR | error | Class '%s' has no creator function. | |
ROSE_EC_NO_DESIGN | warning | Problems reading design '%s' | |
ROSE_EC_NO_FORMAT | warning | Format '%s' is not recognized. | |
ROSE_EC_NO_HANDLER | warning | No handler for file extension '%s' | |
ROSE_EC_NO_KEYSTONE_OID | warning | No OID for built-in type '%s' | |
ROSE_EC_NO_PROTO | error | Class '%s' has no prototype. | |
ROSE_EC_NP_EXTREF | warning | Unable to resolve external in non-persistent object. | |
ROSE_EC_NP_SLOTADDR | error | Non-persistent object, slotAddress of %s.%s not available | |
ROSE_EC_OBJSUB_TOODEEP | warning | Object forwarding too deep | |
ROSE_EC_OID_BOOT | error | Problems creating ROSE oid index. | |
ROSE_EC_OID_EXISTS | error | Object with OID already exists in design '%s'. | |
ROSE_EC_OID_FAILED | error | Could not generate unique OID. | |
ROSE_EC_PAD_EMPTY_BIN | error | Empty binary with nonzero pad '%c' | |
ROSE_EC_PNEW_TOO_DEEP | fatal | New operator nesting too deep (%d levels) | |
ROSE_EC_READING_ALIASFILE | info | Reading short-name alias file: %s | |
ROSE_EC_ROSE_NOT_SET | info | Using built-in schemas, no runtime schema path. | |
ROSE_EC_RTP_AFTER_BOOT | error | RoseTypePtrs cannot be created after ROSE is initialized. | |
ROSE_EC_RTP_ON_HEAP | fatal | RoseTypePtrs must be statically created. | |
ROSE_EC_SELF_CYCLIC | fatal | Design %s is part of cyclic dependency | |
ROSE_EC_SELF_SCHEMA | fatal | Design %s has itself as it's own schema. | |
ROSE_EC_SET_EXTREF | warning | Unable to put unresolved external reference. | |
ROSE_EC_TRASH_SCHEMA | warning | Schema designs can not be put in the trash | |
ROSE_EC_USEDIN_INTRAV | error | usedin() in begin/end traversal requires backpointers | |
ROSE_EC_USEDIN_NONENT | error | usedin() search for non-ENTITY type (%s) | |
ROSE_EC_USEDIN_NOPATTERN | error | usedin() search pattern object missing | |
ROSE_EC_WRONG_ATT | warning | RoseUnion attribute is %s (requested %s) |
File I/O Errors
These are the issues and messages reported during Part 21 file reading and writing. The RoseError structures for these are kept by the rose_io_ec() context. This context also keeps a different reporting threshold so that file messages can be controlled separately. The preprocessor defines for these codes are prefixed with ROSE_IO and are defined in rose_errors.h.
Error Code | Severity | Message String | Comments |
---|---|---|---|
ROSE_IO_BAD_AGGR_DELIM | error | Unknown delimiter after element %d in attribute %s | |
ROSE_IO_BAD_ALIAS | warning | Unknown type '%s' (short name for '%s'), ignored entity. | |
ROSE_IO_BAD_DELIM | error | Unknown delimiter after attribute '%s' (field %d). | |
ROSE_IO_BAD_DOMAIN | warning | Unknown type '%s', entity ignored. | |
ROSE_IO_BAD_ENTITY | error | Could not read entity definition, ignored. | |
ROSE_IO_BAD_ENTREF | error | Reference to #%lu is %s, not compatible with %s. | |
ROSE_IO_BAD_ENTREF_AT | error | Reference to #%lu on line %d is %s, not compatible with %s. | |
ROSE_IO_BAD_ENUM | error | Unknown enum '%s' for %s in attribute '%s'. | |
ROSE_IO_BAD_HEADER_ENTRY | error | Expecting header entry, found %s instead. | |
ROSE_IO_BAD_IMP_LEVEL | error | Urecognized implementation level '%s' | |
ROSE_IO_BAD_OID_PREFIX | error | Bad OID prefix | |
ROSE_IO_BAD_REFERENCE | error | Reference index can not handle object of type '%s' (nodetype=%d) | |
ROSE_IO_BAD_SCHEMA | warning | Could not find schema '%s'. | |
ROSE_IO_BAD_SELECT_VALTYPE | error | Could not determine type of select value. | |
ROSE_IO_BAD_TYPE_ATTLIST | error | Bad attribute list for %s. | |
ROSE_IO_BAD_TYPE_FOR_AGGR | warning | Type '%s' not allowed in aggregate '%s'. | |
ROSE_IO_BAD_TYPE_FOR_ATT | warning | Type '%s' not allowed for attribute '%s' (type '%s'). | |
ROSE_IO_BAD_TYPE_TOKEN | error | Problems reading %s in attribute '%s'. | |
ROSE_IO_BAD_XTERN_REF | error | Attribute %s of #%lu is an external reference. Writing as NULL. | |
ROSE_IO_CANT_TYPECAST | error | Object of type '%s': Problems casting '%s' value to '%s'. | |
ROSE_IO_COMPLEX_NOT_NEEDED | warning | Entity incorrectly written using an external mapping. | |
ROSE_IO_CONVERT_AGGR | warning | %s: Converting '%s' value to '%s'. | |
ROSE_IO_CONVERT_ATT | warning | Attribute '%s': Converting '%s' value to '%s'. | |
ROSE_IO_DESIGN_LOCKED | error | Design %s is locked, save aborted | |
ROSE_IO_DUPLICATE_EID | warning | Duplicate entity #%lu found, ignored. | |
ROSE_IO_EXPECTING | error | Expecting %s, found %s instead. | |
ROSE_IO_EXPECTING_2 | error | Expecting %s or %s, found %s instead. | |
ROSE_IO_EXPECTING_3 | error | Expecting %s, %s, or %s, found %s instead. | |
ROSE_IO_EXTRA_ENDSCOPE | warning | ENDSCOPE without matching &SCOPE. | |
ROSE_IO_EXTRA_KEYWORD | error | Unexpected keyword '%s' in attribute %s | |
ROSE_IO_EXTRA_OBJ | warning | More than one '%s' object found. | |
ROSE_IO_EXTRA_TYPE_QUALIFIER | warning | Unexpected type qualifier %s() in attribute %s, ignored. | |
ROSE_IO_FIELD_COUNT | error | Expecting %d attribute%s, found %d instead. | |
ROSE_IO_FILE_OPEN_FAILED | error | Unable to open file: %s | |
ROSE_IO_FILE_REMOVE_FAILED | error | Unable to remove file: %s | |
ROSE_IO_FILE_RENAME_FAILED | error | Unable to rename file: %s | |
ROSE_IO_FILE_SAVE_FAILED | error | Unable to save file: %s | |
ROSE_IO_FREAKY_ENUM | error | Found enum of size (%d). Expected: %d %d or %d | |
ROSE_IO_HEADER_ORDER | warning | Header section objects are incorrectly ordered. | |
ROSE_IO_MISSING_OBJ | warning | %s object missing. | |
ROSE_IO_NO_ANDOR | warning | No AND/OR domain for this combination of types, entity ignored. | |
ROSE_IO_NO_ATTRIBUTE | error | Attribute not specified | |
ROSE_IO_NO_ATT_LPAREN | error | Opening paren missing in attribute '%s'. | |
ROSE_IO_NO_ATT_RPAREN | error | Closing paren missing in attribute '%s'. | |
ROSE_IO_NO_ENUM_DOTS | error | '%s' should have enclosing dots in attribute '%s' | |
ROSE_IO_NO_EQUALS | error | Missing equal sign in entity definition. | |
ROSE_IO_NO_HEADER_SCHEMA | warning | Can not find header_section_schema in ST-Runtime | |
ROSE_IO_NO_KEYWORD | error | '%s' keyword missing. | |
ROSE_IO_NO_SCHEMAS | warning | No schemas, make sure compiled EXPRESS is present. | |
ROSE_IO_NO_SECTION_NAME | warning | Expecting a data section name | |
ROSE_IO_NO_SECTION_SCHEMAS | warning | Expecting a list of schemas | |
ROSE_IO_NO_START_PAREN | error | Could not find opening paren, entity ignored. | |
ROSE_IO_NO_TYPENAME | warning | Missing type name in external mapping. | |
ROSE_IO_QUALTYPE_MISMATCH | error | Type %s is inappropriate for select. | |
ROSE_IO_QUALTYPE_NOTFOUND | error | Unknown type %s | |
ROSE_IO_READ_MISMATCH | warning | Reading '(%s) %s.%s': Converting to '(%s) %s.%s' | |
ROSE_IO_TYPE_NOT_DERIVABLE | warning | SELECT type element for '%s' not found. | |
ROSE_IO_UD_ENTITY | warning | User-defined type '%s', entity ignored. | |
ROSE_IO_UNKNOWN_PRIMITIVE | error | Unknown primitive type '(%s) %s.%s' (%d) | |
ROSE_IO_WRITE_MISMATCH | warning | Writing '(%s) %s.%s': Converting to '(%s) %s.%s' |