Overview
The MachineState object holds STEP-NC process state and other information for material removal simulation. You need a unique MachineState object for every file you wish to simulate. Call the constructor with the path to the file you wish to simulate to instantiate an instance of it- ms = new machineState("File.stpnc"); A second option for creating a machine state object is accessed by calling ms = new machineState("File.stpnc", true); This will also create a simulation alongside the Machine State. new machineState("File.stpnc", false) will have the same behavior as new machineState("File.stpnc")
Since MachineState often used with worker threads performing calculations, all functions return a JavaScript Promise object that will resolve to the return value. You can call .then on the promise with a closure that will use the value.
ms.GetGeometryJSON(uuid) .then((out)=>{ console.log("Got JSON: "+out); }).catch((err)=>{ console.log("Promise failed"); };
Constructor
Constructor MachineState(void); MachineState(String^ FileName);
The MachineState class has a default constructor that takes no arguments and one that takes a filename. The filename version is equivalent to creating the object with the default constructor and then calling OpenFile().
Class Init()
static void Init()
The MachineState class has a static Init() function that should be called at the start of an application to do some general initialization.
AdvanceState()
int AdvanceState();
The AdvanceState() function advances the simulation by the smallest possible amount. This will move the tool along all of the toolpaths within a workingstep.
The function returns zero (0) while moving along the workingstep. It returns one (1) when it reaches the end of the workingstep and will continue to return one until you call NextWS() to advance to the next workingstep. The function returns -1 if an error occured.
When simulating material removal, the function returns immediately, but the computation is done in a separate thread. Call WaitForStateUpdate() to block until the removal is complete.
AdvanceStateByT()
int AdvanceStateByT(double t)
The AdvanceStateByT() function behaves as AdvanceState() but it advances the simulation by a user-specified toolpath curve parameter value. See GetCurrentWorkingstepT() for a discussion of parametric curves.
GetCurrentCrossSectionParams()
void GetCurrentCrossSectionParams( [System::Runtime::InteropServices::Out] System::String^ %name, [System::Runtime::InteropServices::Out] double %admax, [System::Runtime::InteropServices::Out] double %rdmax, [System::Runtime::InteropServices::Out] double %xmaxofs, [System::Runtime::InteropServices::Out] double %ymaxofs, [System::Runtime::InteropServices::Out] double %csa, [System::Runtime::InteropServices::Out] double %xcgofs, [System::Runtime::InteropServices::Out] double %ycgofs );
The GetCurrentCrossSectionParams() function returns the aggregate cross section parameters calculated from the most recent material removal simulation, but certain files may also have the parameters stored with the toolpath. These parameters describe a 2D profile in the plane normal to the feed direction.
- admax is the maximum axial depth of the tool contact cross section. The axial depth is measured parallel to the tool axis. For turning, this is measured parallel to the spindle axis.
- rdmax is the maximum radial depth of the tool contact cross section. The radial depth is perpendicular to both tool axis and feed direction.
- xmaxofs is the location along the X axis where the maximum radial depth measure is located. This is the radial offset of the origin (lower left corner) of tool contact cross section measured from the tool tip.
- ymaxofs is the location along the Y axis where the maximum axial depth measure is located. This is the axial offset of the origin (lower left corner) of tool contact cross section measured from the tool tip.
- csa is the total cross section area of the tool engagement.
- xcgofs is the radial offset of the center of gravity of this cross section area. It is measured from the tool tip.
- ycgofs is the axial offset of the center of gravity of this cross section area. It is measured from the tool tip.
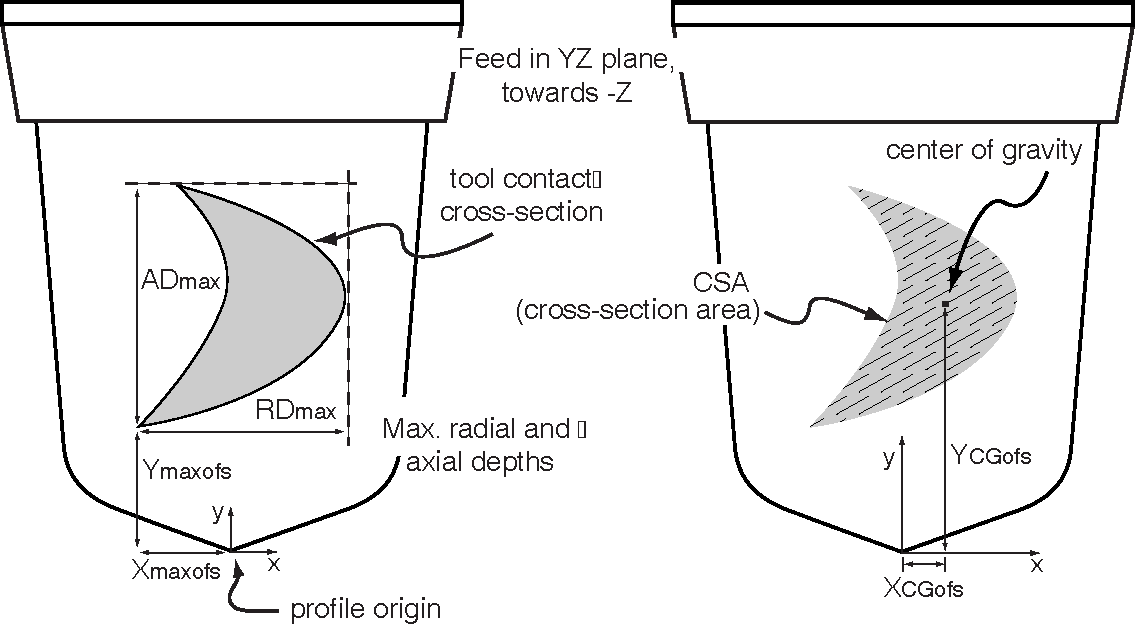
GetCurrentFeedrate()
double GetCurrentFeedrate(); Promise<double> GetCurrentFeedrate();
The GetCurrentFeedrate() function returns the numeric value of the feed rate at the current point in the machining program.
GetCurrentFeedrateUnit()
System::String^ GetCurrentFeedrateUnit();
The GetCurrentFeedrateUnit() function returns the unit for the feed rate at the current point in the machining program.
GetCurrentSpindleSpeed()
double GetCurrentSpindleSpeed(); Promise<double> GetCurrentSpindleSpeed();
The GetCurrentSpindleSpeed() function returns the numeric value of the spindle speed at the current point in the machining program. A zero value means the spindle is stopped. A positive value indicates counter-clockwise rotation and a negative value indicates clockwise rotation. The direction are given looking down the tool axis from the spindle towards the tip of the tool.
GetCurrentSpindleSpeedUnit()
System::String^ GetCurrentSpindleSpeedUnit();
The GetCurrentSpindleSpeed() function returns the unit value of the spindle speed in the machining program.
GetCurrentTechnology()
System::Int64 GetCurrentTechnology();
The GetCurrentTechnology() function returns the technology settings at the current location in the STEP-NC program. The technology instance contains the feedrate and spindle speed settings.
GetCurrentToolpath()
System::Int64 GetCurrentToolpath();
The GetCurrentToolpath() function returns the toolpath instance at the current location in the STEP-NC program.
GetCurrentWorkingstepT()
double GetCurrentWorkingstepT()
The GetCurrentWorkingstepT() function returns the curve parameter value at the current location in the workingstep. Toolpaths are stored as STEP curves which can be described as a function of a single parameter f(t). The parameterization of each different type of curve is given by ISO 10303-42. A workingstep can have many toolpaths and the parameter ranged of all of them are concatenated. The T value returned by this function is the location in all of the toolpaths of the workingstep.
Polylines are the most common curve used for toolpaths and each point on the line increments the T by one. So the first point is at T=0, the second point is at T=1, and so on.
It is important to note that this value is not directly linked to either time or distance, but it can be compared against the maximum T value to estimate progress through a workingstep.
GetCurrentWorkingstepMaxT()
double GetCurrentWorkingstepMaxT()
The GetCurrentWorkingstepMaxT() function returns the maximum curve parameter value for the current workingstep, which is the sum of the max parameters of all toolpaths. See GetCurrentToolpathT() for a discussion of curve parameters.
GetEIDfromUUID()
Promise<int> GetEIDfromUUID( int uuid );
The GetEIDfromUUID() function returns the entity id that is associated with the uuid given.
GetNextWSID
System::Int64 GetNextWSID(); Promise<int> GetNextWSID();
The GetNextWSID function returns the identifier of the workingstep preceeding the current location in the machine process. The workingsteps are linearized, so any nested workplans, disabled steps, and so forth do not appear. Use GetPrevWSID() or or GetWSID() to get the preceeding or active workingsteps in the sequence.
GetPrevWSID
System::Int64 GetPrevWSID(); Promise<int> GetPrevWSID();
The GetPrevWSID function returns the identifier of the workingstep preceeding the current location in the machine process. The workingsteps are linearized, so any nested workplans, disabled steps, and so forth do not appear. Use GetNextWSID() or or GetWSID() to get the following or active workingsteps in the sequence.
GetWSID
System::Int64 GetWSID(); Promise<int> GetWSID();
The GetWSID function returns the identifier of the workingstep at the current location in the machine process. The workingsteps are linearized, so any nested workplans, disabled steps, and so forth do not appear. Use GetNextWSID() or GetPrevWSID() to get adjacent workingsteps in the sequence.
GoToWS()
int GoToWS(System::Int64 wsid); Promise<int> GoToWS(System::Int64 wsid);
The GoToWS() function changes the current position of the machine state to the start of the given workingstep. Returns 0 if the operation succeeds or -1 if an error occured.
LoadMachine()
void LoadMachine(String^ FileName);
The LoadMachine() function initializes the machine state object with a machine tool model. The file must be an XML description file as found in the "machines" subdirectory of STEP-NC Machine installation. This XML file annotates a plain STEP assembly to identify the geometry for each axis. The file is a trivial wrapper if the STEP model is an advanced AP242 file with kinematics.
NextWS()
void NextWS(); Promise<void> NextWS();
The NextWS() function changes the current execution position to the start of the next sequential workingstep.
OpenFile()
void OpenFile(String^ FileName, Boolean enable_sim);
The OpenFile() function initializes the machine state object to work with the machining project in the given STEP-NC file. The second parameter is an optional boolean value that indicates whether to do material removal simulation.
PrevWS()
void PrevWS(); Promise<void> PrevWS();
The PrevWS() function changes the current execution position to the start of the previous sequential workingstep.
GetKeyStateJSON() / GetDeltaStateJSON()
System::String^ GetKeyStateJSON(); System::String^ GetDeltaStateJSON(); Promise<string> GetKeyStateJSON(); Promise<string> GetDeltaStateJSON();
The GetKeyStateJSON() function return a JSON object which describes the current placement of geometry objects in the scene.
The GetDeltaStateJSON() function returns a JSON object with only the items that have moved since the last call to either of these two functions. The C++ version takes a bool, when true it returns a Key State, when false it returns a Delta State.
Update()
Promise<void> Update();
The Update() function rebuilds the machine state cache. This should be called if the process is changed by adding, removing, enabling or disabling workingsteps.
WaitForStateUpdate()
int WaitForStateUpdate();
The WaitForStateUpdate() function blocks until AdvanceState() finishes calculations in a separate thread, and it will return the same return code as the original call to AdvanceState().
If material removal is not being calculated, the function returns immediately. The return value should not be used for anything if not doing material removal.
This function is not used by the node interface. All asynchronous processing is coordinated by resolving returned Promise objects.